Apple’s interviews are not only about technical skills but also about problem-solving abilities. The questions often reflect real-world business scenarios, testing candidates’ algorithm proficiency, logical reasoning, and clear communication. This detailed account of an Apple interview demonstrates how a candidate, with precise guidance from CSOAHelp, confidently tackled the challenges and won the interviewer’s approval.
The interview began with the interviewer introducing the role and presenting the following problem:
Problem 1: Best Time to Buy and Sell Stock with Transaction Fee
Description:
You are given:
- An array
prices
whereprices[i]
is the price of a given stock on thei
th day. - An integer
fee
representing a transaction fee.
Find the maximum profit you can achieve. You may complete as many transactions as you like, but you need to pay the transaction fee for each transaction.
Constraints:
- You may not engage in multiple transactions simultaneously (i.e., you must sell the stock before you buy again).
- The transaction fee is charged once for each stock purchase and sale.
Input:
prices = [1, 3, 2, 8, 4, 9]
fee = 2
Output:
8
Explanation:
The total profit is calculated as:
- Buy at
1
, sell at8
→ profit =(8 - 1) - 2 = 5
- Buy at
4
, sell at9
→ profit =(9 - 4) - 2 = 3
Total profit = 5 + 3 = 8
.
After listening to the problem, CSOAHelp provided a clear solution and guided the candidate to explain it effectively to the interviewer.
The candidate, following CSOAHelp's suggestions, explained: The problem can be solved using dynamic programming with two state variables:
hold
: Maximum profit when holding a stock.cash
: Maximum profit when not holding a stock.
The two states are updated daily as follows:
- For buying:
hold = max(hold, cash - prices[i])
, subtracting the stock price from the current cash to update the hold state. - For selling:
cash = max(cash, hold + prices[i] - fee)
, adding the stock price minus the transaction fee to update the cash state.
The interviewer praised the candidate's explanation, describing it as logical and easy to understand.
With CSOAHelp’s guidance, the candidate implemented the following code:
def max_profit(prices, fee):
hold, cash = float('-inf'), 0 # Initialize variables
for price in prices:
hold = max(hold, cash - price) # Update profit when holding
cash = max(cash, hold + price - fee) # Update profit when not holding
return cash # Return the maximum profit
Code Explanation:
hold
is initialized to negative infinity, as it is impossible to hold a stock initially.- The prices array is traversed, updating
hold
andcash
based on the state transition formulas. - The final profit is returned in the
cash
state.
Follow-Up Questions
Interviewer: Why can’t a greedy algorithm solve this problem?
CSOAHelp suggested: Due to transaction fees, a greedy approach might result in excessive transactions, reducing overall profit. Dynamic programming ensures each step considers both cost and profit for optimal results.
The candidate, guided by CSOAHelp, responded: A greedy algorithm overlooks the impact of transaction fees and may lead to unnecessary trades. Dynamic programming carefully balances costs and profits to guarantee a global optimum.
Interviewer: What is the time complexity of this solution?
CSOAHelp suggested: The time complexity is O(n), as the prices array is traversed once. The space complexity is O(1), using only two variables.
The candidate repeated: The time complexity is O(n), as the algorithm iterates through the array once. The space complexity is O(1), making it efficient for large datasets.
Interviewer: How would you handle dynamic transaction fees or massive stock data?
CSOAHelp suggested: Use event-driven mechanisms to adjust transaction fees dynamically. For large datasets, leverage distributed computing frameworks like Hadoop or Spark.
The candidate, based on this guidance, responded: Event-driven mechanisms can monitor and adapt to changes in transaction fees. Distributed frameworks can partition and process data efficiently, improving performance for large-scale problems.
Additional Questions
Interviewer: How does Apple ensure data privacy in distributed systems?
CSOAHelp suggested: Implement end-to-end encryption for data transmission. Use partitioned storage to distribute sensitive data across multiple nodes. Employ zero-knowledge proofs to enable computations without exposing raw data.
The candidate responded: End-to-end encryption ensures secure data transmission. Partitioned storage minimizes risks by spreading data across nodes. Zero-knowledge proofs allow collaborative computations while keeping data confidential.
Interviewer: How do you promote collaboration in a diverse team?
CSOAHelp suggested: Organize regular technical sharing sessions to foster mutual understanding. Clearly define roles and responsibilities to streamline workflows.
The candidate repeated: Regular technical sharing sessions help team members learn from each other. Clear roles and responsibilities ensure smooth project execution, especially in agile environments.
Interviewer: How does Apple’s focus on innovation influence technical roles?
CSOAHelp suggested: Foster a culture of openness to encourage creativity. Allow teams to experiment and learn from failures, optimizing solutions iteratively.
The candidate, guided by the prompt, answered: Encouraging open dialogue fosters creativity. Teams should have the freedom to try new methods, even if they fail initially, as learning from mistakes drives continuous improvement.
Conclusion
Throughout the interview, CSOAHelp provided precise, real-time guidance, enabling the candidate to approach each question methodically. By accurately following prompts and presenting clear, logical explanations, the candidate showcased strong technical skills and problem-solving abilities, ultimately earning the interviewer’s approval.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
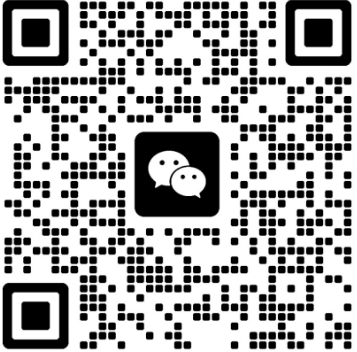