At PayPal, a global leader in payment technology, technical interviews go beyond assessing coding skills—they test problem-solving, scalability, and your ability to stay calm under pressure. In the Virtual Onsite (VO) interview, candidates are expected to demonstrate their capacity to handle complex problems while maintaining logical clarity and precision, even when faced with challenging follow-up questions.
The "Shopping Cart Billing" problem is a prime example of PayPal’s business-oriented interview design. It tests not only a candidate's programming proficiency but also their ability to optimize algorithms, think modularly, and extend solutions for real-world e-commerce scenarios. In this case, the candidate, with the discreet yet powerful support of CSOAHELP, was able to navigate through all obstacles, leaving a strong impression on the interviewer.
The Problem: Shopping Cart Billing
The problem statement reads as follows:
An e-commerce company is currently celebrating ten years in business. They are having a sale to honor their privileged members, those who have been using their services for the past five years. They receive the best discounts indicated by any discount tags attached to the product. Determine the minimum cost to purchase all products listed. As each potential price is calculated, truncate it to its integer part before adding it to the total. Return the cost to purchase all items as an integer.
There are three types of discount tags:
Type 0: discounted price, the item is sold for a given price.
Type 1: percentage discount, the customer is given a fixed percentage discount from the retail price.
Type 2: fixed discount, the customer is given a fixed amount off from the retail price.
Example Input and Output
Input:
Products: [['10', 'd0', 'd1'], ['15', 'EMPTY'], ['20', 'd1', 'EMPTY']]
Discounts: [['d0', '1', '27'], ['d1', '2', '5']]
Output:
35
Explanation:
- First product (price = 10):
d0
(Type 1, percentage discount):10 - 10 * 0.27 = 7.3
→ truncated to7
.d1
(Type 2, fixed discount):10 - 5 = 5
.- Minimum price:
5
.
- Second product (price = 15):
- No discounts available, final price:
15
.
- No discounts available, final price:
- Third product (price = 20):
d1
(Type 2, fixed discount):20 - 5 = 15
.- Minimum price:
15
.
The total cost is 5 + 15 + 15 = 35
.
Candidate’s Implementation
The candidate initially provided the following code:
def findLowestPrice(products, discounts):
# Create a mapping for discounts
discount_map = {}
for discount in discounts:
tag, type_, amount = discount
discount_map[tag] = (int(type_), float(amount))
total_cost = 0
for product in products:
price = int(product[0]) # Base price
min_price = price # Start with the base price as the minimum
for tag in product[1:]:
if tag == "EMPTY":
continue
if tag in discount_map:
type_, amount = discount_map[tag]
if type_ == 0: # Fixed price
min_price = min(min_price, int(amount))
elif type_ == 1: # Percentage discount
discounted_price = price - (price * (amount / 100))
min_price = min(min_price, int(discounted_price))
elif type_ == 2: # Fixed discount
discounted_price = price - amount
min_price = min(min_price, int(discounted_price))
total_cost += min_price
return total_cost
The candidate’s approach correctly computes the minimum cost for each product by evaluating all discount tags. While functional, this code opened the door to deeper questions about efficiency, scalability, and extensibility.
The Interview: Layers of Challenges and Follow-Up Questions
Once the implementation was complete, the interviewer began a series of probing questions to evaluate the candidate’s ability to handle larger data sets, introduce new requirements, and analyze worst-case scenarios. Each question was designed to challenge the candidate's understanding and adaptability.
Question 1: Optimizing the Algorithm
The interviewer’s question:
“How would you optimize this solution if the number of products increased to 10,000, with each product having up to 100 discount tags? Can you make the algorithm more efficient?”
At this moment, CSOAHELP stepped in to provide precise and actionable guidance.
CSOAHELP’s Complete Assistance:
- Highlight the current complexity:
“Mention the current complexity as O(n × m), where n is the number of products and m is the average number of discount tags per product.” - Propose specific optimizations:
- “Use a hash map (dictionary) to store discount tags for O(1) lookups.”
- “Introduce caching for repeated discount calculations.”
- “Suggest batch processing or parallel computation for large-scale inputs.”
- Explain the expected outcome:
“After optimization, the complexity could approach O(n) if redundant computations are minimized.”
Candidate’s Answer:
“The current algorithm has a complexity of O(n × m), where n is the number of products and m is the average number of discount tags. To optimize:
- I would use a hash map to store discount tags, reducing lookup time to O(1).
- Cache discount computations for frequently used tags to avoid recalculating.
- For extremely large data sets, I’d employ batch processing or distributed systems to parallelize the workload. These optimizations would significantly reduce processing time, especially for high-volume datasets.”
The interviewer nodded, impressed by the clarity and depth of the response.
Question 2: Adding a New Discount Type
The interviewer’s question:
“If we were to introduce a new discount type, say ‘buy-one-get-one-free,’ how would your implementation adapt to this change?”
This question tested the modularity and scalability of the candidate’s design.
CSOAHELP’s Complete Assistance:
- Highlight modular design:
“Mention that the discount logic is already abstracted into separate functions, allowing easy extension.” - Provide a concrete example:
- “Define a new function for the ‘buy-one-get-one-free’ logic, e.g.:”
def handle_buy_one_get_one(price, count): return (count // 2 + count % 2) * price
- “Integrate the new function into a discount handler mapping table.”
- “Define a new function for the ‘buy-one-get-one-free’ logic, e.g.:”
- Conclude with benefits:
“Emphasize that this approach adheres to the open/closed principle, making the system extensible without altering existing logic.”
Candidate’s Answer:
“I’d abstract each discount type into its own function. For example, a new type like ‘buy-one-get-one-free’ could be handled as follows:
def handle_buy_one_get_one(price, count): return (count // 2 + count % 2) * price
This function would then be added to a mapping table of discount handlers, ensuring that the main logic remains untouched. This approach makes the code easy to extend while maintaining clarity and modularity.”
The candidate’s ability to illustrate a practical implementation left the interviewer visibly impressed.
Question 3: Worst-Case Complexity Analysis
The interviewer’s question:
“If every product has the maximum number of discount tags, how does your algorithm perform? What bottlenecks might you face?”
This question pushed the candidate to assess both theoretical complexity and practical implications under extreme conditions.
CSOAHELP’s Complete Assistance:
- Break down the complexity:
“Explain that the worst-case complexity is O(n × d), where d is the maximum number of discount tags.” - Identify bottlenecks:
- “Highlight computational bottlenecks from repeated discount evaluations.”
- “Point out memory usage issues with large product-discount mappings.”
- Propose solutions:
- “Use sparse matrix representations to handle sparse product-discount relationships.”
- “Introduce distributed processing frameworks like Hadoop or Spark for large-scale tasks.”
Candidate’s Answer:
“In the worst case, the algorithm’s complexity is O(n × d), where n is the number of products and d is the maximum number of discount tags. Potential bottlenecks include:
- Repeated evaluations for overlapping discount tags, which can slow down computation.
- Memory usage spikes due to large-scale mappings of products and discounts. To address this, I’d use a sparse matrix to represent product-discount relationships and minimize redundant calculations. Additionally, for extremely large datasets, distributed processing frameworks like Hadoop or Spark could be employed to scale efficiently.”
The interviewer seemed satisfied, acknowledging the candidate’s ability to address both theoretical and practical concerns.
The Role of CSOAHELP: Invisible but Indispensable
Throughout the interview, CSOAHELP’s timely and detailed guidance was the silent force behind the candidate’s success. From offering structured answers to providing implementation-ready examples, CSOAHELP ensured the candidate’s responses were not only accurate but also professionally articulated.
Conclusion
The "Shopping Cart Billing" interview problem pushed the candidate to demonstrate technical skill, scalability, and clarity under pressure. Thanks to CSOAHELP’s behind-the-scenes assistance, the candidate delivered precise, thoughtful answers to every question, earning the respect of the interviewer.
For candidates aiming to excel in high-stakes interviews, CSOAHELP isn’t just a tool—it’s the ultimate partner, ensuring they perform at their best when it matters most.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
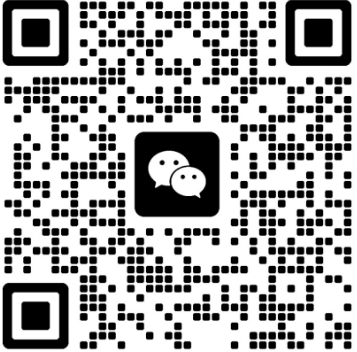