Question
The current selected programming language is Java 21. We emphasize the submission of a fully working code over partially correct but efficient code. Once submitted, you cannot review this problem again. You can use system.out.println to debug your code. The system.out.println() may not work in case of syntax/runtime error. The version of Java 21 being used is 21.0.4.
An alternate sort of a list consists of alternate elements (starting from the first position) of the given list after sorting it in an ascending order. You are given a list of unsorted elements. Write an algorithm to find the alternate sort of the given list.
Input
- The first line of the input consists of an integer size, representing the size of the given list (N).
- The second line consists of N integers arr1, arr2, ..., arrN, representing the elements of input list.
Output
Print space-separated integers representing the alternately sorted elements of the given list.
Constraints
0 < size ≤ 10^6
-10^6 ≤ arr[i] ≤ 10^6
0 ≤ i < size
Example
Input:
8
3 5 1 5 9 10 2 6
Output:
1 3 5 9
Explanation:
After sorting, the list is [1, 2, 3, 5, 5, 6, 9, 10].
So, the alternate elements of the sorted list are [1, 3, 5, 9].
Question
The current selected programming language is Java 21. We emphasize the submission of a fully working code over partially correct but efficient code. Once submitted, you cannot review this problem again. You can use system.out.println to debug your code. The system.out.println() may not work in case of syntax/runtime error. The version of Java 21 being used is 21.0.4.
You are given a non-empty list of positive integers. You can sum any two consecutive elements to form a single element. The result thus obtained can be reused further and this process can be repeated any number of times to convert the given list into a palindromic list of maximum length.
Write an algorithm to convert this list into a palindromic list of maximum length.
Input
- The first line of the input consists of an integer num, representing the number of elements in the list (N).
- The second line consists of N space-separated positive integers, arr[0], arr[1], ..., arr[N-1] representing the elements of the list.
Output
Print the space-separated positive integers representing a palindromic list.
Example
Input:
6
15 10 15 34 25 15
Output:
15 25 34 25 15
Explanation:
The list - [15 10 15 34 25 15] can be converted into a palindromic list [15 25 34 25 15] by combining 10 and 15 at index 2 and index 3, respectively, to create 25 at index 2.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
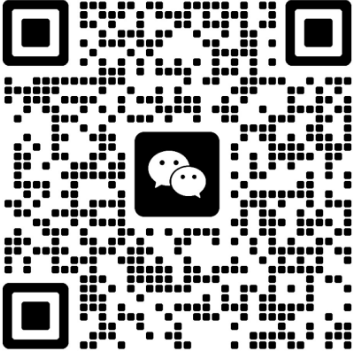