The cloud storage system should support operations to add files, copy files, and get files stored on the system.
- add_file(self, name: str, size: int) -> bool
Should add a new filename
to the storage.size
is the amount of memory required in bytes. The current operation fails if a file with the samename
already exists. ReturnsTrue
if the file was added successfully orFalse
otherwise. - copy_file(self, name_from: str, name_to: str) -> bool
Should copy the file atname_from
toname_to
. The operation fails ifname_from
points to a file that does not exist or points to a directory. The operation fails if the specified file already exists atname_to
. ReturnsTrue
if the file was copied successfully orFalse
otherwise. - get_file_size(self, name: str) -> int | None
Should return the size of the filename
if it exists, orNone
otherwise.
Examples
The example below shows how these operations should work:
Queries
add_file("/dir1/dir2/file.txt", 10)
- Returns
True
; adds file/dir1/dir2/file.txt
of size 10.
- Returns
copy_file("/not-existing.file", "/dir1/file.txt")
- Returns
False
; the file/not-existing.file
does not exist.
- Returns
copy_file("/dir1/dir2/file.txt", "/dir1/file.txt")
- Returns
True
; adds file/dir1/file.txt
with size 10.
- Returns
add_file("/dir1/file.txt", 15)
- Returns
False
; the file/dir1/file.txt
already exists.
- Returns
copy_file("/dir1/file.txt", "/dir1/dir2/file.txt")
- Returns
False
; the file/dir1/dir2/file.txt
already exists.
- Returns
get_file_size("/dir1/file.txt")
- Returns
10
.
- Returns
get_file_size("/not-existing.file")
- Returns
None
; the file does not exist.
- Returns
Implement support for retrieving file names by searching directories via prefixes and suffixes.
- find_file(self, prefix: str, suffix: str) -> list[str]
Should search for files with names starting withprefix
and ending withsuffix
. Returns a list of strings representing all matching files in this format:["<name_1>(<size_1>)", "<name_2>(<size_2>)", ...]
.
The output should be sorted in descending order of file sizes or, in the case of ties, lexicographically. If no files match the required properties, should return an empty list.
Examples
The example below shows how these operations should work:
Queries
add_file("/root/dir/another_dir/file.mp3", 10)
- Returns
True
.
- Returns
add_file("/root/file.mp3", 5)
- Returns
True
.
- Returns
add_file("/root/music/file.mp3", 7)
- Returns
True
.
- Returns
copy_file("/root/music/file.mp3", "/root/dir/file.mp3")
- Returns
True
.
- Returns
find_file("/root", ".mp3")
- Returns
["/root/dir/another_dir/file.mp3(10)", "/root/music/file.mp3(7)", "/root/file.mp3(5)"]
.
- Returns
find_file("/root", "file.txt")
- Returns
[]
; there is no match.
- Returns
find_file("/dir", "file.mp3")
- Returns
[]
; there is no match.
- Returns
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
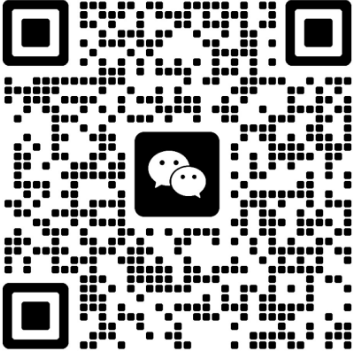