Problem Statement: Find the Maximum Length of a Good Subsequence
You are given an integer array nums
and a non-negative integer k
. A sequence of integers seq
is called good if there are at most k indices i
in the range [0, seq.length - 2]
such that seq[i] != seq[i + 1]
.
Return the maximum possible length of a good subsequence of nums
.
Example 1:
Input:
nums = [1, 2, 1, 1, 3], k = 2
Output:
4
Explanation:
The maximum length subsequence is [1, 2, 1, 1, 3]
.
Example 2:
Input:
nums = [1, 2, 3, 4, 5, 1], k = 0
Output:
2
Explanation:
The maximum length subsequence is [1, 2, 3, 4, 5, 1]
.
Constraints:
1 ≤ nums.length ≤ 500
1 ≤ nums[i] ≤ 10^9
0 ≤ k ≤ min(nums.length, 25)
Problem Statement: Remove Stones to Minimize the Total
You are given a 0-indexed integer array piles
, where piles[i]
represents the number of stones in the i
-th pile, and an integer k
. You should apply the following operation exactly k times:
- Choose any
piles[i]
and remove floor(piles[i] / 2) stones from it.
Notice:
- You can apply the operation on the same pile more than once.
Goal:
Return the minimum possible total number of stones remaining after applying the k
operations.
floor(x)
is the greatest integer that is smaller than or equal to x
(i.e., rounds x
down).
Examples
Example 1:
Input:
piles = [5,4,9], k = 2
Output:
12
Explanation:
Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are
[5, 4, 5]
. - Apply the operation on pile 0. The resulting piles are
[3, 4, 5]
.
The total number of stones in [3, 4, 5]
is 12.
Example 2:
Input:
piles = [4,3,6,7], k = 3
Output:
12
Explanation:
Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are
[4, 3, 3, 7]
. - Apply the operation on pile 3. The resulting piles are
[4, 3, 3, 4]
. - Apply the operation on pile 0. The resulting piles are
[2, 3, 3, 4]
.
The total number of stones in [2, 3, 3, 4]
is 12.
Constraints:
1 ≤ piles.length ≤ 10^5
1 ≤ piles[i] ≤ 10^4
1 ≤ k ≤ 10^5
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
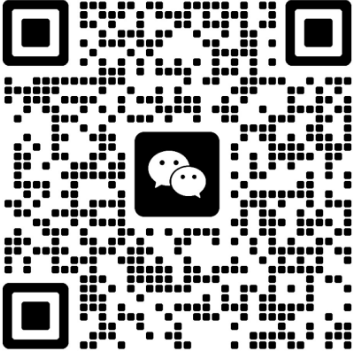