Your best friend is a mutant who can run at extreme speeds and perform complex movements. However, due to a light form of amnesia, he cannot remember the first instruction he was given.
Your friend is moving on a grid, following a sequence of 4 possible instructions:
- FORWARD: Moves 1 step in the direction he’s facing.
- BACK: Moves 1 step away from the direction he’s facing, without changing orientation.
- TURN LEFT: Rotates a quarter turn to the left without changing coordinates.
- TURN RIGHT: Rotates a quarter turn to the right without changing coordinates.
Your friend has memorized an incorrect sequence of instructions, which does not lead him to the target coordinates. You must fix this sequence by changing only the first instruction.
Objective:
Write a function fix_first_instruction(instructions, target)
that returns a string in the format:
"Replace instruction 1 with [command]"
Where [command]
is the correct instruction to replace the first one.
Rules
- Your friend always starts at coordinates
(0, 0)
facing the right. - Moving to the right increases the X coordinate; moving up increases the Y coordinate.
- Instructions provided to your code should result in exactly the target coordinates after executing all movement instructions.
- Only the first instruction can be replaced, and there is always a valid replacement instruction.
Example
Input:
instructions = [
"FORWARD",
"TURN RIGHT",
"FORWARD",
"TURN RIGHT",
"FORWARD",
"FORWARD",
"FORWARD",
"FORWARD"
]
target = (-1, 4)
Current Coordinates:
Executing the instructions leads to (3, -1)
, which is not the target.
Output:
"Replace instruction 1 with TURN RIGHT"
Explanation:
Replacing the first instruction with TURN RIGHT
changes the path to lead the friend to (-1, 4)
. The corrected sequence of instructions is:
TURN RIGHT, TURN RIGHT, FORWARD, TURN RIGHT, FORWARD, FORWARD, FORWARD, FORWARD
Function Signature:
def fix_first_instruction(instructions: list, target: tuple) -> str:
pass
Let me know if you'd like me to implement the function!
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
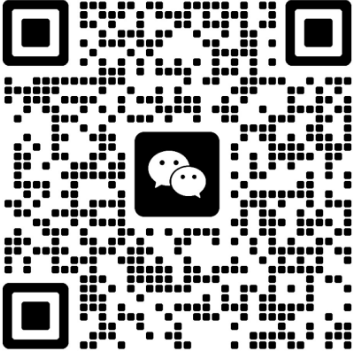