Problem Statement
You are given an array of integers arr
. Consider its elements in pairs:(arr[0], arr[1])
, (arr[2], arr[3])
, and so on.
Arrange the elements in each pair in ascending order. In other words, swap the position of paired elements if the left element is greater than the right one.
If arr
contains an odd number of elements, the last element should be left unchanged.
Note: You are not expected to provide the most optimal solution, but a solution with time complexity not worse than O(arr.length) will fit within the execution time limit.
Example
Example 1:
For arr = [1, 5, 7, 3, 2, 1]
, the output should be:solution(arr) = [1, 5, 3, 7, 1, 2]
Explanation:
- The given array consists of 3 pairs:
(1, 5)
,(7, 3)
,(2, 1)
. - After arranging elements in each pair in ascending order, we get:
(1, 5)
,(3, 7)
,(1, 2)
. - So, the answer is
[1, 5, 3, 7, 1, 2]
.
Example 2:
For arr = [6, 7, 8, 8, 3, 5, 2]
, the output should be:solution(arr) = [6, 7, 8, 8, 3, 5, 2]
Explanation:
- The given array consists of 3 pairs and 1 ending element:
(6, 7)
,(8, 8)
,(3, 5)
, and(2)
. - After arranging elements in every pair in ascending order, we get:
(6, 7)
,(8, 8)
,(3, 5)
, and(2)
. - So, the answer is
[6, 7, 8, 8, 3, 5, 2]
.
Input/Output
Execution Time Limit:
4 seconds (py3)
Memory Limit:
1 GB
Input:array.integer arr
- An array of integers.
Guaranteed Constraints:
- 1 <= arr.length <= 1000
- -10^9 <= arr[i] <= 10^9
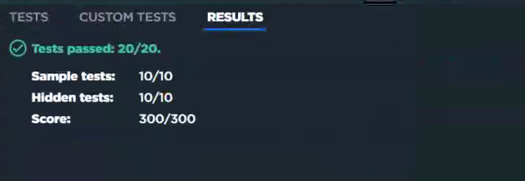
Problem Statement
Given a string message
and an integer n
, replace every nth consonant with the next consonant from the alphabet while keeping the case consistent (e.g., 'b'
becomes 'c'
, 'x'
becomes 'y'
, etc.).
Notes:
- The list of consonants in alphabetical order is:
'b', 'c', 'd', 'f', 'g', 'h', 'j', 'k', 'l', 'm', 'n', 'p', 'q', 'r', 's', 't', 'v', 'w', 'x', 'y', 'z'
.'z'
must be replaced with'b'
(and'Z'
with'B'
). - You are not expected to provide the most optimal solution, but a solution with time complexity not worse than O(message.length) will fit within the execution time limit.
Example
Example 1:
For message = "CodeSignal"
and n = 3
, the output should be:solution(message, n) = "CodeTignam"
Explanation:
- The given string
"CodeSignal"
contains the following consonants:'C', 'd', 'S', 'g', 'n', 'l'
.'C'
is the first consonant, so it is unchanged.'d'
is the second consonant, so it is unchanged.'S'
is the third consonant, so it is replaced with'T'
.'g'
is the first consonant, so it is unchanged.'n'
is the second consonant, so it is unchanged.'l'
is the third consonant, so it is replaced with'm'
.
- The resulting string becomes
"CodeTignam"
.
Example 2:
For message = "Quiz, Citizenship, puzZle"
and n = 5
, the output should be:solution(message, n) = "Quiz, Citizenship, quZzle"
Explanation:
- The string
"Quiz, Citizenship, puzZle"
contains the following consonants:'Q', 'z', 'C', 't', 'z', 'n', 's', 'h', 'p', 'p', 'Z', 'z', 'l'
.'Q'
is the first consonant, so it is unchanged.'z'
is the second consonant, so it is unchanged.'C'
is the third consonant, so it is unchanged.'t'
is the fourth consonant, so it is unchanged.'z'
is the fifth consonant, so it is replaced with'b'
.'n'
is the first consonant, so it is unchanged.'s'
is the second consonant, so it is unchanged.'h'
is the third consonant, so it is unchanged.'p'
is the fourth consonant, so it is unchanged.'p'
is the fifth consonant, so it is replaced with'q'
.'Z'
is the first consonant, so it is unchanged.'z'
is the second consonant, so it is unchanged.'l'
is the third consonant, so it is unchanged.
- The resulting string becomes
"Quiz, Citizenship, quZzle"
.
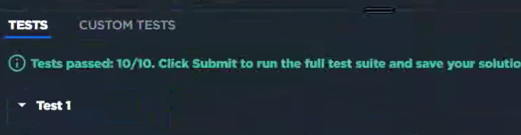
Problem Statement
Civil engineers are simulating rainfall on a digital elevation model to study water flow. You are given a 2D array of integers heights
representing terrain heights and two integers startRow
and startCol
indicating the water's starting point.
Simulate the water flow from the starting point with these rules:
- Water flows to adjacent cells (up, down, left, right) if the adjacent cell's height is less than or equal to the current cell's height.
- The flow stops when no adjacent cell has a lower or equal height.
Return a 2D array of integers where each cell contains the time step when it becomes wet, starting at 0 for the initial cell. If a cell remains dry, its value should be -1. The dimensions of the output array should match those of the input heights
.
Note:
You are not expected to provide the most optimal solution, but a solution with time complexity not worse than O(heights.length × heights[0].length) will fit within the execution time limit.
Example
Example 1:
For
heights = [
[3, 2, 1],
[6, 5, 4],
[9, 8, 7]
]
startRow = 1, startCol = 1
The output should be:
solution(heights, startRow, startCol) = [
[-1, 1, 2],
[-1, 0, 1],
[-1, -1, -1]
]
Explanation:
- Water starts at cell
(1, 1)
and flows to adjacent cells with lower height. The simulation stops when no more cells can be flowed into from the current cells. - The lower number in the cell represents the time step when the cell becomes wet.
Example 2:
For
heights = [
[42]
]
startRow = 0, startCol = 0
The output should be:
solution(heights, startRow, startCol) = [
[0]
]
Explanation:
- The terrain consists of a single cell. The starting point is this cell, so it becomes wet at time 0.
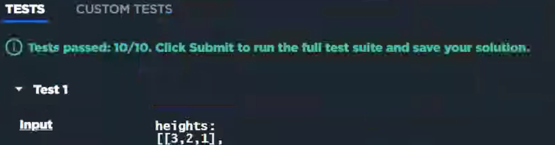
Problem Statement
You are given two arrays of integers a
and b
, and an array queries
, the elements of which are queries you are required to process. Every queries[i]
can have one of the following two forms:
[0, i, x]
: In this case, you need to assigna[i]
the value ofx
(i.e.,a[i] = x
).[1, x]
: In this case, you need to find the total number of pairs of indicesi
andj
such thata[i] + b[j] = x
.
Perform the given queries in order and return an array containing the results of the queries of the type [1, x]
.
Example
Example 1:
For
a = [3, 4], b = [1, 2, 3], queries = [[1, 5], [0, 0, 1], [1, 5]]
The output should be:
solution(a, b, queries) = [2, 1]
Explanation:
The arrays look like this initially:
a = [3, 4]
b = [1, 2, 3]
- For the query
[1, 5]
, there are two ways to form a sum of 5 using an element from each array:5 = 3 + 2 = a[0] + b[1]
5 = 4 + 1 = a[1] + b[0]
So, the result is2
.
- The query
[0, 0, 1]
re-assigns the value ofa[0]
to1
, so the arrays now look like this:a = [1, 4] b = [1, 2, 3]
- For the query
[1, 5]
, there is now only one way to form a sum of 5 using an element from each array:5 = 4 + 1 = a[1] + b[0]
So, the result is1
.
Since the two queries of type [1, x]
gave results of 2
and 1
respectively, the answer is [2, 1]
.
Example 2:
For
a = [2, 3], b = [1, 2, 2], queries = [[1, 4], [0, 0, 3], [1, 5]]
The output should be:
solution(a, b, queries) = [3, 4]
Explanation:
The arrays look like this initially:
a = [2, 3]
b = [1, 2, 2]
- For the query
[1, 4]
, there are three ways to form a sum of 4 using an element from each array:4 = 2 + 2 = a[0] + b[1]
4 = 2 + 2 = a[0] + b[2]
4 = 3 + 1 = a[1] + b[0]
So, the result is3
.
- The query
[0, 0, 3]
re-assigns the value ofa[0]
to3
, so the arrays now look like this:a = [3, 3] b = [1, 2, 2]
- For the query
[1, 5]
, there are now four ways to form a sum of 5 using an element from each array:5 = 3 + 2 = a[0] + b[1]
5 = 3 + 2 = a[0] + b[2]
5 = 3 + 2 = a[1] + b[1]
5 = 3 + 2 = a[1] + b[2]
So, the result is4
.
Since the two queries of type [1, x]
gave results of 3
and 4
respectively, the answer is [3, 4]
.
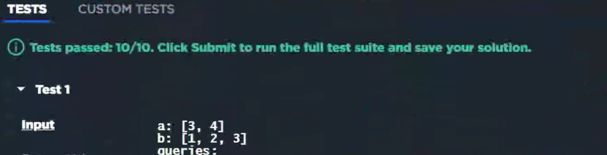
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
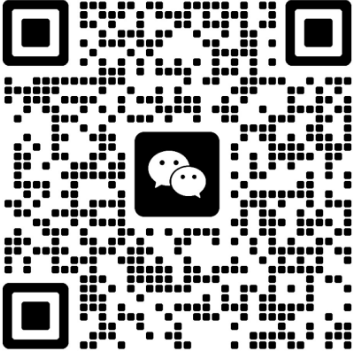