Title: Movie Recommendation System
Objective: Create a movie recommendation system based on user choices and ratings.
Description: You are tasked with building a basic recommendation system for movies. The system should take a list of user ratings for various movies by genre and recommend movies to a specific user based on genre and the ratings of other users.
Input:
- A list of users with their ratings for various movies in different genres. Each rating is between 1 and 5.
- A specific user for whom the recommendations need to be generated.
- Genre of their choice.
Output:
- A list of recommended movies for the specified user and genre, sorted by the highest predicted rating.
Constraints:
- Handle edge cases where the user has rated all or no movies.
- Handle edge cases where a movie is not rated at all – it should not be part of the recommended list.
- Ensure your solution is efficient and can handle large datasets.
Instructions:
- Write a program in a language of your choice (Java preferred, Python, etc.) to implement the recommendation system.
- Document your code and explain your approach in a README file.
- Include tests to verify the correctness of your implementation.
Inputs to use:
- Create the classes needed for below and initialize the input. Hackerrank might force you to use the main class with the Solution.java (code stub), and you may have to resort to static methods in the Result class.
Movies & Genres:
- "Inception": "Sci-Fi"
- "Avatar": "Sci-Fi"
- "Titanic": "Romance"
- "The Notebook": "Romance"
Users:
- Alice
- Bob
- Charlie
- Dave
Ratings:
- "Alice", "Inception", 5
- "Alice", "Avatar", 3
- "Bob", "Inception", 4
Title: Stock Market Prices Moving Average
Task Description: You are tasked with developing a simple stock market analyzer that processes a stream of stock prices and calculates the moving average. The system should be able to:
- Ingest real-time stock price updates. This method is needed before the method in step 2 is implemented.
- Calculate the moving average of stock prices over a given window size. The latest prices should fall within the window; for example, if the window size is 2 but there are 5 prices, the average should be on the latest 2, not the first 2 that were inserted.
- Ensure thread safety for concurrent updates and queries.
Requirements:
- Ingestion of Stock Prices:
- Implement a method
ingest(String stockSymbol, double price)
to ingest real-time stock prices for a given stock symbol to support the below method. - Ensure that the ingestion can handle concurrent updates.
- Implement a method
- Moving Average Calculation:
- Implement a method
getMovingAverage(String stockSymbol, int windowSize)
that returns the moving average of the stock prices for the given stock symbol over the specified window size. - If there are fewer prices than the window size, return the average of all available prices.
- Test cases will evaluate this method.
- Implement a method
- Concurrency:
- Ensure that the methods can handle concurrent access and updates.
Constraints:
- Stock symbols are case-sensitive and consist of uppercase letters.
- Prices are positive real numbers.
- The window size for moving average and maximum price calculation will always be a positive integer.
Inputs for Testing:
ingest("AAPL", 150.0)
ingest("AAPL", 155.0)
ingest("AAPL", 160.0)
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
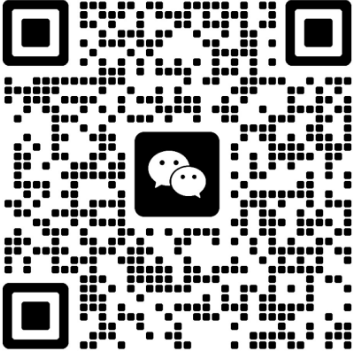