Problem: Reach the End in Time
You are given a 2-D grid consisting of some blocked cells (represented as '#'
) and some unblocked cells (represented as '.'
). The pointer starts at the top-left corner of the grid. It is guaranteed that the starting position is in an unblocked cell, and the bottom-right corner is also unblocked.
Each cell in the grid is connected to its adjacent cells on the right, left, top, and bottom (if those cells exist). It takes 1 second for the pointer to move from one cell to an adjacent cell. Your task is to determine whether the pointer can reach the bottom-right corner of the grid within the given time constraint maxTime
seconds.
If the pointer can reach the bottom-right corner within maxTime
seconds, return the string 'Yes'
. Otherwise, return 'No'
.
Function Description
Complete the function reachTheEnd
in the editor.
Function Signature:
def reachTheEnd(grid: List[str], maxTime: int) -> str:
Parameters:
grid
(List[str]): A list of strings representing the rows of the grid, where each string represents a row in the grid.maxTime
(int): An integer representing the maximum time allowed to reach the bottom-right corner of the grid.
Output:
- Return a string:
"Yes"
if the pointer can reach the bottom-right corner withinmaxTime
seconds, otherwise return"No"
.
Example 1:
Input:
grid = ["..#", "#.##", "#..."]
maxTime = 5
Output:
"Yes"
Explanation:
..#
#.##
#...
It will take the pointer 5 seconds to reach the bottom-right corner. As long as maxTime >= 5
, return 'Yes'
.
Example 2:
Input:
grid = ["..", ".."]
maxTime = 3
Output:
"Yes"
Explanation:
..
..
The grid has 2 rows and 2 columns, and the time within which the pointer needs to reach the bottom-right cell is 3 seconds. Starting from the top-left cell, the pointer can either move to the top-right unblocked cell or bottom-left unblocked cell, and then to the bottom-right cell. It takes 2 seconds to reach the bottom-right cell on either path. Thus, the pointer reaches the bottom-right cell within 3 seconds, so the answer is "Yes"
.
Example 3:
Input:
grid = [".#", "#."]
maxTime = 2
Output:
"No"
Explanation:
.#
#.
The grid has 2 rows and 2 columns, and the time within which the pointer needs to reach the bottom-right cell is 2 seconds. It can neither move to the top-right cell nor to the bottom-left cell in 2 seconds, so the pointer cannot reach the bottom-right cell within the time limit. Therefore, the output is "No"
.
Constraints:
- 1 ≤ rows ≤ 500
- 1 ≤ maxTime ≤ 100,000
This version of the problem presents the task without LaTeX and focuses on explaining the input/output format and the problem's logic in plain text.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
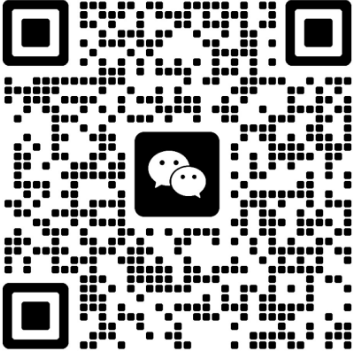