Technical interviews often involve designing and implementing real-world tools. Today, we’ll break down a challenging Amazon interview question that requires building a simplified version of the UNIX find
command. Through this detailed walkthrough, we’ll demonstrate how a candidate successfully navigates the interview, supported by real-time assistance from csoahelp, a powerful tool designed to help candidates perform their best.
The Problem
Implement the functionality of the "find" command in UNIX as an API that returns a list of files based on the provided search parameters (Search should be limited to name and size only).
Requirements:
- Find all files that start with "abc".
- Find all files that are greater than or equal to 2MB in size.
Additional Information:
The interviewer provided a File
object with the following methods:
getName()
- Retrieves the name of a file or directory.getSize()
- Retrieves the size of a file.isDir()
- Checks if a given path is a directory.listFiles()
- If initialized with a directory, returns a list of files.
The Interview: A Step-by-Step Breakdown
Interviewer: Today, we’re going to design an API inspired by the UNIX find
command. Your task is to identify files in a directory that match the following criteria:
- The file name starts with "abc".
- The file size is greater than or equal to 2MB.
Does the problem make sense to you?
Candidate: Yes, I think I understand the requirements. To ensure I’m clear, let me ask a few questions:
- Should the search traverse subdirectories recursively?
- Is file size measured in bytes?
- What should I do if the input path is a file and not a directory?
Interviewer: Good questions! The search must be recursive. File size is measured in bytes, and you can assume 2MB equals 2,000,000 bytes. If the input path is a file, you should check it directly against the conditions.
csoahelp (real-time prompt):
"Ask if there are any edge cases, like empty directories or no matching files. This will demonstrate your attention to detail."
Candidate: Great, I also want to confirm edge cases. For example:
- What should I return if the directory is empty or no files match the criteria?
- Are there any special rules for hidden files?
Interviewer: If the directory is empty or no files match, return an empty list. You can ignore hidden files for now. Shall we move on?
Candidate: (Explaining their approach)
Here’s how I plan to solve this:
- I’ll first check if the input path is a directory. If it is, I’ll recursively traverse all files and subdirectories.
- For each file, I’ll evaluate the two conditions:
- Whether the name starts with "abc".
- Whether the size is greater than or equal to 2MB.
- If a file matches either condition, I’ll add it to a result list.
- At the end, I’ll return the list of matching files.
Interviewer: That sounds reasonable. But recursion can sometimes cause performance issues, especially with deeply nested directories. How would you handle that?
csoahelp (real-time prompt):
"Propose using an iterative approach with an explicit stack to simulate recursion. This will help avoid stack overflow."
Candidate: You’re right—deep recursion can lead to stack overflow. To avoid this, I can use an iterative approach by maintaining an explicit stack to handle directories. This way, I can control the depth of the traversal and prevent potential memory issues.
Interviewer: Good thinking. Let’s discuss the time and space complexity of your solution.
Candidate:
- Time complexity: O(n), where n is the total number of files and directories, as each one needs to be visited once.
- Space complexity: O(d), where d is the maximum directory depth, since the stack size will depend on the depth.
csoahelp (real-time prompt):
"Highlight additional factors like I/O costs for retrieving file details, to show a deeper understanding of real-world constraints."
Candidate: In addition, I’d like to point out that the time complexity can be influenced by I/O overhead, such as retrieving file details like names and sizes. These operations depend on the file system’s performance.
Interviewer: Excellent. Now, what if the results need to be sorted by file size?
Candidate: After collecting all matching files, I would sort the result list using a comparator based on file size. Java’s Collections.sort
or a custom comparator would handle this efficiently.
Interviewer: Let’s switch gears. Tell me about a challenging project you’ve worked on and how you approached it.
Candidate: During an internship, I worked on a log analytics system that had to process massive datasets in real time. My main task was to optimize the log parsing module. By redesigning the parsing logic and implementing multi-threading, I reduced processing time by 40%.
csoahelp (real-time prompt):
"Discuss how you validated the solution, such as using benchmarks or testing on production-like data."
Candidate: To ensure the improvements were effective, I benchmarked the performance on simulated datasets that mimicked production workloads. This allowed me to quantify the impact of my changes and verify their scalability.
Interviewer: Impressive. Any questions for me?
Candidate: Thank you for the opportunity! I’d love to learn more about how Amazon’s engineering teams handle large-scale data processing challenges.
How csoahelp Made the Difference
Throughout the interview, csoahelp provided real-time guidance that significantly enhanced the candidate’s performance:
- Clarifying the problem: Suggested insightful edge-case questions to show attention to detail.
- Optimizing the solution: Introduced the idea of using an explicit stack to handle recursion safely.
- Analyzing complexity: Encouraged the candidate to discuss practical factors like I/O overhead, demonstrating a strong understanding of real-world constraints.
- Enhancing project answers: Helped the candidate highlight validation methods and quantify performance improvements in behavioral questions.
Conclusion
This example highlights the importance of thorough preparation and real-time adaptability in technical interviews. With csoahelp’s guidance, candidates can confidently tackle complex questions, articulate their thought process, and leave a lasting impression.
If you’re preparing for a technical interview, csoahelp is your secret weapon to excel in both technical and behavioral questions. Don’t leave your success to chance—let csoahelp pave the way to your dream job!
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
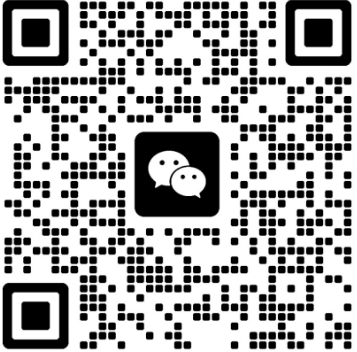