Colors are often expressed as "RGB" hexadecimal triplets such as (AB,12,88), where the positions of the expression correspond to the intensity of (Red, Green, Blue) components of that color.
Write a function that takes an RGB hexadecimal triplet as a string parameter formatted as "(RR,GG,BB)", parses and validates the parameter, and returns the color group based on the following table. Do not use a built-in hexadecimal parsing function.
Condition | Color Group |
---|---|
If all three values are equal | Gray |
If two values are equal and greater than the other: | |
R = G, greater than B | Orange |
R = B, greater than G | Purple |
G = B, greater than R | Cyan |
If one value is greater than the others: | |
R is greatest | Red |
G is greatest | Green |
B is greatest | Blue |
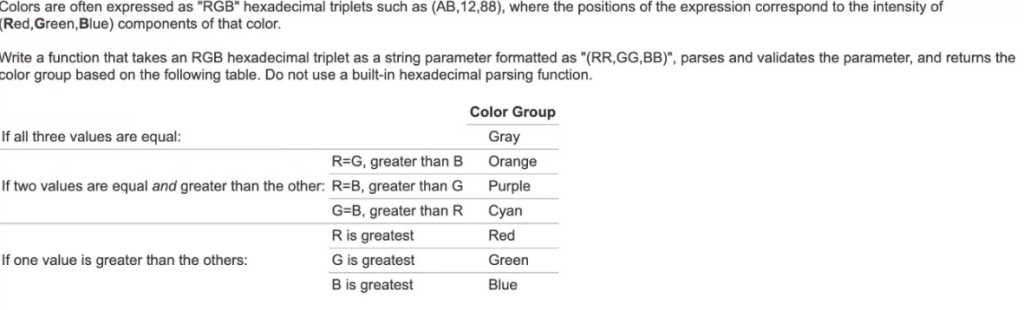
#2: Contagion
You are asked by a government organization to write a program to track a contagious disease that is easily transmitted by handshake at a dinner party. One attendee has come to the party unknowingly infected with the disease. Once the infected attendee shakes hands with another attendee, the other attendee carries the disease and spreads it to additional attendees through more handshakes during the party. The attendees mingle with each other, one-on-one, and move around the party at a given, regular signal. The attendees shake hands at the beginning of each one-on-one interaction.
The government trackers will provide you with an input array listing the interactions between the attendees. The array contains a sub-array for each attendee. The n-th
cell of the sub-array lists the person that attendee interacted with at the n-th
step. An attendee may choose not to interact with anyone at a given interval, which appears as -1
in the list.
For example, if there are four guests and five rounds of interaction, the array might look like this:
[
[3, 4, -1, -1, -1],
[-1, 3, 3, -1, 4],
[1, 2, 2, 4, -1],
[-1, 1, -1, 3, 2]
]
In the example above, if attendee 2
was the original disease carrier, the list of disease carriers at the end of the party would be [2, 3, 4]
.
Write a function which returns the list of disease carriers given the input array and the initial disease carrier. You may assume the array is correctly formatted.
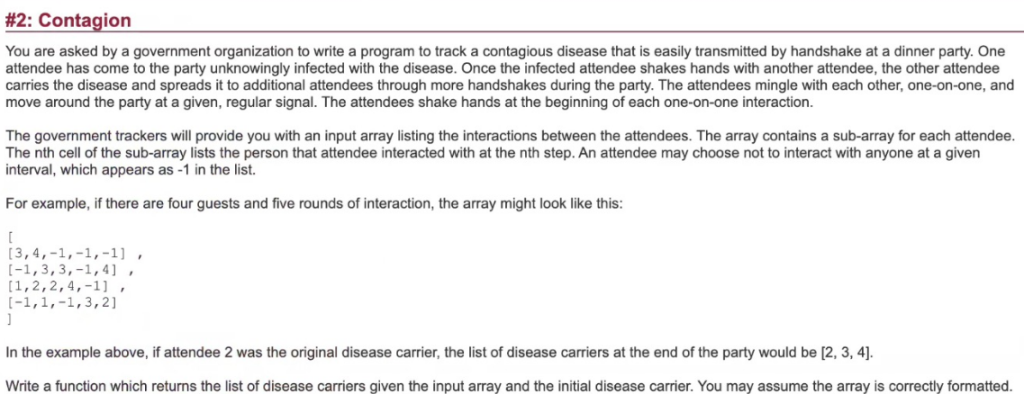
#3: Hacker
The number 138 is called "well-ordered" because the digits in the number (1,3,8) increase from left to right (1 < 3 < 8). The number 365 is not well-ordered because 6 is larger than 5.
You are ch40t1c-g00d, an elite anti-hacker. An informant has revealed that an evil hacker ring's password is a well-ordered number; another informant has revealed the number of digits in the password. Write a function which, given the number of digits as a parameter, displays all possible well-ordered numbers with that many digits.

The game "Jumpers"
The game "Jumpers" is played by two players on a board configured in an N x N
grid. Each space in the board can be unoccupied or occupied by a piece from either player.
During a player’s turn, the player attempts to jump over as many of the opponent’s pieces as possible. A piece can jump over a single opponent’s horizontally or vertically adjacent piece if the landing space (the next space in the same direction) is unoccupied. After landing, the same piece can immediately jump over another opponent’s piece to which it is now horizontally or vertically adjacent, and so forth until no more jumps are possible for that piece. The player’s piece cannot jump over the same piece more than once.
Write a function which finds the number of jumps in the longest possible jump path for a piece at a given location on a board. The two-dimensional array representing the board and the coordinates of the specific piece will be passed into the function as parameters. In the array, 0
denotes an unoccupied space, 1
denotes a space in which Player 1 has a piece, and 2
denotes a space in which Player 2 has a piece. You may assume the input is valid.
For example, if this is the board:
0 2 0 0
2 0 2 0
0 1 1 2
0 2 1 0
and (2,2)
is the given location, the number of jumps in the longest possible path is 3 since the piece at that location can jump up, then left, and then down.
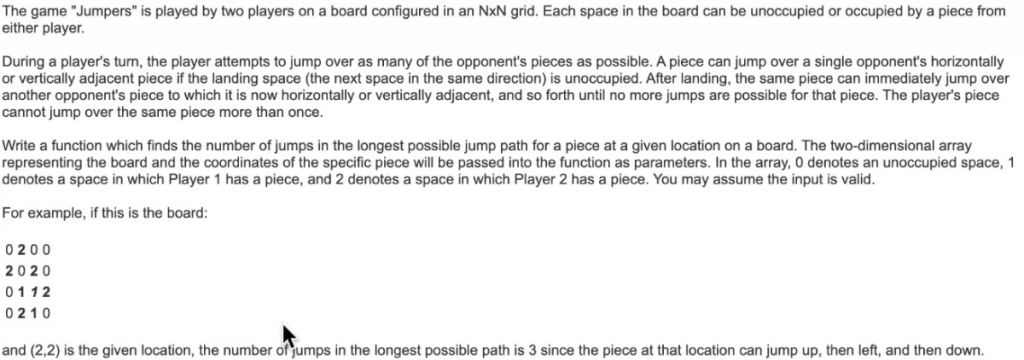
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
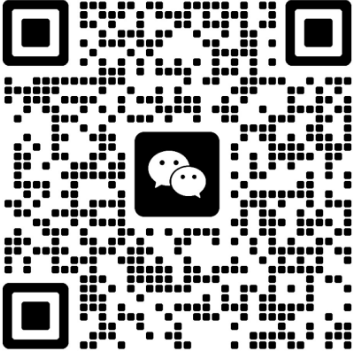