Apple's technical interviews are known for their rigor, assessing a candidate's ability to design and optimize algorithms. In this blog, we'll break down two common interview problems: checking if two binary trees are identical and moving all occurrences of a specific number to the end of an array. We'll also highlight how CSOAHelp can empower candidates to excel in such high-pressure situations.
Problem 1: Check if Two Binary Trees are Identical
Problem Statement:
Implement a function
isIdentical(TreeNode root1, TreeNode root2)
to check if two binary trees are identical.
Two binary trees are considered identical if they are structurally identical and the nodes have the same value.
- Time Complexity: O(n), where n is the total number of nodes.
- Space Complexity: O(n) in the worst case (skewed tree), and O(log(n)) on average for balanced trees.
Clarifying the Problem
In the interview, the candidate begins by asking clarifying questions to ensure a correct understanding of the problem:
Candidate:
“Should two empty trees be considered identical?”
Interviewer:
“Yes, two empty trees are identical.”
Candidate:
“What if one tree is empty and the other is not? Should the function return false
in that case?”
Interviewer:
“Correct. If one tree is empty but the other isn’t, they are not identical.”
How CSOAHelp Supports You:
We train candidates to identify edge cases in tree problems, such as when one or both trees are empty. This preparation ensures that candidates are thorough in their clarifications, avoiding missteps during the actual coding phase.
Solution Discussion
Candidate:
“My solution uses recursion to traverse both trees simultaneously. At each step, I check the following:
- If both nodes are
null
, returntrue
. - If one node is
null
but the other is not, returnfalse
. - If the values of the current nodes are different, return
false
. - Otherwise, recursively check the left and right subtrees.”
Interviewer:
“That makes sense. Can you explain the time and space complexity of your approach?”
Candidate:
“The time complexity is O(n) because we visit each node once. The space complexity is O(n) in the worst case (for a completely skewed tree), but it’s O(log(n)) for a balanced tree because the recursive call stack depth corresponds to the height of the tree.”
How CSOAHelp Supports You:
We provide candidates with visual tools to understand recursion in binary trees and train them to articulate their solutions step-by-step. Our mock interview sessions help candidates build confidence in explaining their logic and complexity analysis.
Problem 2: Move All 8s to the End of an Array
Problem Statement:
Implement a function
moveEightsToEnd(int[] nums)
to move all occurrences of the number 8 to the end of the array while maintaining the relative order of other elements.
- Time Complexity: O(n).
- Space Complexity: O(1).
Clarifying the Problem
Candidate:
“Am I allowed to modify the input array directly, or should I create a new array for the result?”
Interviewer:
“You need to modify the input array directly.”
Candidate:
“Can I assume that the input array is always valid and non-empty?”
Interviewer:
“Yes, you can assume the input is valid.”
How CSOAHelp Supports You:
During practice sessions, we prepare candidates to clarify constraints and assumptions, such as whether input arrays are non-empty or whether in-place modifications are required. This helps avoid misunderstandings and ensures a clear problem-solving strategy.
Solution Discussion
Candidate:
“I will use a two-pointer approach:
- One pointer,
nonEightIdx
, tracks the next position for non-8 elements. - Another pointer,
i
, iterates through the array.
If an element is not 8, I swap it with the element atnonEightIdx
, then incrementnonEightIdx
. This preserves the order of non-8 elements.”
Interviewer:
“That’s efficient. Why is your solution O(n) in time complexity?”
Candidate:
“The algorithm traverses the array exactly once, performing constant-time swaps for non-8 elements. Hence, the time complexity is O(n).”
Interviewer:
“And the space complexity?”
Candidate:
“The space complexity is O(1) because we don’t use any additional data structures beyond a few variables.”
How CSOAHelp Supports You:
Our training sessions include detailed walkthroughs of two-pointer techniques, ensuring candidates can clearly explain their approach. We also provide practical examples so candidates can confidently discuss both correctness and complexity.
Behavioral Questions: Highlighting Problem-Solving Skills
Interviewer:
“Can you share an example of solving a challenging problem under resource constraints?”
Candidate:
“In a recent team project, we faced performance issues in a data pipeline due to high data volume. I identified bottlenecks and introduced caching to optimize critical parts of the process. This reduced processing time by 50%, demonstrating the importance of resource prioritization and teamwork.”
How CSOAHelp Supports You:
We prepare candidates for behavioral questions using the STAR method (Situation, Task, Action, Result), ensuring their answers are well-structured and impactful. Candidates learn to emphasize their problem-solving abilities and collaborative skills effectively.
Conclusion
With the support of CSOAHelp, this candidate successfully tackled Apple’s technical interview. From clarifying problem requirements to discussing complexity analysis, each step was carefully practiced and refined. The candidate not only demonstrated strong algorithmic skills but also excelled in explaining their approach and answering behavioral questions.
If you’re preparing for technical interviews, CSOAHelp can provide personalized training sessions, mock interviews, and expert guidance to help you excel in every aspect of the interview process.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
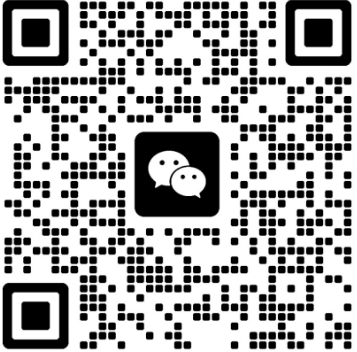