Smart pointers and memory management are central topics in C++ interviews, as they test a candidate's understanding of modern C++ features and best practices. In this blog, we will break down a Verisk interview question focusing on smart pointers, analyze the differences between manual memory management and modern practices, and demonstrate how CSOAHelp equips candidates to excel in these scenarios.
Problem Overview: The Question Presented by the Interviewer
Problem Statement:
#include
struct Base {
virtual void f();
};
struct Derived : Base {};
void f() {
std::unique_ptr b = std::make_unique();
}
void f() {
Base *b = new Derived();
// ...
delete b;
}
int main() {
f();
return 0;
}
The question primarily aims to compare the use of std::unique_ptr
with manual memory allocation and deallocation, exploring the implications of each approach.
Step 1: Clarifying the Problem
The candidate began by asking clarifying questions to understand the requirements better:
Candidate:
“Is the goal here to compare the behavior of std::unique_ptr
with manual memory management, or should we focus on identifying and mitigating potential risks in the provided code?”
Interviewer:
“The focus is on understanding how smart pointers can prevent common pitfalls of manual memory management.”
Candidate:
“Got it. Should we analyze the memory release behavior in detail and highlight any potential risks in the code?”
Interviewer:
“Yes, analyze the code for potential risks and suggest optimizations.”
CSOAHelp’s Role:
We train candidates to identify key areas of focus in such scenarios, such as comparing modern C++ practices with traditional ones, and help them ask precise questions to align their answers with the interviewer’s expectations.
Step 2: Analyzing the Code and Suggesting Solutions
After clarifying the problem, the candidate proceeded to analyze the code and offer solutions:
Candidate:
“The first code snippet uses std::unique_ptr
and std::make_unique
, which are part of modern C++ practices. This ensures that the memory allocated for the Derived
object is automatically released when the function scope ends, preventing memory leaks.”
Interviewer:
“Good observation. What about the second snippet?”
Candidate:
“The second snippet uses manual memory allocation with new
and delete
. This approach is error-prone because if an exception is thrown before delete
is called, the allocated memory will not be released, resulting in a memory leak.”
Interviewer:
“How can this issue be mitigated?”
Candidate:
“We can replace manual memory management with std::unique_ptr
. Smart pointers ensure that memory is automatically released when the pointer goes out of scope, even if an exception occurs.”
CSOAHelp’s Role:
Our training emphasizes the importance of clearly explaining why modern C++ features like std::unique_ptr
are preferable. We also provide candidates with real-world examples to help them articulate the benefits of automated memory management.
Step 3: Follow-Up Questions and Edge Case Handling
The interviewer followed up with deeper questions to evaluate the candidate’s understanding of smart pointers and polymorphism:
Interviewer:
“In the first snippet, what issues might arise if we don’t use std::make_unique
but instead create the std::unique_ptr
manually?”
Candidate:
“If we manually create a std::unique_ptr
using new Derived()
, there’s a risk of memory leaks if an exception is thrown between the allocation and the std::unique_ptr
initialization. std::make_unique
avoids this risk by combining allocation and initialization into a single atomic operation.”
Interviewer:
“Great. What if you need to share this object across multiple functions or threads?”
Candidate:
“In that case, we would use std::shared_ptr
instead of std::unique_ptr
. However, we should be cautious about the performance overhead of maintaining reference counts with std::shared_ptr
. If unique ownership can be guaranteed, std::unique_ptr
remains the optimal choice.”
CSOAHelp’s Role:
We guide candidates on how to address such advanced questions by explaining the specific use cases and trade-offs of std::unique_ptr
and std::shared_ptr
. This ensures they can confidently handle deeper explorations of these concepts.
Step 4: Summarizing Complexity and Optimizations
The candidate then provided a concise summary of their findings and proposed optimizations:
Candidate:
“The first snippet, using std::unique_ptr
, represents modern C++ best practices. It ensures automatic memory management, reducing the risk of leaks. In contrast, the second snippet’s reliance on manual memory management introduces the potential for memory leaks or dangling pointers if delete
is not correctly executed.”
Interviewer:
“Well summarized. Are there additional optimizations you’d recommend?”
Candidate:
“We can further validate memory management by adding unit tests to cover all execution paths, including exception handling scenarios. Additionally, tools like Valgrind can be used to detect and fix any potential memory issues.”
CSOAHelp’s Role:
We help candidates practice summarizing their findings effectively and suggesting actionable optimizations, showcasing a comprehensive understanding of both the problem and the solution.
Step 5: Addressing Behavioral Questions
Interviewer:
“Can you share an example of when you improved the quality or maintainability of a codebase?”
Candidate:
“In a recent project, I identified numerous instances of manual memory management that led to recurring memory leaks. I proposed transitioning to smart pointers and trained the team on their use. This change significantly improved code maintainability and eliminated memory issues, making the system more robust.”
CSOAHelp’s Role:
We provide structured mock behavioral interviews, helping candidates craft compelling stories using the STAR framework (Situation-Task-Action-Result). This enables them to effectively highlight their technical contributions and leadership.
Conclusion
With CSOAHelp’s expert coaching, this candidate confidently tackled Verisk’s C++ interview question. From analyzing memory management issues to recommending modern solutions, the candidate demonstrated their technical expertise and ability to communicate effectively. CSOAHelp’s personalized training ensures that candidates are prepared to excel in every aspect of their interviews.
If you’re preparing for a challenging technical interview, CSOAHelp can provide tailored guidance to help you succeed. From mastering advanced C++ concepts to handling tricky behavioral questions, we’ll ensure you’re ready to shine.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
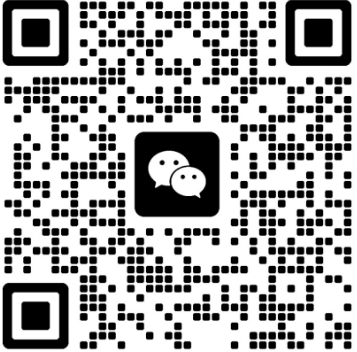