Meta's technical interviews are known for their logical rigor and high expectations for algorithm design. This article documents a candidate's complete interview experience at Meta, tackling two challenging problems—Finding a Local Minimum and Continuous Subsequence Sum. With csoahelp's real-time support, the candidate navigated through these questions confidently, addressing follow-up queries and behavioral questions along the way.
Problem 1: Finding a Local Minimum
The original problem is as follows:
Given an array of integers, find any one local minimum from the array.
A local minimum is defined as an integer in the array that is less than or equal to its neighbors.
Examples:
[3, 2, 1] => 1
[5, 9, 10, 7, 12] => 5 or 7
Interview Walkthrough
Clarifying the Problem: Understanding the Local Minimum
The candidate began by clarifying the problem with the interviewer, guided by csoahelp's prompts.
- Candidate's Questions:
- “Is the array guaranteed to be non-empty?”
- “For boundary elements, how should neighbors be considered?”
- “If there are multiple local minima, can we return any one of them?”
- Interviewer’s Responses:
- The array is guaranteed to be non-empty.
- Boundary elements only have one neighbor, and the definition applies accordingly.
- Yes, returning any one local minimum is acceptable.
- csoahelp’s Real-Time Guidance:
- “Clarify boundary conditions, such as single-element arrays or cases where the minimum is at the edges.”
- “Confirm the expected time complexity—this will help frame your solution.”
Thanks to csoahelp’s guidance, the candidate avoided misunderstandings and demonstrated attention to detail, building rapport with the interviewer.
Solution Design: From Brute Force to Optimized Binary Search
The candidate presented two solutions, starting with a straightforward approach and progressing to a more efficient method.
- Candidate’s Proposed Solutions:
- Linear Scan:
- Iterate through the array and check each element against its neighbors.
- Return the first element that satisfies the local minimum condition.
- Time complexity: O(n), Space complexity: O(1).
- Binary Search:
- Compare the middle element with its neighbors:
- If the middle element is greater than its right neighbor, a local minimum exists in the right half.
- Otherwise, a local minimum exists in the left half.
- Recursively or iteratively narrow down the range until a local minimum is found.
- Time complexity: O(log n), Space complexity: O(1).
- Compare the middle element with its neighbors:
- Linear Scan:
- csoahelp’s Real-Time Input:
- “Linear scan is a good starting point to validate your understanding, but the interviewer may expect an optimized solution.”
- “For binary search, provide concrete examples to illustrate how the search space is reduced.”
- “Highlight edge cases, such as arrays with a single element or strictly increasing/decreasing sequences.”
By clearly explaining both approaches and their trade-offs, the candidate effectively conveyed their problem-solving process.
Problem 2: Continuous Subsequence Sum
The second problem was presented as follows:
Given a sequence of non-negative integers and an integer total target, return whether a continuous sequence of integers sums up to target.
Examples:
[1, 3, 1, 4, 23], 8 => True (because 3 + 1 + 4 = 8)
[1, 3, 1, 4, 23], 7 => False
Clarifying the Problem: Understanding Input and Output
The candidate once again clarified key details with the interviewer.
- Candidate's Questions:
- “Are all inputs guaranteed to be non-negative integers?”
- “Can the target value be 0?”
- “If multiple subsequences satisfy the condition, do we only need to return
True
?”
- Interviewer’s Responses:
- Yes, all inputs are non-negative integers.
- The target can be 0, and only an empty subsequence would satisfy this condition.
- Yes, returning
True
for any valid subsequence is sufficient.
- csoahelp’s Real-Time Guidance:
- “Ask about edge cases like empty arrays or single-element arrays.”
- “Clarify the handling of the special case where the target is 0.”
With these clarifications, the candidate confidently moved forward with their solution.
Solution Design: Sliding Window Approach
The candidate proposed an efficient sliding window solution.
- Candidate’s Proposed Solution:
- Initialize two pointers to represent the start and end of the current window, with an initial sum of 0.
- Expand the window by moving the right pointer to increase the sum.
- Shrink the window by moving the left pointer if the sum exceeds the target.
- If the sum equals the target at any point, return
True
. - If the loop completes without finding a match, return
False
. - Time complexity: O(n), Space complexity: O(1).
- csoahelp’s Real-Time Input:
- “Sliding window is an excellent choice here. Use an example to demonstrate how the pointers move.”
- “Highlight that this approach works efficiently because the input contains only non-negative numbers.”
- “Explain the limitations of this method, such as its inapplicability to arrays with negative numbers.”
The candidate used a step-by-step example to clearly explain the sliding window process, impressing the interviewer.
Follow-Up Questions: Handling Variations and Extensions
The interviewer then posed additional questions to test the candidate’s understanding and ability to adapt their solutions.
- Interviewer’s Questions:
- “How would your solution change if the array contained negative numbers?”
- “Can you modify the algorithm to return all subsequences that sum up to the target?”
- “For very large sequences, how can you optimize memory usage?”
- Candidate’s Responses:
- “If the array contains negative numbers, sliding window may not work. I would use prefix sums or dynamic programming instead.”
- “To return all subsequences, I would iterate through the array, tracking all valid subsequences dynamically.”
- “For large sequences, I would use an online processing approach to avoid storing the entire array in memory.”
- csoahelp’s Real-Time Input:
- “For negative numbers, quickly acknowledge the limitation of sliding window and suggest an alternative.”
- “For memory optimization, emphasize the importance of processing data in chunks or streams.”
The candidate’s thoughtful responses demonstrated adaptability and a deep understanding of the problem space.
Behavioral Questions: Teamwork and Time Management
After the technical discussion, the interviewer transitioned to behavioral questions to assess the candidate’s soft skills.
- Interviewer Asked:
- “Tell me about a time when you had to prioritize competing tasks.”
- “How do you handle disagreements in a team setting?”
- Candidate’s Responses:
- “During a previous project, I had to manage both development and testing tasks. I prioritized by identifying critical deliverables and delegating less critical tasks to teammates. This ensured the project stayed on schedule.”
- “When faced with team disagreements, I encourage open discussions to understand everyone’s perspectives. In one instance, this approach led us to combine two proposed solutions, which ultimately improved the project outcome.”
- csoahelp’s Real-Time Suggestions:
- “Use the STAR framework (Situation, Task, Action, Result) to structure your answers for clarity.”
- “Highlight your ability to collaborate and make data-driven decisions.”
Conclusion: How csoahelp Ensures Interview Success
Throughout this Meta interview, csoahelp provided invaluable real-time support:
- Guided the candidate in clarifying problems and avoiding misunderstandings.
- Helped frame solutions, from simple implementations to optimized approaches.
- Prepared the candidate for follow-up questions, ensuring they addressed edge cases and extensions comprehensively.
- Shaped clear and structured responses to behavioral questions, showcasing teamwork and leadership.
If you’re preparing for technical interviews at top companies like Meta, csoahelp can provide the real-time guidance you need to excel. With tailored support for every stage of the process, csoahelp ensures you stand out and succeed.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
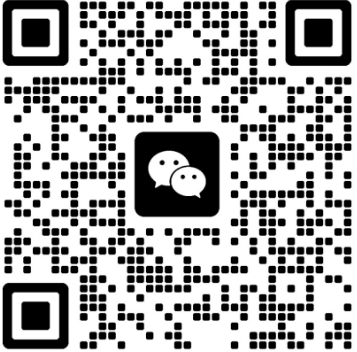