Problem Description
Analysts have determined that people often use personal information in their passwords, which is insecure. A new feature is needed to address this issue.
The feature will:
- Search for the presence of a reference string as a subsequence in any permutation of the password.
- If the reference string is found as a subsequence, determine the minimum cost to remove characters from the password so that no permutation contains the reference string as a subsequence.
Details:
- The cost of removing any character is specified in an array
cost
, with 26 elements.cost[0]
corresponds to the cost of removing'a'
.cost[25]
corresponds to the cost of removing'z'
.
Inputs:
string password
: The password string.string reference
: The string that should not appear as a subsequence in any permutation of the password.int cost[26]
: The costs to remove each character in the lowercase English alphabet.
Output:
long int
: The minimum total cost to remove characters from the password such that no permutation contains the reference string as a subsequence.
Example
Input:
password = "adefgh"
reference = "hf"
cost = [1, 0, 0, 2, 4, 4, 3, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
Explanation:
- Removing either
'h'
or'f'
will ensure thatreference
no longer appears as a subsequence in any permutation. - Removing
'h'
has a cost of 1, while removing'f'
has a cost of 4. - The minimum cost is 1 (removing
'h'
).
Output:
1
Function Signature
def minCost(password: str, reference: str, cost: List[int]) -> int:
pass
Plan for Implementation
- Character Frequency Count:
- Count the frequency of each character in the
password
. - Use these counts to determine the minimum removals required.
- Count the frequency of each character in the
- Subsequence Check:
- Iterate over the characters in the
reference
to determine their presence in thepassword
frequency.
- Iterate over the characters in the
- Cost Calculation:
- Compute the cost of removing each required character.
- Use a greedy approach to minimize the total cost.
- Output the Minimum Cost:
- Return the minimum cost to modify the
password
.
- Return the minimum cost to modify the
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
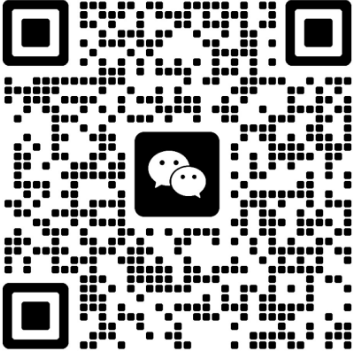