Amazon's technical interviews are renowned for their focus on problem-solving and system design. Recently, a candidate shared their experience of tackling a Product Search System Design challenge during an Amazon interview. This task not only tested their algorithmic skills but also their ability to design scalable and extendable systems.
In this article, we’ll walk through the problem statement, the candidate’s approach, and key takeaways from their experience.
Problem Statement: Designing a Product Search System
The interview question was as follows:
- Amazon products are stored hierarchically within their respective categories.
- Products have various metadata, like current price or whether they are prime-eligible.
- For example, a dress might be located under
Clothing > Women > Dresses
, with a price of $20 and it is prime-eligible. - The system should be easy to add additional criteria to search by in the future.
- Initially, it only needs to handle querying by category path or maximum price.
The goal was to design a solution that could efficiently handle these queries while ensuring that the system could accommodate future requirements, such as additional search filters.
Interview Process Breakdown
1. Clarifying the Problem
The candidate first clarified the requirements:
- Input:
- A category path (e.g.,
Clothing > Women > Dresses
). - A maximum price (e.g.,
$50
).
- A category path (e.g.,
- Output:
- A list of products that match the input criteria.
The interviewer also emphasized that the system should scale to handle a large dataset and support additional filters in the future, such as prime-eligible or brand-specific filtering.
2. Data Structure Design
To store and query product information, the candidate proposed a hierarchical data structure:
- Hierarchical Tree:
- Each node represents a category, and leaf nodes store product data.
- Product Metadata:
- Attributes include product name, price, prime-eligibility, and other details.
Example Structure:
{
"Clothing": {
"Women": {
"Dresses": [
{"name": "Red Dress", "price": 20, "prime_eligible": True},
{"name": "Blue Dress", "price": 45, "prime_eligible": False}
]
}
}
}
3. Query Algorithm
The candidate implemented a straightforward algorithm to traverse the data structure and apply filtering:
- Category Path Traversal:
- Start at the root node and follow the path step-by-step until reaching the desired category.
- Price Filtering:
- Filter the products within the target category based on the given maximum price.
Code Implementation:
def search_products(data, category_path, max_price):
# Split the category path into individual categories
categories = category_path.split(" > ")
current_level = data
# Traverse the hierarchy
for category in categories:
if category in current_level:
current_level = current_level[category]
else:
return [] # Return empty list if path doesn't exist
# Apply price filter
return [
product for product in current_level
if product["price"] <= max_price
]
4. Supporting Extensibility
To address the requirement for adding new search criteria (e.g., prime eligibility), the candidate introduced a dynamic filtering mechanism:
- Dynamic Filters:
- Use a list of filter functions that are applied sequentially to the product list.
- Performance Optimization:
- Maintain sorted lists for attributes like price to enable binary search and reduce complexity.
Enhanced Code:
def search_products(data, category_path, max_price=None, prime_eligible=None):
categories = category_path.split(" > ")
current_level = data
# Traverse the hierarchy
for category in categories:
if category in current_level:
current_level = current_level[category]
else:
return []
# Apply filters dynamically
filters = []
if max_price is not None:
filters.append(lambda p: p["price"] <= max_price)
if prime_eligible is not None:
filters.append(lambda p: p["prime_eligible"] == prime_eligible)
# Apply all filters
for f in filters:
current_level = filter(f, current_level)
return list(current_level)
5. Time Complexity Analysis
The candidate analyzed the time complexity of their solution:
- Category Path Traversal:
- Complexity: O(d)O(d), where dd is the depth of the category path.
- Price Filtering:
- Complexity: O(n)O(n), where nn is the number of products in the target category.
To handle larger datasets, the candidate proposed sorting the products by price during preprocessing, enabling faster queries using binary search.
Highlights of the Candidate’s Approach
- Clear Problem Decomposition:
- The candidate effectively broke down the problem into two components: category traversal and product filtering.
- Focus on Scalability:
- Designed the system to handle large datasets and accommodate future requirements.
- Algorithm and System Thinking:
- Combined efficient algorithms with a practical system design approach.
Takeaways from the Interview
This challenge demonstrated how Amazon evaluates both algorithmic and system design skills in their interviews. The problem was crafted to test a candidate’s ability to:
- Understand the trade-offs between simplicity and scalability.
- Design a system that meets current requirements while being extensible for future needs.
- Optimize performance for large-scale datasets.
By successfully addressing these aspects, the candidate showcased their ability to think like a software engineer at Amazon.
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
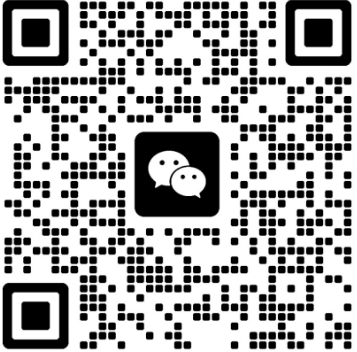