几天前我们公布了部分真题,让大家久等了,接下来我们会公布剩余的真题,OA已经快要结束了,请大家抓住最后的时机。
A few days ago, we released some of the test questions, which made everyone wait for a long time. Next, we will release the remaining test questions. OA is almost over, please seize the last opportunity.
Question 3: Abstraction in Design – Interface Implementation
You are developing an application where multiple payment methods (CreditCard
, PayPal
) need to be processed. Both payment types should implement a standard method for processing payments. Which of the following pseudocode demonstrates proper abstraction through interface implementation?
# option 1
interface Payment:
def processPayment(amount)
class CreditCard implements Payment:
def processPayment(amount):
print("Processing credit card payment for " + amount)
class PayPal implements Payment:
def processPayment(amount):
print("Processing PayPal payment for " + amount)
payment = new CreditCard()
payment.processPayment(1500)
# option 2
interface Payment:
def processPayment(amount)
class CreditCard implements Payment:
def processPayment(amount):
print("Processing credit card payment for " + amount)
class PayPal implements Payment:
def processPayment(amount):
print("Processing PayPal payment for " + amount)
payment = new PayPal()
payment.processPayment(1500)
# option 3
interface Payment:
def processPayment(amount)
class CreditCard implements Payment:
def processPayment():
print("Processing credit card payment")
class PayPal implements Payment:
def processPayment():
print("Processing PayPal payment")
payment = new PayPal()
payment.processPayment()
# option 4
class Payment:
def processPayment(amount):
print("Processing generic payment for " + amount)
class CreditCard extends Payment:
def processPayment(amount):
print("Processing credit card payment for " + amount)
class PayPal extends Payment:
def processPayment(amount):
print("Processing PayPal payment for " + amount)
payment = new PayPal()
payment.processPayment(1500)
Question 4: Object Composition vs. Inheritance
You are tasked with building a system where a Person
class should contain address information but avoid inheritance. Instead, use composition to include an Address
object inside Person
. Which of the following pseudocode properly implements composition?
# option 1
class Address:
def __init__(city, country):
self.city = city
self.country = country
class Person extends Address:
def __init__(name, city, country):
self.name = name
Address.__init__(city, country)
person = new Person("John Doe", "New York", "USA")
print(person.name + " lives in " + person.city)
# option 2
class Address:
def __init__(city, country):
self.city = city
self.country = country
class Person:
def __init__(name, address):
self.name = name
self.address = address
address = new Address("New York", "USA")
person = new Person("John Doe", address)
print(person.name + " lives in " + person.address.city)
# option 3
class Address:
def __init__(city, country):
self.city = city
self.country = country
class Person:
def __init__(name, address):
self.name = name
self.address = address
address = Address("New York", "USA")
person = Person("John Doe", address)
print(person.name + " lives in " + person.address.city)
Q7.Maximize Collaboration
As a data analyst at Trollbox, you've been assigned an innovative project to help maximize creator collaborations for peak viral impact. With so many creators competing for attention, each creator has their own engagement power, which measures how likely they are to boost views, likes, and shares.
Your task is to create collaboration teams where creators join forces to generate ultimate viral content. However, forming these teams follows specific rules:Creators must be adjacent based on their posting order. This means you can only form teams with creators who post one after the other, without skipping anyone. For example, if creators 1, 2, and 3 post consecutively, they can form a team. However, you cannot combine creators 1, 3, and 5 since they are not adjacent in the posting sequence.You need at least a minimum number of creators (given by minCreatorsRequired) to form a valid team.The total engagement power of the creators of the team must meet or exceed a threshold (given by minTotalEngagementPowerRequired) for their collaboration to generate enough buzz to go viral.
Given a list of the creators and their engagement powers, along with the minimum team size and target engagement power, determine the maximum number of collaborations you can create. Remember, each creator can only belong to one collaboration team.
Function Description
Complete the function createMaximumCollaborations
in the editor.
createMaximumCollaborations
has the following parameter(s):
int creatorsEngagementPower[n]
: An array that denotes the engagement power of each creator.int minCreatorsRequired
: The minimum number of creators required in a collaboration.int minTotalEngagementPowerRequired
: The minimum total engagement power required for a collaboration.
Returns
int
: The maximum number of collaborations that can be formed from the creators.
Example 1:
Input: creatorsEngagementPower = [4, 4, 3, 6, 4, 3, 5], minCreatorsRequired = 2, minTotalEngagementPowerRequired = 8
Output: 3
Explanation: One of the optimal ways to form the collaborations is to first use the first and second creators (4 + 4 = 8). Then, take the third and fourth creators (3 + 6 = 9). Finally, take the fifth, sixth, and seventh creators, with a total engagement power of (4 + 3 + 5 = 12).
Example 2:
Input: creatorsEngagementPower = [5, 4, 3, 2, 1], minCreatorsRequired = 3, minTotalEngagementPowerRequired = 20
Output: 0
Explanation: No collaboration is possible in this case because the threshold value is greater than the total sum of the engagement powers of all the creators.
Example 3:
Input: creatorsEngagementPower = [4, 6, 8, 11, 9, 12], minCreatorsRequired = 2, minTotalEngagementPowerRequired = 15
Output: 2
Explanation: There are n = 6 creators. Each collaboration must include at least minCreatorRequired = 2 creators, and the total minTotalEngagementPowerRequired must be equal to 15 😀 The first collaboration includes the first, second and third creators, with a total engagement power of 4 + 6 + 8 = 18. This is above the minTotalEngagementPowerRequiredThe second collaboration includes the fourth and fifth creators, with a total engagement power of 11 + 9 = 20 Therefore, the max num of collaboration groups that can be formed is ✌️ so we return ✌️
Constraints:
1 ≤ n ≤ 10^5
1 ≤ creatorsEngagementPower[i] ≤ 10^9
1 ≤ minCreatorsRequired ≤ n
1 ≤ minTotalEngagementPowerRequired ≤ 10^14
CSOAhelp长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
CSOAhelp consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
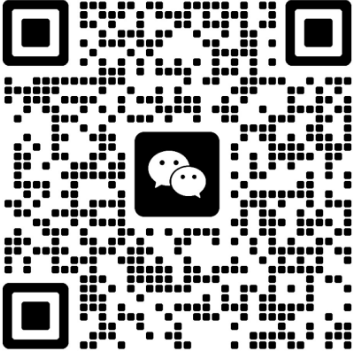