In the highly competitive tech industry, passing a technical interview at a leading company like Meta is a significant challenge. Here’s a detailed account of how one candidate, guided by csoahelp, navigated through Meta’s technical interview with precision and confidence.
Question 1:
Write a function that returns true if a given string is a palindrome (a palindrome is a string that is the same when reversed, if you ignore punctuation and capitalization). Some examples of palindromes are:
- "Race car!"
- "A man, a plan, a canal, Panama!"
The candidate read the problem carefully and asked, "To confirm, the palindrome check should ignore all punctuation and capitalization, correct?"
The interviewer replied, "Yes, you only need to consider alphanumeric characters, and the comparison should be case-insensitive."
The candidate continued, "So the string may contain spaces, punctuation, and a mix of uppercase and lowercase letters, right?"
The interviewer confirmed, "That's correct."
The candidate then began explaining their approach: "I would preprocess the input string to remove all non-alphanumeric characters and convert the remaining characters to a uniform case. This will produce a standardized string. After that, I would use a two-pointer technique to check if the string is a palindrome by comparing characters from both ends moving towards the center. If all characters match, the string is a palindrome."
The interviewer asked, "Can you elaborate on your preprocessing steps and the two-pointer algorithm?"
The candidate explained:
"Certainly. For preprocessing:
- Initialize an empty string
filtered_str
to store valid characters. - Iterate through each character
c
in the input strings
:- If
c
is an alphanumeric character, convert it to lowercase and append it tofiltered_str
.
- If
For the palindrome check using two pointers:
- Set two pointers:
left
starting at the beginning offiltered_str
andright
at its end. - While
left
is less thanright
:- Compare
filtered_str[left]
andfiltered_str[right]
. - If they are not equal, return
false
as the string is not a palindrome. - If they are equal, move
left
one step forward andright
one step backward.
- Compare
- If the loop completes without returning
false
, returntrue
, as the string is a palindrome."
The interviewer followed up, "What’s the time and space complexity of this approach?"
The candidate responded, "The preprocessing step requires a single traversal of the string, which takes O(n), where n is the length of the string. The two-pointer comparison also takes O(n) in the worst case. Thus, the overall time complexity is O(n). Regarding space complexity, storing the preprocessed string requires O(n) additional space, so the space complexity is O(n)."
The interviewer asked, "Can you optimize the space complexity?"
The candidate thought for a moment and said, "Yes, we can avoid creating the filtered_str
altogether by directly applying the two-pointer technique on the original string. The pointers would skip over non-alphanumeric characters and compare valid characters on the fly while converting them to the same case during the comparison. This reduces the space complexity to O(1)."
The interviewer nodded in agreement. "Good. Let’s move on to the next problem."
Question 2:
Given a sequence of positive/0 integers and a positive/0 integer total target, return whether a continuous sequence of integers sums up to target.
Example:
- [1, 3, 1, 4, 23], 8 : True (because 3 + 1 + 4 = 8)
- [1, 3, 1, 4, 23], 7 : False
The candidate carefully reviewed the problem and asked, "To confirm, all numbers in the sequence are non-negative integers, including zero?"
The interviewer replied, "Yes, that’s correct."
The candidate continued, "And we’re looking for a continuous subsequence that sums exactly to the target?"
The interviewer confirmed, "Exactly."
The candidate explained their approach:
"I would use the sliding window technique, leveraging the property that all numbers are non-negative. The idea is to maintain a window of elements that represents the current sum and adjust the window size dynamically to match the target value. Here’s how it works:
- Initialize two pointers,
start
andend
, both at the beginning of the sequence, and setcurrent_sum
to 0. - While
end
is less than the length of the sequence:- Add
sequence[end]
tocurrent_sum
. - If
current_sum
exceeds the target, increment thestart
pointer to shrink the window while subtracting the value atstart
fromcurrent_sum
. - If
current_sum
equals the target, returntrue
. - Increment
end
to expand the window.
- Add
- If the loop completes without finding a valid subsequence, return
false
."
The interviewer asked, "Can you explain why this algorithm is effective?"
The candidate replied, "Since all numbers are non-negative, increasing the end
pointer will only increase the sum, and increasing the start
pointer will only decrease it. This ensures we can efficiently adjust the window size to match the target."
The interviewer asked, "What are the time and space complexities?"
The candidate said, "The time complexity is O(n) because each pointer traverses the sequence at most once. The space complexity is O(1) because we only use a few variables to store the current state."
The interviewer then posed a follow-up question, "What if the sequence contains negative numbers?"
The candidate answered, "If the sequence contains negative numbers, this approach won’t work because the sum can increase or decrease unpredictably. In that case, we would need a different approach, such as using a hash map to track prefix sums. However, this would increase the space complexity."
The interviewer acknowledged, "Good analysis."
Achieving success in technical interviews requires both solid technical knowledge and effective communication skills. With csoahelp, you can gain the support and expertise needed to excel. If you’re aiming to land your dream job, csoahelp is here to guide you every step of the way.
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
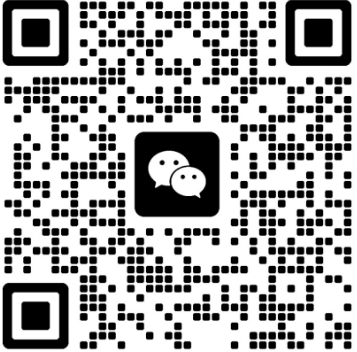