Jane Street is renowned for its rigorous interview process, particularly in testing a candidate's ability to solve complex algorithmic and design problems. These interviews not only evaluate your coding skills but also demand strong logical reasoning and structured thinking. This is where csoahelp comes in—providing comprehensive support to ensure you’re fully prepared to tackle even the most difficult questions.
Let’s look at a sample interview question from Jane Street and see how csoahelp played a vital role in helping a candidate excel.
Interview Question
Simulating a 2D Board Game
Simulate a 2D board game with the following setup:
- The board extends infinitely to the left, right, and top but is limited by the bottom (y = 0).
- Two players, Red ('R') and Blue ('B'), take turns placing their pieces.
- Each move places a piece at the bottom of a specified column (x-coordinate). If the column already contains pieces, they are shifted upwards to make space.
Your task:
1. Implement a `move` function to update the board state.
2. Determine if the current move results in a win for the player:
- Vertical win: `k` consecutive pieces in the same column.
- Horizontal win: `k` consecutive pieces in adjacent columns on the same row.
Recreating the Interview Scene
The candidate joined the virtual interview and listened carefully as the interviewer explained the problem. The candidate began by asking several clarifying questions to ensure a complete understanding of the requirements:
Candidate's Questions:
- "Is the initial board empty?"
- "Do we need to check all possible winning conditions after each move, or just the column where the move was made?"
- "If both players win simultaneously, which result should we prioritize?"
Interviewer’s Responses:
- The board starts empty, stored as a dynamic structure.
- Every move requires checking both vertical and horizontal winning conditions.
- If both players win simultaneously, the function should prioritize the player who achieved the condition first.
Candidate's Algorithm Approach
Data Structure Design
The candidate chose a hashmap to represent the board:
- Key: The x-coordinate of the column.
- Value: A list storing the stack of pieces in that column, where the end of the list represents the bottom of the column.
Example:
board = {
-1: ['R', 'B'],
0: ['B', 'R', 'R'],
1: ['R']
}
This structure supports infinite x-coordinates and allows efficient insertion and retrieval of pieces.
Optimized Functionality
- Placing Pieces: Using the
append()
operation to add a piece at the bottom of the column ensures O(1) insertion time. - Winning Condition Check: Separate logic was implemented to check for both vertical and horizontal wins:
- Vertical Check: Iterating over the same column to count consecutive pieces.
- Horizontal Check: Iterating across adjacent columns on the same row, extending both left and right.
Code Strategy
Before coding, the candidate detailed the steps of the algorithm and explained how edge cases would be handled:
move
Function:- Accepts the x-coordinate and the player making the move.
- Inserts the piece and checks for winning conditions using the
check_win
function.
check_win
Function:- Examines both vertical and horizontal directions for consecutive pieces.
- Includes logic to handle simultaneous victories by returning the first player to meet the condition.
How csoahelp Provided Critical Support
Throughout the preparation and the actual interview, csoahelp offered extensive guidance:
- Preparation Phase:
- Provided mock interview sessions focused on 2D grid simulations and dynamic data structure design.
- Delivered in-depth tutorials on optimizing hashmap usage for game-related problems.
- Real-time Interview Assistance:
- Clarifying Questions: Helped the candidate prepare a list of strategic questions to clarify problem constraints and expectations.
- Algorithm Design: Equipped the candidate with a structured framework to explain their approach logically and clearly.
- Code Implementation: Offered pre-prepared skeleton code and a detailed checklist for handling edge cases, ensuring a smooth coding process.
Sample Code Skeleton:
class GameBoard:
def __init__(self, k):
self.board = {} # A hashmap to store the board state
self.k = k # Number of consecutive pieces required to win
def move(self, x_position, player):
if x_position not in self.board:
self.board[x_position] = []
self.board[x_position].append(player)
return self.check_win(x_position, player)
def check_win(self, x_position, player):
# Check vertical and horizontal win conditions
pass
Success Story
With the help of csoahelp, the candidate successfully navigated the challenging Jane Street interview. By demonstrating a clear understanding of the problem, presenting a well-thought-out algorithm, and handling edge cases confidently, the candidate showcased their expertise and logical thinking.
Why Choose csoahelp?
If you’re preparing for high-stakes technical interviews at top companies like Jane Street, csoahelp is here to give you the edge. From mock interviews and algorithm tutorials to real-time support during interviews, we provide everything you need to succeed.
Get in touch with csoahelp today and take the first step towards landing your dream job!
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
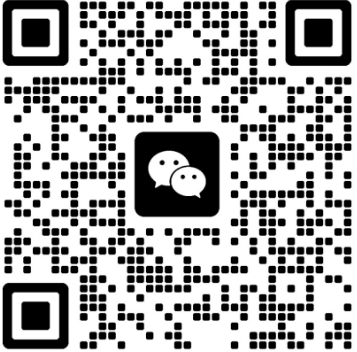