As an organization dedicated to helping job seekers ace their interviews in real-time, csoahelp has once again helped a candidate secure success in a Meta technical interview. This article provides a comprehensive walkthrough of the candidate's interview experience, covering clarifying questions, problem-solving discussions, follow-up answers, time and space complexity summaries, and behavioral questions (BQ).
Problem 1: Continuous Subarray Sum Equals Target
Given a sequence of integers and an integer total target, return whether a continuous sequence of integers sums up to the target.
Example:
[1, 3, 1, 4, 23], 8 → True
(because3 + 1 + 4 = 8
)[1, -3, 1, -4, 23], 7 → False
I carefully read the question and then began to clarify some details with the interviewer.
"May I ask if the array might contain negative numbers?" I inquired.
He nodded and replied, "Yes, the array may contain negative numbers."
I continued, "What is the size of the array? Do we need to consider optimizations for time and space complexity?"
He answered, "Assume the array is of moderate length, but we still hope your solution is as efficient as possible."
I thought for a moment and said, "Since the array contains negative numbers, the traditional Sliding Window method might not be applicable because negative numbers can affect the growth direction of the subarray sum."
He signaled me to continue.
"I'm considering using Prefix Sum and a hash table to solve this problem. Specifically, I can traverse the array, calculate the prefix sum up to the current position, and store each prefix sum in a hash table. If the current prefix sum minus some previous prefix sum equals the target value, then the sum of the subarray from that previous position to the current position equals the target."
The interviewer smiled and said, "That's a good idea. Can you explain the implementation process in detail?"
I continued, "Sure. First, initialize a hash table called prefix_sums
, where the keys are the prefix sums and the values are the corresponding index positions. Initially, set the prefix sum of 0 at position -1. Then, traverse the array and accumulate the prefix sum current_sum
. At each step, calculate current_sum - target
; if this value has appeared in prefix_sums
, it means that the sum of the subarray from the previous index to the current index equals the target."
He nodded, "Understood. What are the time and space complexities of this method?"
I replied, "The time complexity is O(n) because we only need to traverse the array once. The space complexity is also O(n) for storing the hash table."
He then asked, "If the array is very large, how would you optimize the space complexity?"
I thought for a moment and said, "If we need to optimize space, we could consider the Two Pointers method, but since there are negative numbers, we cannot guarantee correctness. Alternatively, we could limit the size of the hash table under certain conditions, but that might sacrifice correctness."
He acknowledged, "Very good. Let's move on to the next question."
He then presented the second question.
Problem 2: Shortest Path in a 2D Array
You are given a game board represented as a 2D array of zeroes and ones. Zero stands for passable positions, and one stands for impassable positions. Design an algorithm to find the shortest path from the top-left corner to the bottom-right corner.
Example Board:
Entrance → 0 0 0 0 0 0 0
0 1 0 0 1 0 0
0 1 0 1 1 0 0
0 1 0 1 0 1 0
1 1 1 0 0 0 → Exit
A possible path is:
Entrance → + + + + 0 0 0
0 1 + 0 1 0 0
0 1 + 1 1 0 0
0 1 + 1 0 1 0
1 1 1 + + + + → Exit
Assuming a zero-indexed grid of rows and columns, with (0, 0) at the top-left corner (Entrance), we'd return:
(0, 0) → (0, 1) → (0, 2) → (0, 3) → (1, 3) → (2, 3) → (3, 3) → (4, 3) → (4, 4) → (4, 5) → (4, 6)
I first confirmed, "Can I only move in the four basic directions: up, down, left, and right?"
He answered, "Yes, only the four basic directions."
I asked again, "Is it possible that the destination is unreachable?"
He nodded, "Yes, you need to consider that possibility."
I began to explain my thinking, "I plan to use the Breadth-First Search (BFS) algorithm to solve this problem because BFS can find the shortest path from the starting point to the endpoint."
He signaled me to elaborate.
"Specifically, I'll use a queue, initially adding the starting coordinate to it. At the same time, I'll create a visited
2D array of the same size to record visited positions and prevent revisiting. At each step, I dequeue the current coordinate and check its neighbors in the four directions. If a neighbor is passable (value is 0) and hasn't been visited, I'll add it to the queue and mark it as visited. Meanwhile, I'll record the path length. During the traversal, if we reach the endpoint, we'll return the current path length."
He asked, "How do you handle obstacles?"
I replied, "When checking neighbors, if we find a value of 1, indicating an obstacle, we'll skip that neighbor and not proceed further with it."
He continued, "What's the time and space complexity of this algorithm?"
I answered, "The time complexity is O(mn), where m and n are the number of rows and columns of the 2D array because, in the worst case, we need to visit every position. The space complexity is also O(mn) for storing the queue and the visited markers."
He nodded in satisfaction.
Next, the interviewer moved on to the behavioral questions segment.
He asked me, "Can you share an experience where you resolved a conflict within a team?"
I thought for a moment and said, "In a previous project, our team had differing opinions on technology selection. Some wanted to use technology A, while others preferred technology B. To prevent team division, I proactively organized a meeting where everyone could present their viewpoints and reasons. Ultimately, through discussion and combining the strengths of both technologies, we chose a solution that better suited the project requirements."
He continued, "What did you learn from this experience?"
I replied, "I learned the importance of listening and communication. In team collaboration, understanding others' perspectives and finding common ground can solve problems more effectively."
The interviewer smiled and said, "Very good. Do you have any questions for me?"
I seized the opportunity and asked, "Thank you for your time. I'd like to know what kind of support Meta provides for the training and growth of new employees?"
He answered, "We have a comprehensive training system and mentorship program. New employees will have experienced colleagues as mentors to help you quickly integrate into the team and enhance your skills."
As the interview drew to a close, I thanked the interviewer for his time and answers. The entire interview process made me feel both nervous and excited.
Reflecting on this interview, I deeply appreciate csoahelp's assistance. Under their guidance, I not only consolidated my algorithm knowledge but also improved my interview skills and adaptability. They tailored practice plans based on Meta's interview style, allowing me to handle the actual interview with ease.
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
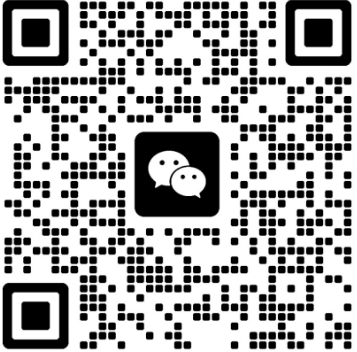