Original Problem Statement
Flood Fill Implementation
Given a 2-dimensional 2-color bitmap, write a function to implement "flood fill," e.g., the "bucket" in MS Paint. Assume that the fill will only fill "white" pixels with "black."
The function should take the following parameters:
- A 2D array of booleans representing the pixels in the bitmap.
- The X and Y position of the start point for the fill.
For example, in Java:
public void floodFill(boolean[][] bitmap, int xStart, int yStart)
Breaking Down the Problem and How We Helped
This classic Flood Fill problem is often used in technical interviews to assess a candidate's knowledge of recursion, depth-first search (DFS), breadth-first search (BFS), and boundary conditions. It mimics the "bucket fill" functionality in graphic design tools like Microsoft Paint.
Here's how CSOAHelp assisted the candidate in solving this problem step by step, optimizing their approach, and preparing for additional clarifications and edge cases during the interview.
Step 1: Understanding the Problem
We started by discussing the problem with the candidate, clarifying key aspects with questions such as:
- Is the starting point always valid?
- The candidate confirmed that the starting point would always fall within the bitmap bounds.
- What are the constraints on the bitmap?
- It only contains two colors, represented as
true
(white) andfalse
(black).
- It only contains two colors, represented as
- Is recursion allowed, and are there limitations?
- The interviewer allowed recursion but wanted the candidate to address potential stack overflow issues.
- Is optimization needed for large bitmaps?
- Yes, the interviewer hinted that solutions requiring less memory would be favored.
With these clarifications, we guided the candidate to approach the problem systematically.
Step 2: Designing the Solution
We encouraged the candidate to explore both recursive and iterative solutions, emphasizing their respective pros and cons.
Recursive Approach
The candidate started with a straightforward recursive implementation:
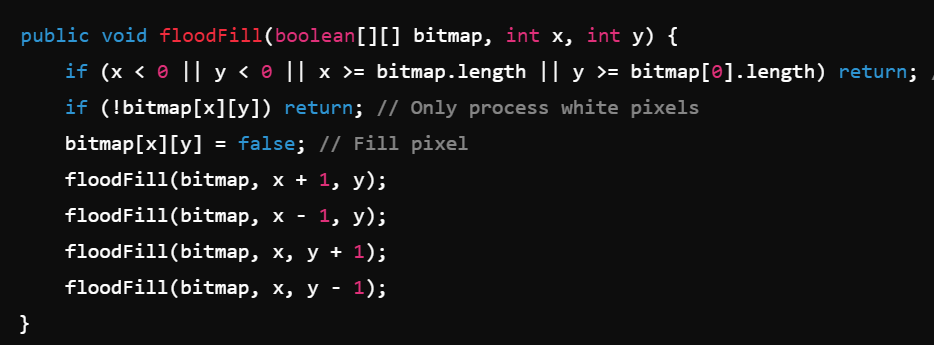
We highlighted two key issues:
- Stack Overflow: For large bitmaps, deep recursion could lead to a stack overflow.
- Lack of Input Validation: The implementation did not handle invalid or empty inputs.
Step 3: Optimizing with an Iterative Approach
To address the recursion depth issue, we suggested converting the solution to an iterative BFS approach using a queue:
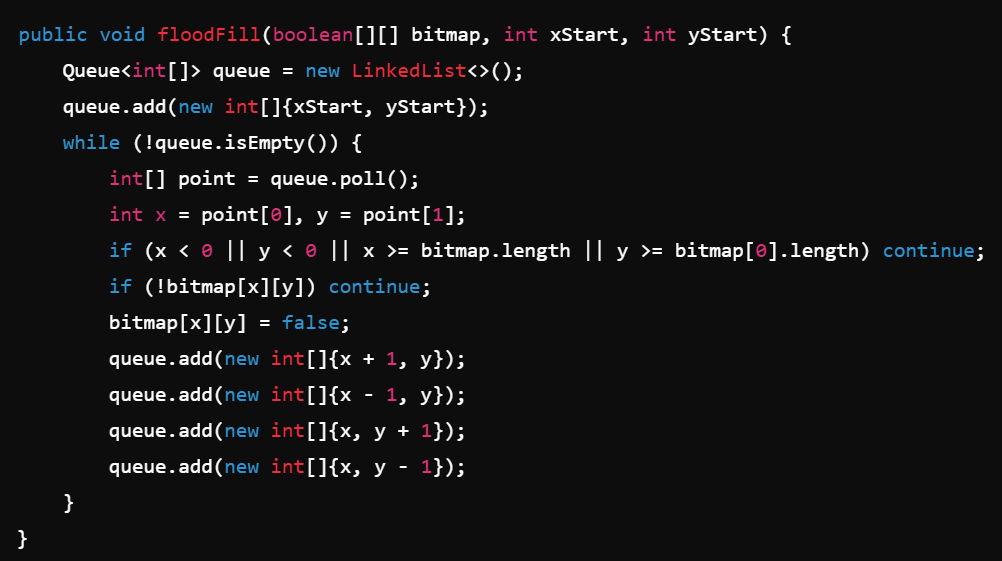
This version uses a queue to manage the exploration, avoiding stack overflow. Additionally, we worked with the candidate to ensure the boundary conditions and visited checks were properly implemented.
Step 4: Walking Through an Example
To help the candidate confidently explain their solution, we walked them through the following example:
Input Bitmap:
1 1 1 1
1 0 0 1
1 1 0 1
1 1 1 1
Start Position: (1, 1)
Goal: Replace all connected 0
s with 1
.
Execution Walkthrough:
- Begin at
(1, 1)
. Fill the pixel and add its neighbors(0, 1)
,(2, 1)
,(1, 0)
,(1, 2)
to the queue. - Move to
(1, 2)
. Fill the pixel and add its neighbors(0, 2)
,(2, 2)
,(1, 1)
,(1, 3)
to the queue. - Repeat until no more
0
pixels are reachable.
Final Output:
1 1 1 1
1 1 1 1
1 1 1 1
1 1 1 1
By breaking the problem into steps, the candidate was able to confidently explain the iterative process and how it avoids common pitfalls.
Step 5: Complexity Analysis
We worked with the candidate to analyze their solution:
- Time Complexity:
O(m * n)
, wherem
andn
are the dimensions of the bitmap. Each pixel is visited once. - Space Complexity:
O(m * n)
for the queue in the worst-case scenario.
Step 6: Preparing for Follow-Up Questions
We helped the candidate prepare for potential follow-up questions, such as:
- How would you handle multi-colored bitmaps?
- Extend the solution to support multiple colors by replacing only pixels matching the starting color.
- How can you reduce space complexity?
- Use in-place modifications to mark visited pixels, such as changing their value to a placeholder.
Final Interview Support
To ensure the candidate could confidently communicate their thought process, we reviewed the following:
- Algorithm Design: A clear explanation of how BFS works and why it was chosen.
- Edge Cases: Handling empty bitmaps, invalid start points, and boundary conditions.
- Follow-Up Questions: Strategies for scaling the solution and adapting it for new requirements.
Conclusion
Through our guidance, the candidate transformed a basic recursive solution into a robust, optimized BFS implementation. By focusing on clear communication, edge case handling, and performance analysis, the candidate was well-prepared to ace this question.
If you're preparing for interviews and need help breaking down challenging problems or refining your solutions, CSOAHelp is here to guide you every step of the way!
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
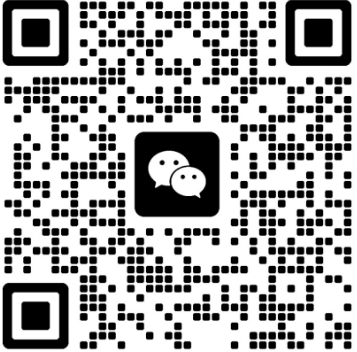