Question 1: Log Server
Problem Statement
You are tasked with building a log server to handle a large number of logs. Each log consists of a logId
and a timestamp
. Due to storage constraints, the server can only keep logs received in the past hour and store up to m
logs.
You need to implement the following functions:
- recordLog(logId, timestamp):
- Records a log entry with a given
logId
andtimestamp
. - Logs may arrive out of order.
- Duplicate
logId
s are allowed.
- Records a log entry with a given
- getLogs():
- Returns the
logId
s of all logs received in the past hour in order of theirtimestamp
.
- Returns the
- getLogCount():
- Returns the number of logs received in the past hour.
Constraints
- 1 ≤ m ≤ 1000
- 1 ≤ q ≤ 1,000,000
- timestamp: integer representing seconds.
Input Format for Custom Testing
Sample Input
100
10
RECORD 1 0
RECORD 2 300
GET_LOGS
COUNT
RECORD 3 1200
RECORD 1 1800
GET_LOGS
GET_LOG_COUNT
Sample Output
1, 2
2
1, 2, 3, 1
4
Explanation:
RECORD 1 0
: Log withlogId
1 is recorded attimestamp
0.RECORD 2 300
: Log withlogId
2 is recorded attimestamp
300.GET_LOGS
: Returns thelogId
s[1, 2]
in order of their timestamps.COUNT
: Returns the count of logs received in the past hour, which is2
.RECORD 3 1200
: Log withlogId
3 is recorded attimestamp
1200.RECORD 1 1800
: Another log withlogId
1 is recorded attimestamp
1800.GET_LOGS
: Returns[1, 2, 3, 1]
(logs recorded within the past hour).GET_LOG_COUNT
: Returns4
(total logs recorded in the past hour).
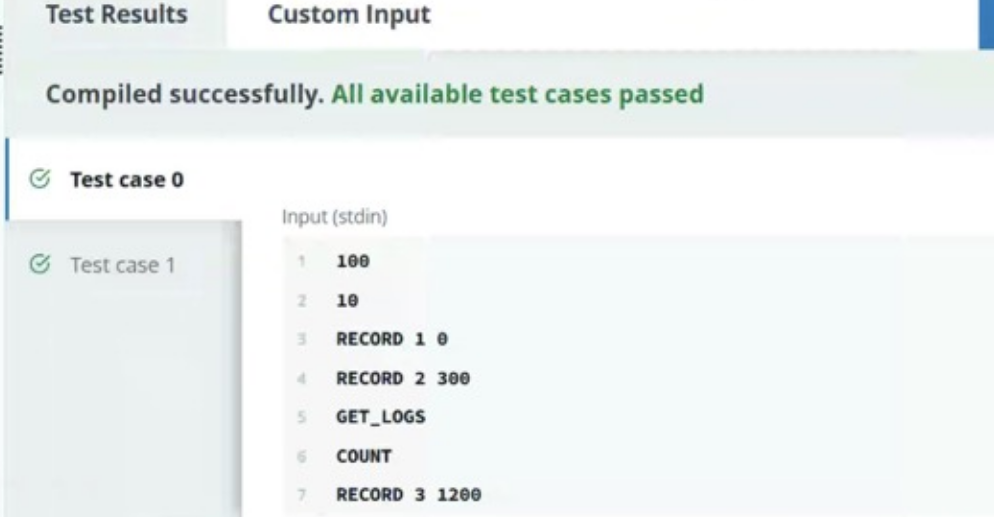
Question 2: Lion Competition
Problem Statement
As a lion trainer, you are participating in an international lion exhibition. Lions enter and exit the showroom at specific times, and judges tend to award the largest lions the highest scores. You want to estimate your chances of winning by identifying the largest lions present in the showroom at any given time.
You need to implement the following:
- LionCompetition constructor:
- Takes
lions
(list of lion descriptions) andschedule
(list of schedules for entry and exit times).
- Takes
- lionEntered(currentTime, height):
- Called when a lion enters the room at
currentTime
with a specificheight
.
- Called when a lion enters the room at
- lionLeft(currentTime, height):
- Called when a lion exits the room at
currentTime
with a specificheight
.
- Called when a lion exits the room at
- getBiggestLions():
- Returns a sorted list of lions in the room with heights greater than or equal to the largest competing lion.
Constraints
- Lion names are unique.
- Times are integers.
- Lions follow a strictly defined schedule for entry and exit.
Input Format for Custom Testing
Sample Input
definition marry 300
definition rob 250
schedule marry 10 15
schedule rob 13 20
start
8 enter 200
10 enter 310
10 enter 300
11 inspect
13 enter 250
13 inspect
15 exit 300
16 inspect
20 exit 250
21 end
Sample Output
2 marry rob
1 rob
Explanation:
- At time
10
, two lions (marry and rob) enter with heights 310 and 300, respectively. Both are considered the largest at this point. - At time
13
, another lion enters with height 250. Now, onlyrob
is the largest at this time.
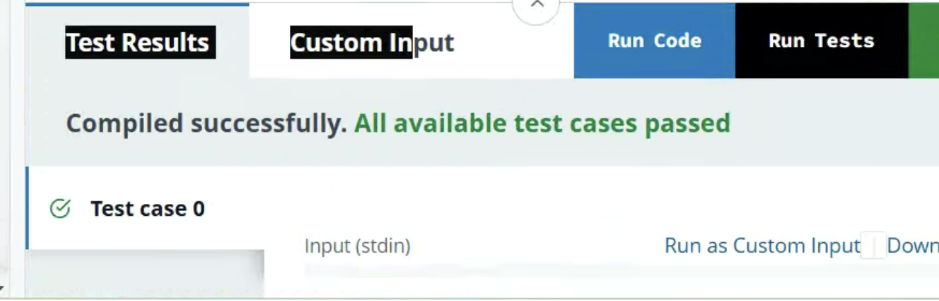
CSOAhelp长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
CSOAhelp consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
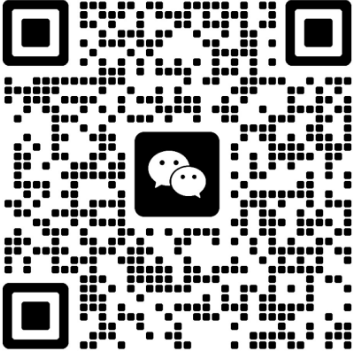