Introduction
In a TikTok system design interview, the candidate was tasked with designing an efficient Key-Value Store system. The challenge focused on designing high-performance operations and optimizing time complexity. With the assistance of csoahelp, the candidate successfully navigated the problem and provided a robust solution.
Problem Description
Task: Design an in-memory key-value store to handle integers (keys
as strings, values
as integers) and a global version number. No persistent storage is required.
Requirements:
- PUT
<key> <value>
- Assigns a
value
to the givenkey
. - Increments a global version number, assigning it to the write operation.
- Output format:
PUT(#<version_number>) <key> = <value>
.
- Assigns a
- GET
<key>
- Retrieves the most recent value of a
key
. - Output format:
GET <key> = <value>
orGET <key> = <NULL>
if the key is not set.
- Retrieves the most recent value of a
- GET
<key> <version_number>
- Retrieves the value of a
key
at a specific version. - Output format:
GET <key>(#<version_number>) = <value>
or<NULL>
if the version is invalid.
- Retrieves the value of a
Input Example:
PUT key1 5
PUT key2 6
GET key1
GET key1 1
GET key2 2
PUT key1 7
GET key1 3
GET key4
GET key1 4
Output Example:
PUT(#1) key1 = 5
PUT(#2) key2 = 6
GET key1 = 5
GET key1(#1) = 5
GET key2(#2) = 6
PUT(#3) key1 = 7
GET key1(#3) = 7
GET key4 = <NULL>
GET key1(#4) = 7
Interview Walkthrough
Clarifications
At the start of the interview, the candidate sought clarification on the problem:
- Is the key always a string, and the value always an integer?
- The interviewer confirmed that
key
is a string andvalue
is an integer.
- The interviewer confirmed that
- Does the global version expire?
- The global version number does not expire and is incremented with each write operation.
- Is the version specific to each key, or global across all keys?
- The version is global across all keys.
Once these details were clarified, the candidate, with our guidance, finalized their approach to the problem.
Solution Approach
Data Structure Design
The candidate, guided by us, used a HashMap as the primary storage structure:
- Structure:
HashMap<String, List<Tuple>>
- Key: The string
key
. - Value: A list of tuples, where each tuple is
(version, value)
.
- Key: The string
Algorithm Design
- PUT
<key> <value>
- If the
key
does not exist, initialize an empty list for it. - Append a tuple
(global_version, value)
to the list. - Increment the
global_version
after every write. - Time Complexity:
O(1)
.
- If the
- GET
<key>
- Retrieve the most recent value of the
key
. - Return
<NULL>
if thekey
does not exist. - Time Complexity:
O(1)
.
- Retrieve the most recent value of the
- GET
<key> <version_number>
- Use binary search to find the tuple with the highest version number ≤ the requested version.
- Time Complexity:
O(log(n))
, wheren
is the number of versions for the givenkey
.
Implementation Steps
Step 1: PUT Operation
- The candidate implemented the following steps:
- Check if the
key
exists in theHashMap
. If not, initialize an empty list. - Append the new
(version, value)
tuple to the list. - Increment the
global_version
and print the operation result.
- Check if the
Step 2: GET Operation
- Standard GET: Retrieve the last value in the list. If the
key
does not exist, return<NULL>
. - Versioned GET: Use binary search to find the closest version ≤ the requested version. If no valid version is found, return
<NULL>
.
Step 3: Optimization and Testing
- We guided the candidate to test edge cases:
- Requests for non-existent keys or versions.
- Performance under high numbers of versions.
- Validating the binary search logic for
GET
with a version number.
Test Cases
Example 1: Basic Operations
- Input:
PUT key1 5 PUT key2 6 GET key1 GET key1 1 PUT key1 7 GET key1 3 GET key1 2 GET key4 GET key1 4
- Output:
PUT(#1) key1 = 5 PUT(#2) key2 = 6 GET key1 = 5 GET key1(#1) = 5 GET key2(#2) = 6 PUT(#3) key1 = 7 GET key1(#3) = 7 GET key1(#2) = 5 GET key4 = <NULL> GET key1(#4) = 7
Example 2: Edge Cases
- Requesting a non-existent key:
- Input:
GET key3
- Output:
GET key3 = <NULL>
.
- Input:
- Requesting a version that exceeds the current global version:
- Input:
GET key1 10
- Output:
GET key1(#10) = 7
.
- Input:
Insights and Feedback
This problem emphasized system design and algorithm optimization. By leveraging HashMaps and binary search, the candidate efficiently addressed the requirements, ensuring optimal performance.
With csoahelp’s guidance, the candidate not only completed the task but also confidently handled edge cases and performance considerations.
If you are preparing for challenging technical interviews like this one, let csoahelp guide you to success!
Contact csoahelp today and make your technical interviews a breeze!
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
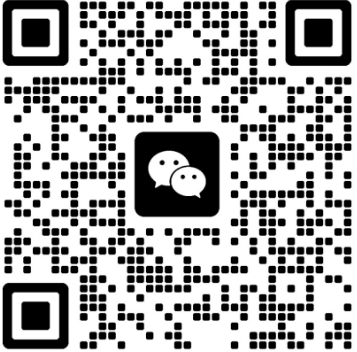