Introduction
This article delves into three critical Amazon interview problems, focusing on algorithm design, data structure implementation, and optimization techniques. CSOAHelp supported the candidate throughout these interviews by clarifying problems, enhancing solution strategies, and summarizing key technical details. Below, we analyze each question and the approach taken to solve them during the interview.
Problem 1: Most Frequently Used Cache
Problem Statement:
Design a data structure to manage a cache, implementing the following functionalities:
Cache(int capacity)
: Initialize the cache with a positive integer capacity.int get(int key)
: Return the value of the key if it exists; otherwise, return-1
.void put(int key, int value)
: Insert or update the key-value pair in the cache. If the cache reaches its capacity, remove the most frequently used item before inserting the new one.
Solution Approach:
- Caching Strategy:
- Use a Most Frequently Used (MFU) policy.
- Key Data Structures:
- HashMap: To store key-value pairs and their access frequency.
- Priority Queue: To dynamically track and sort items based on their frequency.
- Implementation Steps:
- For
put
: Check the cache size, evict the most frequently accessed key if full, then insert the new key-value pair. - For
get
: Fetch the value and update its frequency to reflect the access.
- For
- Complexity Analysis:
- Insert and delete operations:
O(log n)
. - Access operations:
O(1)
.
- Insert and delete operations:
Interview Insights:
- The candidate initially sought clarification on the difference between MFU and LRU (Least Recently Used) strategies.
- By constructing an example-driven explanation, the candidate demonstrated the logic for both insertion and eviction in an MFU cache.
Problem 2: Minimum Garbage Baskets
Problem Statement:
A city needs to calculate the minimum number of garbage baskets required, where each basket has a fixed size k
. The goal is to distribute all garbage sizes in the fewest number of baskets.
Solution Approach:
- Core Algorithm:
- Use a greedy strategy to pack garbage items into baskets, prioritizing larger items first.
- Implementation Steps:
- Sort the garbage sizes in descending order.
- Allocate each item to an existing basket if it fits; otherwise, create a new basket.
- Complexity Analysis:
- Sorting:
O(n log n)
. - Allocating items:
O(n)
.
- Sorting:
Interview Insights:
- The candidate explored alternative solutions, including using a priority queue to manage basket capacity dynamically.
- When asked to validate the optimality of the approach, the candidate reasoned that minimizing leftover capacity ensures fewer baskets.
Problem 3: Lowest Common Ancestor (LCA)
Problem Statement:
Given a binary tree where each node has a pointer to its parent, implement a function to find the lowest common ancestor (LCA) of two given nodes.
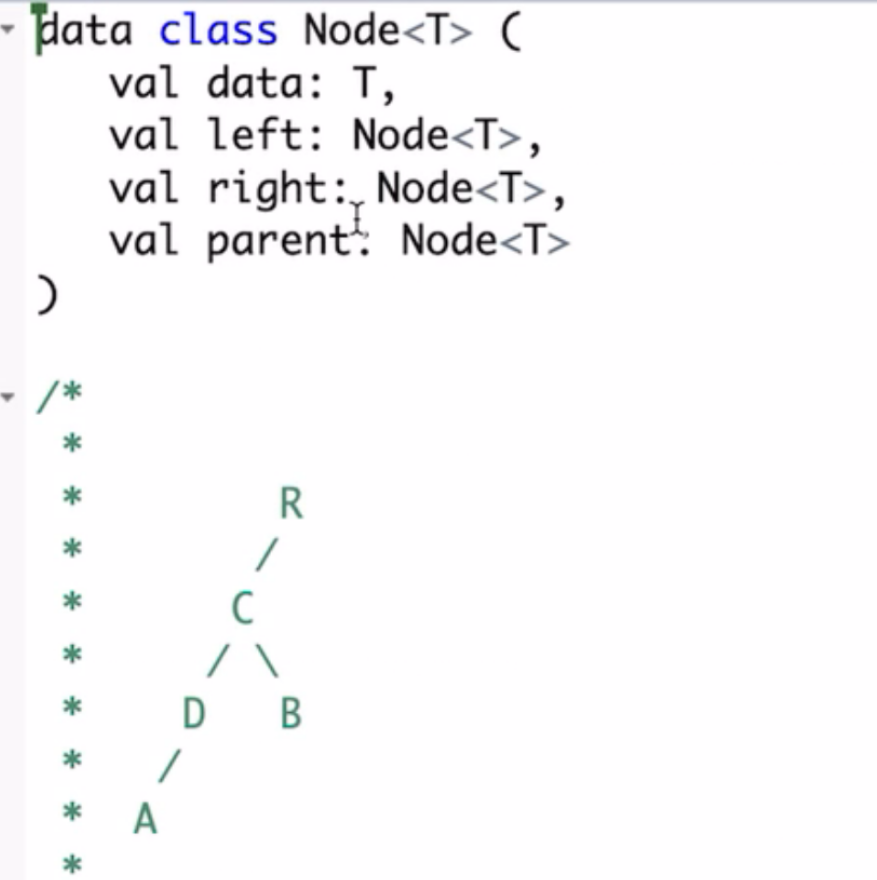
Solution Approach:
- Method 1: Path Intersection.
- Trace the path from each node to the root, storing visited nodes in a set. The first common node encountered is the LCA.
- Method 2: Pointer Movement.
- Use two pointers starting at the given nodes and move them upwards, switching to the other node’s path when reaching the root. They converge at the LCA.
- Complexity Analysis:
- Time Complexity:
O(h)
, whereh
is the tree height. - Space Complexity:
O(h)
for storing paths (in Method 1).
- Time Complexity:
Interview Insights:
- The candidate effectively explained the difference between the two methods, highlighting the reduced space complexity of Method 2.
- The interviewer encouraged discussion on edge cases, such as handling unbalanced trees and null nodes.
CSOAHelp's Role in the Interview Process
- Problem Clarification: During the interviews, CSOAHelp guided the candidate in understanding ambiguous requirements and edge cases.
- Strategy Refinement: The team provided optimized algorithmic approaches tailored to each problem.
- Mock Interviews: By simulating real interview scenarios, CSOAHelp ensured the candidate could articulate their solutions confidently and systematically.
Conclusion
These three Amazon interview problems represent the complexity and depth expected in technical interviews. With CSOAHelp’s expert assistance, the candidate successfully navigated each challenge, demonstrating strong problem-solving skills and strategic thinking. This case highlights the value of structured preparation and professional guidance in technical interviews.
Keywords: Amazon Interview Questions, Most Frequently Used Cache, Garbage Bin Optimization, Lowest Common Ancestor, Algorithm Design, Technical Interview Preparation.
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
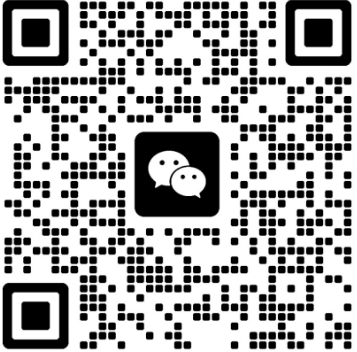