限时115分钟,有5个选择题和2道算法题,算法题如下
6. Job Execution
Problem Description
There are n jobs that can be executed in parallel on a processor, where the execution time of the ith job is executionTime[i]. To speed up execution, the following strategy is used:
- In one operation, a job is chosen (the major job) and is executed for x seconds. All other jobs are executed for y seconds, where y < x.
- A job is complete when it has been executed for at least executionTime[i] seconds, then it exits the pool.
Find the minimum number of operations in which the processor can completely execute all the jobs if run optimally.
Example
Consider n = 5, executionTime = [3, 4, 1, 7, 6], x = 4, y = 2.
The following strategy is optimal using 1-based indexing:
- Choose job 4 as the major job and reduce the execution times of job 4 by x = 4 and other jobs by y = 2.
Now executionTime = [1, 2, -1, 3, 4]. Job 3 is complete, so it is removed. - Choose job 4, executionTime = [-1, 0, -1, -1, 2]. Jobs 1, 2, and 4 are now complete.
- Choose job 5, executionTime = [-1, -1, -1, -1, -2]. Job 5 is complete.
It takes 3 operations to execute all the jobs, so the answer is 3.
Function Description
Complete the function getMinimumOperations in the editor below.
getMinimumOperations has the following parameters:
- int executionTime[n]: the execution times of each job
- int x: the time for which the major job is executed
- int y: the time for which all other jobs are executed
Returns
- int: the minimum number of operations in which the processor can complete the jobs
Constraints
- 1 ≤ n ≤ 100,000
- 1 ≤ executionTime[i] ≤ 10^9
- 1 ≤ y < x ≤ 10^9
Input Format for Custom Testing
Sample Case 0
Sample Input for Custom Testing
STDIN | FUNCTION |
---|---|
5 | → executionTime[] size n = 5 |
3 4 1 7 6 | → executionTime = [3, 4, 1, 7, 6] |
4 | → x = 4 |
2 | → y = 2 |
Sample Output
3
7. Equalize The Arrays
Make a source array equal to a target array using some given operations.
More formally, given an array source of length n, either add 1 to any prefix of the array or add 1 to any suffix of the array. That is, in one operation choose some index i and add 1 to source[0], source[1], ..., source[i] or add 1 to source[i], source[i + 1], ..., source[n - 1].
Find the minimum number of operations required to make the arrays equal. If it is impossible to make the two arrays equal, report -1 as the answer.
Example
For example, n = 3, source = [1, 2, 2], target = [2, 2, 3]. We can make the two arrays equal in two operations:
- Choose i = 0 and add 1 to the prefix:
source = [1, 2, 2] → [2, 2, 2] - Choose i = 2 and add 1 to the suffix:
source = [2, 2, 2] → [2, 2, 3] = target
Hence, the answer is 2 and it can be shown that it cannot be less than 2.
Function Description
Complete the function getMinOperations in the editor below.
getMinOperations has the following parameters:
- source[n]: an array of integers
- target[n]: an array of integers
Input Format for Custom Testing
Sample Case 0
Sample Input for Custom Testing
STDIN | FUNCTION |
---|---|
5 | → source[] size n = 5 |
1 2 3 -1 0 | → source = [1, 2, 3, -1, 0] |
3 | → target[] size n = 5 |
3 4 3 0 4 | → target = [3, 4, 3, 0, 4] |
Sample Output
6
Explanation
Given n = 5, source = [1, 2, 3, -1, 0], target = [3, 4, 3, 0, 4]:
- Choose i = 1 and add 1 to the prefix, and perform this operation two times.
source = [3, 4, 3, -1, 0], target = [3, 4, 3, 0, 4] - Choose i = 3 and add 1 to the suffix.
source = [3, 4, 3, 0, 1], target = [3, 4, 3, 0, 4] - Choose i = 4 and add 1 to the suffix, and perform this operation three times.
source = [3, 4, 3, 0, 4], target = [3, 4, 3, 0, 4]
The answer is 2 + 1 + 3 = 6.
Sample Case 1
Sample Input for Custom Testing
STDIN | FUNCTION |
---|---|
4 | → source[] size n = 4 |
1 2 3 0 | → source = [1, 2, 3, 0] |
1 | → target[] size n = 4 |
1 3 3 0 | → target = [1, 3, 3, 0] |
Sample Output
-1
Explanation
Given n = 4, source = [1, 2, 3, 0], target = [1, 3, 3, 0]:
There is no sequence of operations to make the array source equal to the target.
CSOAhelp长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
CSOAhelp consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
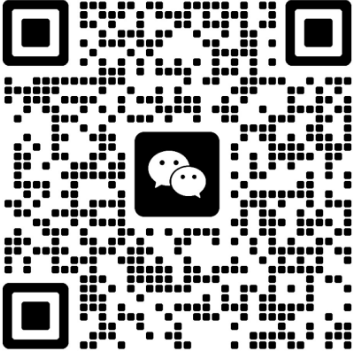