Problem Statement: Merge K Sorted Lists
We're trying to build an information retrieval system for bot prompts.
Information matches can exist in multiple data sources where-in each data source responds back to your service with a sorted list of matches. We now need functionality to take these individual sorted lists and output a single sorted list that merges all of them.
Input: List<List<Integer>> sortedLists
Output: List<Integer>
Example:
Input: [[1,4,5], [1,3,4], [2,6]]
Output: [1,1,2,3,4,4,5,6]
Input and Output Explanation
Input Format:
List<List<Integer>> sortedLists
Field Description:
- The input is a list of multiple sorted integer lists.
Output Format:
List<Integer>
Field Description:
- The output is a single sorted integer list, containing all the integers from the input lists merged in ascending order.
Input Example:
Input: [[1,4,5], [1,3,4], [2,6]]
Output Example:
Output: [1,1,2,3,4,4,5,6]
Problem Analysis and Approach
This is a classic K-way merge problem, where the goal is to merge multiple sorted lists into one sorted list. Various methods can be used, such as sequential merging, divide-and-conquer merging, or using a priority queue. Below, we focus on the priority queue method, which is efficient and optimal for this type of problem.
Solution: Priority Queue (Min-Heap)
Concept: We use a priority queue (min-heap) to keep track of the smallest elements from all the lists. This ensures that we always append the smallest available element to the result list, maintaining the sorted order.
Steps:
- Initialize the Priority Queue:
- Add the first element from each list (if the list is not empty) to the priority queue. Each entry in the queue stores the value, the list index, and the element's index within the list.
- Merge Process:
- Repeatedly extract the smallest element from the priority queue and append it to the result list.
- If the extracted element has a next element in its list, add that element to the priority queue.
- Termination:
- When the priority queue is empty, the merging process is complete.
Algorithm Analysis
Time Complexity:
- Queue Initialization: Adding the first element of each list to the queue takes
O(k log k)
, wherek
is the number of lists. - Merge Process: Extracting and adding elements to the priority queue takes
O(n log k)
in total, wheren
is the total number of elements across all lists. - Overall Complexity:
O(n log k)
.
Space Complexity:
- Priority Queue: The queue can hold up to
k
elements at any given time, so the space complexity isO(k)
. - Result List: Storing the final merged list takes
O(n)
space. - Overall Complexity:
O(n + k)
.
Example Walkthrough
Input:
[[1,4,5], [1,3,4], [2,6]]
Step-by-Step Process:
- Initialize the priority queue with the first element of each list:
Priority Queue: [(1,0,0), (1,1,0), (2,2,0)] // Format: (value, list index, element index)
- Extract the smallest element
1
(from list 0) and append it to the result list. Add the next element4
(from list 0) to the queue:Result: [1] Priority Queue: [(1,1,0), (2,2,0), (4,0,1)]
- Extract the smallest element
1
(from list 1) and append it to the result list. Add the next element3
(from list 1) to the queue:Result: [1,1] Priority Queue: [(2,2,0), (4,0,1), (3,1,1)]
- Continue extracting the smallest element and adding the next element from the respective list. Repeat this process until the priority queue is empty:
Result: [1,1,2,3,4,4,5,6]
Final Output:
[1,1,2,3,4,4,5,6]
Key Considerations
- Empty Lists:
- If a list is empty, simply skip it during the queue initialization.
- Edge Cases:
- If all lists are empty, return an empty list.
- If there is only one list, return it as-is.
- Stability:
- Since we use a min-heap, the merging process is stable, maintaining the order of elements with the same value.
CSOAHelp's Role in Candidate Success
In this Amazon interview scenario, CSOAHelp provided the candidate with the following critical support:
- Guidance on Problem Understanding:
- Helped the candidate clearly define the problem and recognize it as a K-way merge problem.
- Advised on using a priority queue for optimal performance.
- Optimization Advice:
- Explained the time and space complexity trade-offs of different approaches, ensuring the candidate selected the most efficient solution.
- Debugging Assistance:
- Supported the candidate in debugging implementation issues, such as priority queue handling and index management.
Conclusion
This Amazon interview question highlights a candidate's ability to handle multi-list merging efficiently using advanced data structures like priority queues. With CSOAHelp's expert guidance, the candidate successfully implemented the solution, optimized for both time and space complexity.
Need Help with Your Interviews?
If you're preparing for technical interviews, let CSOAHelp guide you to success. Our comprehensive support services ensure you're ready to tackle any challenge and secure your dream job. Contact us today!
如果您也想在面试中脱颖而出,欢迎联系我们。CSOAHelp 提供全面的面试辅导与代面服务,帮助您成功拿到梦寐以求的 Offer!
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
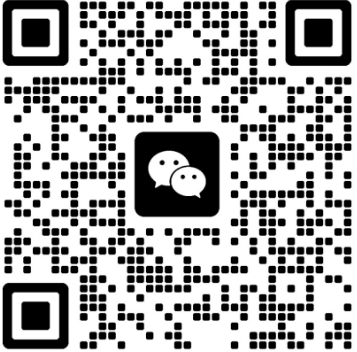