Problem Description
Question:
We have two sorted arrays of integers, A
and B
:
- Array
A
has empty slots at the end, exactly as many as the number of elements inB
. - The goal is to merge all elements from
B
intoA
, ensuring thatA
remains sorted.
Input:
A = [1, 2, 3, _, _, _, _]
B = [2, 4, 6, 100]
Output:
A = [1, 2, 2, 3, 4, 6, 100]
Requirements:
Optimize for speed and memory usage.
Problem Analysis and Solution Approach
This is a classic merging problem that tests efficient use of the two-pointer technique and in-place operations for memory optimization.
Solution Steps
1. Problem Constraints and Observations
- Array
A
has enough space to accommodate all elements ofB
, so no additional memory is needed. - The merge must be done in-place.
- Efficiency is crucial: avoid creating a new array or performing unnecessary comparisons.
2. Reverse Two-Pointer Approach
Instead of merging from the beginning of the arrays, start from their ends. This method avoids overwriting existing elements in A
.
Steps:
- Use two pointers:
i
: Points to the last non-empty element inA
.j
: Points to the last element inB
.k
: Points to the last position inA
(where the merged element will go).
- Compare elements pointed to by
i
andj
, placing the larger one atk
. - Move the respective pointer (
i
orj
) backward and decrementk
. - Repeat until all elements from
B
are merged intoA
.
Time and Space Complexity
Time Complexity:
Each element is visited and moved once, resulting in a time complexity of O(m + n)
, where m
and n
are the lengths of arrays A
and B
.
Space Complexity:
Since the merge is performed in-place, no additional memory is used, resulting in a space complexity of O(1)
.
Example Walkthrough
Input:
A = [1, 2, 3, _, _, _, _]
B = [2, 4, 6, 100]
Process:
- Initialize pointers:
i = 2
(last element inA
before empty slots),j = 3
(last element inB
),k = 6
(last position inA
).
- Compare and merge:
- Compare
A[i] = 3
andB[j] = 100
. Place100
atA[k]
. Updatej = 2
,k = 5
. - Compare
A[i] = 3
andB[j] = 6
. Place6
atA[k]
. Updatej = 1
,k = 4
. - Compare
A[i] = 3
andB[j] = 4
. Place4
atA[k]
. Updatej = 0
,k = 3
. - Compare
A[i] = 3
andB[j] = 2
. Place3
atA[k]
. Updatei = 1
,k = 2
. - Compare
A[i] = 2
andB[j] = 2
. Place2
atA[k]
. Updatej = -1
,k = 1
.
- Compare
- Result:
A = [1, 2, 2, 3, 4, 6, 100]
Key Considerations
- Edge Cases:
- If
B
is empty,A
remains unchanged. - If
A
is empty (except for empty slots), copy all elements fromB
.
- If
- Efficiency: This approach minimizes unnecessary comparisons and uses no extra memory, making it ideal for large datasets.
CSOAHelp’s Role in Candidate Success
In this interview scenario, CSOAHelp provided the candidate with real-time support, ensuring optimal performance under pressure. Here’s how we helped:
- Problem Breakdown:
- Guided the candidate to recognize the benefits of merging in reverse order to avoid overwriting data.
- Optimization Insights:
- Suggested the two-pointer approach for linear time complexity.
- Highlighted the importance of space efficiency in real-world applications.
- Edge Case Handling:
- Ensured the candidate covered scenarios like empty arrays and overlapping elements.
Keywords for Optimization
- Meta interview preparation
- Merge two sorted arrays in-place
- Optimized two-pointer techniques
- Efficient in-place algorithms
- Real-time interview support
Conclusion
Thanks to the rigorous preparation provided by CSOAHelp's Interview Coaching and VO Support, the candidate excelled in this challenging interview. They confidently tackled each question, earning the interviewer’s praise and securing a solid opportunity for their future career. For aspiring candidates, this demonstrates the value of structured preparation and expert guidance.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
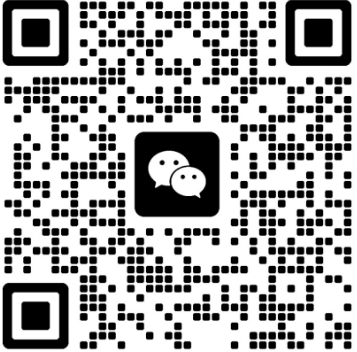