Problem Description
The developers of Hackerworld want to secure a database system consisting of n
data servers. Each server's vulnerability is represented by an array element server[i]
. An upgrade operation can reduce any server's vulnerability by 1.
The task is to determine the maximum possible value of the smallest vulnerability across all servers after exactly k
upgrade operations.
Recreating the MathWorks Interview
Step 1: Clarifying Requirements
Interviewer:
We’d like you to design an algorithm to optimize the server vulnerabilities. Specifically, after k
upgrades, we want to maximize the smallest vulnerability among the servers. Do you have any questions?
Candidate:
Yes, I have a few clarifications:
- Can the same server be upgraded multiple times?
- What should the algorithm do if
k
exceeds the total sum of all vulnerability values? - Is it possible for the output to be negative, especially when
k
is significantly large?
Interviewer:
- Yes, a single server can be upgraded multiple times.
- If
k
exceeds the sum of all vulnerabilities, you'll need to evenly distribute the remaining upgrades. - Yes, negative results are valid if
k
is large enough to reduce all vulnerabilities to zero and beyond.
Step 2: Candidate's Initial Approach
Candidate:
One possible approach is to use a priority queue (max-heap). Here's the basic idea:
- Add all server vulnerabilities to the max-heap.
- Repeatedly extract the maximum value, decrement it, and reinsert it into the heap until
k
operations are completed. - Finally, return the smallest value in the heap.
This approach has a time complexity of O(n + k * log n)
, where n
is the number of servers.
CSOAHelp Assistance:
Using a priority queue is a good start, but its complexity may not be optimal for larger k
values. Consider a frequency-based approach to reduce the complexity to O(n + k)
.
Step 3: Refined Frequency-Based Solution
Candidate:
To improve efficiency, I'll track the frequency of each vulnerability value. Here's the refined plan:
- Track Frequencies:
- Traverse the array and record the frequency of each value.
- Identify the current maximum vulnerability.
- Perform Upgrades:
- Start with the maximum value and decrement its frequency, distributing
k
until it’s exhausted. - If the frequency of the current maximum becomes zero, move to the next largest value.
- Start with the maximum value and decrement its frequency, distributing
- Handle Special Cases:
- If
k
exceeds the sum of all vulnerabilities, distribute the remainingk
evenly across all servers.
- If
Step 4: Complexity Analysis
Candidate:
Here’s the breakdown of the algorithm’s complexity:
- Frequency Tracking:
- Time complexity:
O(n)
to traverse the array and count frequencies.
- Time complexity:
- Upgrade Operations:
- Time complexity:
O(k)
to decrement values iteratively.
- Time complexity:
- Special Case Handling:
- Constant time:
O(1)
ifk
exceeds the total vulnerabilities.
- Constant time:
Total Complexity:O(n + k)
—this is efficient enough to handle large inputs.
Step 5: Real-Time Assistance and Edge Case Discussions
CSOAHelp Guidance:
While implementing the solution, ensure to:
- Efficiently update the maximum value during the upgrade process.
- Consider edge cases where all vulnerabilities are equal, and
k
is excessively large. In such cases, the result should account for distributed upgrades, potentially leading to a negative result.
Candidate:
If all servers have equal vulnerabilities and k
is significantly larger than the total sum, I’ll calculate the result using:result = -(k / n)
, where n
is the number of servers.
Final Interview Evaluation
Interviewer:
You’ve designed an efficient solution that balances performance and complexity. The frequency-based approach is particularly suitable for large-scale problems, and your edge case handling demonstrates a solid understanding of the problem. Excellent work!
Thanks to the rigorous preparation provided by CSOAHelp's Interview Coaching and VO Support, the candidate excelled in this challenging interview. They confidently tackled each question, earning the interviewer’s praise and securing a solid opportunity for their future career. For aspiring candidates, this demonstrates the value of structured preparation and expert guidance.
经过csoahelp的面试辅助,候选人获取了良好的面试表现。如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
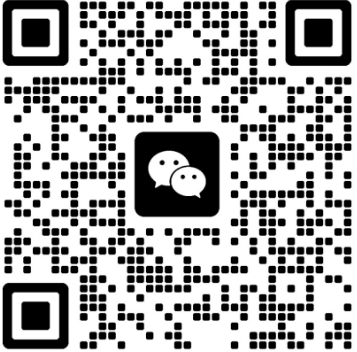