These questions are from a take-home test by Voleon, designed for software engineering candidates. The test consists of three questions to be completed in 120 minutes. It evaluates key technical skills including object-oriented design (OOD), data structures, algorithm design, and problem-solving under constraints.
- Question 1: Focuses on handling and processing market data streams efficiently using concepts like queues and dictionaries to simulate a broker's interaction with a stock exchange.
- Question 2: Tests the ability to design and implement logic for trading on behalf of a single client, considering constraints like participation rate and market conditions.
- Question 3: Builds on Question 2 by introducing multiple clients, requiring candidates to handle cascading effects and prioritize trades while maintaining fairness.
This test assesses coding efficiency, adherence to constraints, and understanding of complex system behaviors.
Question 1
Throughout this problem, you are a broker interfacing with the Berkeley Exchange. Your program will read events from stdin
and emit output to stdout
.
Part 1
In Part 1, you are tasked with tracking market activity on the Exchange. Your program will read printed trades as they come in and, when requested, print market activity data for a particular security. More details below.
Input (stdin
)
The input is composed of a stream of data coming in from the Berkeley Exchange, with two data types:
print
: A record of a trade on the exchange.volume-check
: A computation request for your program.
Each trade print is on a separate line. Each line contains the following space-delimited fields:
- The string
print
. timestamp
(int): The time at which the trade was executed, denoted as seconds since market open. For example, an 8-hour trading day would run from0
to28800
.security
(string): The name of the security traded.quantity
(positive integer): The number of shares traded.price
(positive integer): The price at which the trade was made.
Each volume-check
will contain:
- The string
volume-check
. timestamp
(int): The time at which the volume-check command was issued, denoted as seconds since market open.security
(string): The name of the security for which to calculate.
Example Input
print 12 SILVER 100 12
print 29 PEARL 25 99
print 37 SILVER 10 14
volume-check 49 SILVER
print 61 PEARL 25 100
Notes
- Times are guaranteed to be non-decreasing.
- String values are unquoted.
- All numerical values fit in standard 32-bit primitive types.
Question 2
Part 2: Trading for a Client
In this next part, you are also tasked with trading orders on behalf of one client. The rules for volume checks and general guidelines remain as described in the previous problem statement. You are encouraged to use your solution to the previous part as a starting point for this task.
Orders
Orders have the following space-delimited fields:
- The string
"order"
. - Timestamp (int): The time at which the order is issued.
- Security (string): The name of the desired security to trade.
- Client (string): The client's name.
- Goal Shares (positive integer): The number of shares the client would like to trade.
- Participation Rate (decimal): A 2-digit decimal in (0.00, 1.00) naming what percentage of this security's trailing-minute market trading volume this client is willing to take for this order. More specifics are under "Notes on Participation Rate."
Details
- The price of each new trade you execute is the previous trade price for that security.
- Always trade as soon as possible. That is, fill all feasible trades for any active orders before moving on to the next input item.
Output Format
For each order, output a sequence of trade prints to fulfill the order. A trade print is formatted as follows:
print {timestamp} {security} {num_shares} {price}
Example Input
print 122 GOLD 100 10
print 123 GOLD 100 10
order 123 GOLD BOB 100 0.20
print 124 GOLD 100 10
Correct Output
print 123 GOLD 40 10
print 124 GOLD 20 10
Explanation
When the order is initially processed, 40 shares are tradable for BOB because:
market_volume * p_rate = 200 * 0.20 = 40
This is the maximum integer amount of shares within BOB's participation rate threshold of 0.20. It's also less than BOB's goal of 100 shares. Trading 41 shares would violate the participation rate.
Question 3: Trading for Multiple Clients
Overview
In this next part, you are now tasked with trading orders on behalf of many clients. All rules stated in Parts 1 and 2 remain in effect. You are encouraged to use your solution to Part 2 as a starting point for this task.
Details
Your goal remains the same as Part 2: whenever an order is received, fulfill as many shares as possible in the order (up to goal_shares
), constrained by the participation rate.
Operate under these new multi-client definitions:
- No one client will ever have two active orders simultaneously for the same security. However, across all your clients, you may have multiple active orders for a security.
- Orders are prioritized based on the order in which they were received (seniority first). This rule applies across clients.
- Each trade is executed on behalf of only one client. You may not trade between your own clients. In other words, one trade cannot change the positions of multiple clients.
Output Format
For each order, output a sequence of trade prints in order to fulfill the order. As in Part 1, a trade print is formatted as follows:
print {timestamp} {security} {num_shares} {price}
Notes on Multi-Client Trading
Participation rate includes all market volume except that executed by the order itself:
participation_rate = volume_traded(0, S, T-59, T) / volume_traded(Exchange - O, S, T-59, T)
When multiple clients have active orders:
- Each client’s trade affects the overall market volume observed by other orders.
- One client's trade may enable other clients to trade more shares. You need to carefully handle such cascading effects in your implementation.
Example
Input:
print 122 GOLD 100 10
order 122 GOLD BOB 200 0.50
order 124 GOLD ALICE 100 0.20
Correct Output:
print 122 GOLD 50 10
print 124 GOLD 30 10
print 124 GOLD 15 10
print 124 GOLD 3 10
print 124 GOLD 1 10
Explanation:
- First, BOB trades 50 shares because 100 shares have been traded on the open market:复制代码
50 / 100 <= 0.5
- Next, ALICE trades 30 shares because:复制代码
30 / 150 <= 0.2
- BOB trades again for 15 shares as the new market volume grows. This follows cascading effects until all orders are fulfilled under their respective constraints.
At the end of the process:
- BOB trades a total of 166 shares.
- ALICE trades a total of 66 shares.
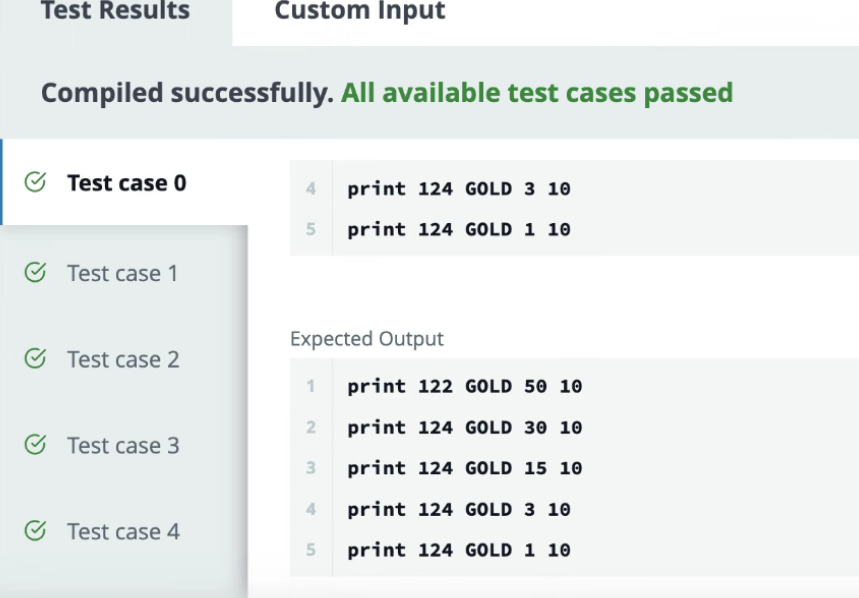
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
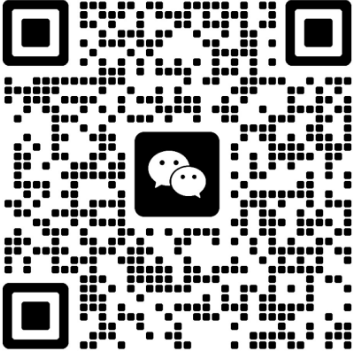