Test duration: 110 mins
Number of questions: 7
1. Implementing a Real-Time Operating System (RTOS)
TikTok is developing an embedded system that requires a real-time operating system (RTOS) to ensure timely task execution.
Which RTOS feature is most critical for ensuring real-time performance?
Pick ONE option
- (•) Preemptive multitasking
- ( ) Virtual memory
- ( ) File system support
- ( ) Multi-user support
2. Call Center Queue Optimization (Circular Queue)
You are implementing a call center software where customer calls are processed in the order they are received. The call center uses a circular queue to optimize memory usage. Implement the enqueue operation in a circular queue.
Which of the following pseudocode correctly implements the enqueue operation for a circular queue?
Function initializeQueue(size):
maxSize = size
# Initialize Max Sizefront = 0
# Initialize front pointerrear = 0
# Initialize rear pointer
Pick ONE option
- ( )
Function enqueue(call, queue, rear, maxSize): If (rear % maxSize) == front: Return "Queue is Full" Else: queue[rear] = call; rear = (rear + 1) % maxSize
- ( )
Function enqueue(call, queue, rear, maxSize): If (rear + 1) % maxSize == front: Return "Queue is Full" Else: queue[rear + 1] = call; rear = (rear + 1) % maxSize
- (•)
Function enqueue(call, queue, rear, maxSize): If (rear + 1) % maxSize == front: Return "Queue is Full" Else: queue[rear] = call; rear = (rear + 1) % maxSize
- ( )
Function enqueue(call, queue, rear, maxSize): If (rear - 1) % maxSize == front: Return "Queue is Full" Else: queue[rear] = call; rear = (rear + 1) % maxSize
3. File Compression (Huffman Encoding)
Your company is developing a file compression tool using Huffman encoding. You need to implement the function that builds the Huffman tree based on character frequencies.
Which of the following pseudocode correctly builds the Huffman tree?
Pick ONE option
- ( )
Function buildHuffmanTree(frequencies): Create a priority queue Q For each char in frequencies: Insert char into Q with frequency as priority While Q.size == 1: left = Q.extractMin() right = Q.extractMin() newNode = Merge left and right Insert newNode into Q Return Q.extractMin()
- ( )
Function buildHuffmanTree(frequencies): Create a priority queue Q For each char in frequencies: Insert char into Q with frequency as priority While Q.size > 1: left = Q.extractMin() right = Q.extractMin() newNode = Merge left and right Insert newNode into frequencies Return Q.extractMin()
- (•)
Function buildHuffmanTree(frequencies): Create a priority queue Q For each char in frequencies: Insert char into Q with frequency as priority While Q.size > 1: left = Q.extractMin() right = Q.extractMin() newNode = Merge left and right Insert newNode into Q Return Q.extractMin()
- ( )
Function buildHuffmanTree(frequencies): Create a priority queue Q For each char in frequencies: Insert char into Q with frequency as priority While Q.size > 1: left = Q.extractMin() right = Q.extractMin() Insert left and right into Q Return Q.extractMin()
4. Object Lifecycle and Resource Management in a Banking System
In a banking application, you are tasked with implementing a BankAccount
class that manages customer accounts, including account opening and closing. The BankAccount
class must handle external resources like file logging for transactions.
Which of the following OOP design ensures that resources are properly cleaned up after use, even when exceptions occur?
Pick ONE option
- ( ) Use of constructors to open resources and System.exit() in finally blocks to close them
- ( ) Use of a destructor (
__del__()
in Python orfinalize()
in Java) to close resources when the object is garbage collected - (•) Implementing the
AutoCloseable
(Java) or__enter__ / __exit__
(Python) pattern for resource management - ( ) Relying on the JVM or Python interpreter’s garbage collector to automatically close all resources
5. Encapsulation and Access Control in an E-Commerce Platform
You are developing a class Order
for an e-commerce platform. This class handles customer orders, including sensitive information like payment details.
Which of the following best demonstrates proper encapsulation to protect sensitive data?
Pick ONE option
- ( ) Make all attributes of the Order class public so that external classes can modify them directly
- ( ) Use protected attributes and provide public getter and setter methods for all attributes
- (•) Declare sensitive attributes like paymentDetails as private and provide only necessary getter methods, without setters
- ( ) Define all methods as private to ensure no other class can access the functionality
Question 6: Counting TikTok Creator Communities
In TikTok's ecosystem, creators often form communities and collaborate with them. These relationships can be represented by a matrix related
, where each cell related[i][j]
contains a binary value ("0" or "1"). A "1" in related[i][j]
means that Creator i and Creator j are directly connected, while a "0" means they are not connected.
Creators can also be connected through indirect connections. For example, if Creator A is connected to Creator B and Creator B is connected to Creator C, then Creator A is indirectly connected to Creator C.
All creators that are connected, either directly or indirectly, form a distinct community. No two creators from different communities are connected to each other, either directly or indirectly.
Your task is to return the number of distinct creator communities based on the given relationship matrix related
.
Example
Consider the following relationship matrix related
among TikTok creators:
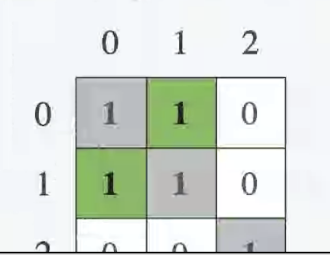
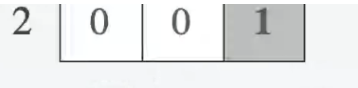
- Creators 0 and 1 are directly connected.
- Creator 2 is not connected to any other creator.
As a result, there are 2 distinct communities.
Question 7: TikTok User
A TikTok developer is creating a method to give numbers to users, shown as an array arr
.
The arrangement of numbers is called "good" if the difference between any two numbers next to each other is 1 or less. For example, the array [1, 2, 1]
is good, but [2, 1, 3]
is not, because the difference between 1 and 3 is 2, which is greater than 1.
The array arr
has n
numbers, but some numbers are missing. These missing numbers are shown as arr[i] = 0
. The goal is to find how many ways you can replace the missing numbers with any arbitrary integer numbers so that the final array is "good."
Since the number of ways can be very large, return the answer as the remainder when divided by 109+710^9 + 7109+7.
Note: The array arr
initially contains elements in the range -10^9 <= arr[i] <= 10^9
. However, the indices with missing values (arr[i] = 0
) can be replaced with any arbitrary integer to make the final array "good."
Example:
n = 3
arr = [0, 0, 1]
There are 9 ways to replace the 0s to make the array good:
arr = [0, 0, 1]
arr = [1, 0, 1]
arr = [-1, 0, 1]
arr = [0, 1, 1]
arr = [1, 1, 1]
arr = [2, 1, 1]
arr = [1, 2, 1]
arr = [2, 2, 1]
arr = [3, 2, 1]
In all of these arrays, the difference between each pair of numbers next to each other is 1 or less, so they are considered good.
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
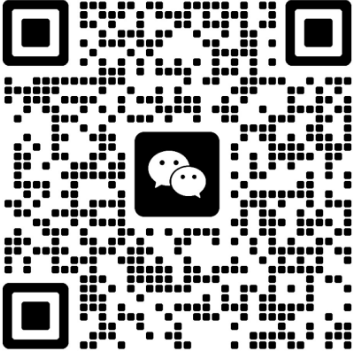