题目原文
This section has 8 questions. For each question, read the code and type your answer under the Answer heading.
You should answer the questions without running the code or consulting external resources to determine the output.
You have 15 minutes to complete this section.
Question A1
Assume you have a list of names, each entry has a first and last name. Write a list comprehension to generate the permutations of names, excluding the last names that are longer than 7 characters.
For example, names = ["John Smith", "Sally Anderson", "Ashwin Long", "Mandy Kim"]
,
returns'John Smith', 'John Long', 'John Kim', 'Sally Smith', 'Sally Long', 'Sally Kim', 'Ashwin Smith', 'Ashwin Long', 'Ashwin Kim', 'Mandy Smith', 'Mandy Long', 'Mandy Kim'
Your answer should be a single line of code. Please assume that there is an existing variable called names
containing a list of input names.
Answer:
中文翻译
问题 A1
假设你有一个名字列表,每个条目包含一个名字和一个姓氏。请编写一个列表推导式来生成姓名的排列组合,但需要排除那些姓氏长度超过 7 个字符的组合。
例如,names = ["John Smith", "Sally Anderson", "Ashwin Long", "Mandy Kim"]
,应返回'John Smith', 'John Long', 'John Kim', 'Sally Smith', 'Sally Long', 'Sally Kim', 'Ashwin Smith', 'Ashwin Long', 'Ashwin Kim', 'Mandy Smith', 'Mandy Long', 'Mandy Kim'
你的答案应为一行代码。假设有一个名为 names
的变量,包含输入的姓名列表。
面试过程记录
在候选人阅读题目后,首先仔细思考了题目中包含的信息。候选人注意到,题目要求生成名字和姓氏的所有组合,同时排除掉那些姓氏长度超过 7 个字符的情况。候选人意识到这是一个排列组合的问题,但带有过滤条件,因此需要使用列表推导式来实现。
为了确保对题目的理解没有偏差,候选人向面试官澄清道:“是否所有组合都需要包含在结果中,除非该组合的姓氏长度超过 7 个字符?” 面试官表示肯定。于是候选人继续推测实现的思路。
候选人首先考虑使用 Python 的 split()
方法将名字和姓氏分开,这样可以单独处理每个名字和姓氏。他解释道:“通过将列表中的每个名字拆分成名字部分和姓氏部分,然后通过列表推导式进行组合,我们可以实现题目要求的排列组合。”
接着,候选人提出了初步的代码框架,但他并未立即给出最终答案,而是进一步分析了代码中可能的逻辑。他想到可以将名字列表分拆成两个部分:名字列表和姓氏列表,然后通过条件筛选符合要求的组合。
候选人对他的思路进行了详细解释。他说:“我们可以用 if
条件来检查姓氏长度是否符合要求,将长度超过 7 个字符的姓氏排除在组合之外。” 面试官对此思路表示认可,并鼓励他继续推进。
在构建了初步框架后,候选人很快意识到列表推导式的具体语法。他最终写出了一行代码,使用了嵌套的列表推导式来实现名字和符合条件的姓氏的组合。候选人解释说:“这样我们就可以用一行代码完成要求,并且可以确保生成的组合符合题目规定的长度限制。”
面试官进一步追问道:“这个实现的时间复杂度是多少?” 候选人分析了一下,表示时间复杂度为 O(n^2),因为每个名字会与符合条件的每个姓氏进行组合。面试官对此表示满意,并称赞了候选人对列表推导式的灵活运用。
最终,候选人提供了一行代码作为答案,完美解决了问题。面试官对他的逻辑清晰度和解决问题的效率表示认可。
Interview Process Recap
After carefully reading the question, the candidate first thought about the information given. They noticed that the task required generating all permutations of first and last names, excluding combinations where the last name is longer than 7 characters. Realizing it was a permutation problem with a filter condition, the candidate concluded that a list comprehension would be ideal for implementing this solution.
To confirm their understanding, the candidate asked the interviewer, "Should we include all combinations unless the last name is longer than 7 characters?" The interviewer confirmed this. The candidate then considered using Python's split()
method to separate first and last names, enabling them to handle each name part independently. "By splitting each name entry into the first and last parts, then using list comprehension for combinations, we can meet the problem requirements," they explained.
The candidate outlined a solution framework but took additional time to think through the logic. They planned to split the list of names into first-name and last-name components, then use a filter condition to meet the requirement.
The candidate further explained, "We can use an if
condition within the list comprehension to check the last name length and exclude those longer than 7 characters." The interviewer appreciated the thought process and encouraged them to proceed.
Once the initial framework was established, the candidate realized the specific syntax for the list comprehension. They then wrote a one-liner code solution using nested list comprehension to generate all first-name and last-name combinations that met the length requirement. They noted, "With this one-liner, we can ensure the combinations meet the length criteria specified by the question."
The interviewer asked a follow-up question: "What is the time complexity of this implementation?" After a brief analysis, the candidate responded that it was O(n^2), given that each first name would combine with every last name that met the criteria. The interviewer was satisfied with the answer and commended the candidate for their efficient use of list comprehension.
Ultimately, the candidate provided a one-liner solution that fulfilled the requirements. The interviewer praised the candidate's clear logical reasoning and efficient problem-solving skills.
Summary
This problem tested the candidate's familiarity with list comprehensions and their ability to filter and combine data according to specific criteria. The candidate demonstrated a logical and structured approach, reasoning through the problem to find an optimal solution while showcasing strong code optimization skills.
通过csoahelp的辅助,候选人几近完美的回答了以上问题。
如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
In this Google interview, I demonstrated my understanding of common algorithmic problems and my problem-solving abilities. Each problem presented different challenges, but all could be solved through logical algorithm strategies.
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
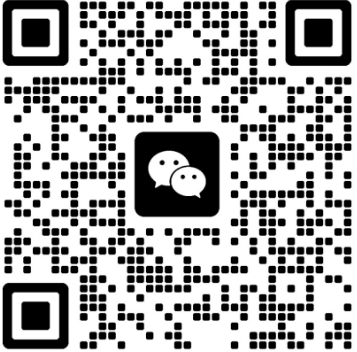