Problem Description
An Amazon pickup location has a set number of lockers in which boxes are dropped off and picked up. Boxes can come in many different sizes. Lockers come in a few pre-defined different sizes, with different heights, widths, and lengths like these:
- S: 2,2,2 (width, height, length)
- M: 4,4,4
- L: 8,8,8
Model the lockers, boxes, and pickup location, and implement:
- An algorithm for efficiently finding the best possible empty locker for a given box (i.e., the smallest empty locker that can fit the box).
- Methods to store a box in a locker within a pickup location.
- Methods to retrieve a box from a locker within a pickup location.
题目描述
在亚马逊的提货点,有若干个固定大小的储物柜,用于放置和提取包裹。包裹有多种尺寸,储物柜的尺寸也有预定义的不同规格,包括高度、宽度和长度,例如:
- 小号(S):2,2,2
- 中号(M):4,4,4
- 大号(L):8,8,8
要求对储物柜、包裹和提货点进行建模,并实现以下功能:
- 一个算法,用于高效地找到适合给定包裹的最佳空储物柜(即能容纳包裹的最小空储物柜)。
- 方法,将包裹存储到指定提货点的储物柜中。
- 方法,从提货点的储物柜中取出包裹。
候选人解题思路
在了解题目后,候选人首先花了一些时间思考如何有效建模,确保系统能够按照要求高效运行。他从面向对象的设计开始,提出了对Box
和Locker
的建模思路。
候选人:"我会将每个包裹和储物柜视作独立的对象,并且赋予它们独特的属性,例如宽度、高度和长度。这样可以保证每个储物柜和包裹都能独立管理自己的尺寸信息,有利于后续的尺寸匹配操作。"
在创建对象的设计中,候选人详细解释了自己的思路。他认为,Box
类需要有三个属性来存储包裹的尺寸,而Locker
类同样需要存储这些尺寸,以便在进行尺寸比较时确保简洁和直接。
接下来,候选人讨论了存储包裹的规则。他提出,系统应该先寻找一个尽可能小的空储物柜,以节省空间。
候选人:"为了实现这一点,我会给每个储物柜划分不同的尺寸类别,比如小号、中号和大号。然后我会使用一种数据结构(如映射表)来分别存储不同尺寸的储物柜。这将使得我们在查找某一特定尺寸的空储物柜时可以更快地找到匹配的空间。"
寻找最佳储物柜的算法
在具体的查找逻辑上,候选人设计了一种逐步检查的机制。
候选人:"我的算法会先检查包裹的尺寸,判断它需要的最小储物柜类别。然后我会尝试在这个尺寸的储物柜中找到一个空的储物柜。如果没有可用的储物柜,我会依次检查更大的尺寸,直到找到一个适合的储物柜。"
他进一步解释到,这种方法能够确保每个包裹都被分配到一个最小适合的空间,而不会浪费更大储物柜的资源。此外,如果找到合适的储物柜,算法会将这个储物柜从空储物柜列表中移除,并加入已使用的储物柜列表,以便管理和跟踪当前使用的空间。
存储和提取包裹
候选人还设计了两个核心方法:一个用于将包裹存储到合适的储物柜中,另一个用于从指定的储物柜中提取包裹。
候选人:"存储包裹的方法会先调用查找最佳储物柜的算法,一旦找到合适的储物柜,就会将包裹放入其中,并标记该储物柜为已使用。而提取包裹的方法则相对简单,只需确认目标储物柜是否包含包裹,并返回包裹信息,随后将储物柜标记为空即可。"
异常情况处理
候选人还特别考虑了一些可能的异常情况。例如,如果没有任何可用的储物柜,系统应该返回适当的错误提示。另外,如果提取时指定的储物柜是空的,也需要给予用户明确的反馈,确保系统的易用性和鲁棒性。
候选人:"为了提升用户体验,我会确保系统在遇到异常情况时提供清晰的反馈信息。比如当储物柜已满时,系统应立即告知用户,让他们知道当前没有空位。同时在提取包裹时,如果储物柜已经是空的,我会返回一条提示信息,而不是简单地执行失败。"
总结
整个设计过程展现了候选人在面向对象设计、数据结构使用以及异常处理上的能力。通过合理的系统设计和分组管理,他有效地优化了储物柜的利用率,为系统的高效运行提供了保障。这种面向对象和系统化的思维方式不仅帮助他快速实现了核心功能,还增强了系统的可扩展性和可维护性。
如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
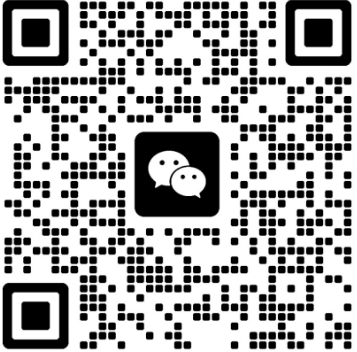
Candidate’s Solution Approach
After understanding the problem, the candidate began by thinking about an efficient way to model the system, ensuring it would meet the requirements for scalability and functionality. They decided to take an object-oriented approach, designing classes for both Box
and Locker
.
Candidate: "I’ll start by creating classes for each box and locker, giving each of them specific attributes like width, height, and length. This way, each box and locker can independently manage its dimensions, which will make it easier to match them later."
The candidate further explained their design, noting that the Box
class would hold the dimensions of each box, while the Locker
class would also store dimensions to enable quick comparisons.
Next, the candidate discussed the logic behind storing boxes. They pointed out that the system should try to find the smallest possible empty locker for each box to save space.
Candidate: "To do this, I’ll categorize lockers by size, such as Small, Medium, and Large, and use a data structure like a map to store the available lockers in each category. This will make it faster to locate an available locker when storing a box."
Algorithm for Finding the Best Locker
For the locker selection process, the candidate designed a step-by-step method to check lockers based on the box’s dimensions.
Candidate: "My algorithm will first determine the minimum locker size that can fit the box. Then, it will attempt to find an available locker in that category. If there are no available lockers in that size, it will move on to the next larger size until it finds a match."
They elaborated that this approach ensures that each box is stored in the smallest available locker, optimizing space usage. If a suitable locker is found, the algorithm will remove it from the list of empty lockers and add it to a list of used lockers to keep track of the occupied space.
Storing and Retrieving Boxes
The candidate also designed two core methods: one for storing a box in an appropriate locker and another for retrieving a box from a specified locker.
Candidate: "The method for storing a box will call the best locker search algorithm. Once it finds the right locker, it will store the box there and mark the locker as used. The retrieval method will simply check if the specified locker has a box, retrieve it if present, and mark the locker as empty."
Handling Edge Cases
The candidate carefully considered potential edge cases. For instance, they anticipated situations where no locker would be available and designed the system to return an appropriate error message. Similarly, if a locker was empty during a retrieval attempt, the system would notify the user.
Candidate: "To enhance user experience, I’ll make sure the system provides clear feedback when encountering errors. For example, if all lockers are occupied, the system should immediately notify the user. Similarly, if a locker is empty during a retrieval attempt, I’ll return a message rather than failing silently."
Summary
This problem allowed the candidate to demonstrate their object-oriented design skills, as well as their ability to work with data structures and handle edge cases. By thoughtfully organizing the lockers and implementing efficient searching and storage mechanisms, the candidate showcased their understanding of system optimization. This design not only ensures the core functionality but also enhances the maintainability and scalability of the system.
如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
In this Amazon interview, I demonstrated my understanding of common algorithmic problems and my problem-solving abilities. Each problem presented different challenges, but all could be solved through logical algorithm strategies.
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
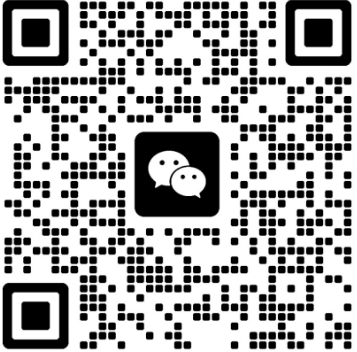