Problem Description (题目描述):
You are given a string s
containing just the characters (
, )
, {
, }
, [
and ]
. Your task is to determine if the input string is valid. An input string is valid if:
- Open brackets are closed by the same type of brackets.
- Open brackets are closed in the correct order.
题目描述 (Problem Description):
给定一个只包含字符 (
, )
, {
, }
, [
和 ]
的字符串 s
,判断输入的字符串是否有效。一个有效的字符串需满足以下条件:
- 左括号必须由相同类型的右括号闭合。
- 左括号必须以正确的顺序闭合。
Clarification Questions (澄清问题环节):
During the interview, the candidate first sought to clarify the problem constraints to ensure a complete understanding:
Candidate: "What are the parentheses we need to handle? Is it just ()
, []
, and {}
?"
Interviewer: "Yes, only those."
Candidate: "Can the string contain other characters that are not brackets?"
Interviewer: "No, the string will only contain the mentioned parentheses."
Candidate: "Is an empty string considered valid?"
Interviewer: "Yes, an empty string would be valid."
Through these clarifications, the candidate confirmed that the problem focuses strictly on the given set of parentheses and that an empty string is valid.
澄清问题环节 (Clarification Questions):
在面试过程中,候选人首先就问题的限制条件进行了澄清,以确保完整理解问题:
候选人: “我们需要处理的括号类型是哪些?仅仅是 ()
, []
和 {}
吗?”
面试官: “是的,只有这些类型。”
候选人: “字符串中是否会包含括号以外的其他字符?”
面试官: “不会,字符串只包含这些括号。”
候选人: “空字符串算作有效的吗?”
面试官: “是的,空字符串是有效的。”
通过这些问题的澄清,候选人确认了问题仅集中于指定的括号集,并且空字符串是有效的输入。
Solution Discussion (解题思路沟通环节):
The candidate proposed using a stack to solve this problem. Here's how they explained their approach:
Candidate: "We can solve this problem using a stack. The main idea is to push opening brackets onto the stack and pop them when we encounter a closing bracket. The last opened bracket must be the first to close."
Algorithm Explanation (算法解释):
- Initialize a stack to keep track of opening brackets.
- Iterate through the string character by character:
- If the character is an opening bracket (
(
,{
, or[
), push it onto the stack. - If it’s a closing bracket (
)
,}
, or]
), check if the stack is empty (which would indicate there's no corresponding opening bracket), or pop the top of the stack and check if it matches the correct type of bracket.
- If the character is an opening bracket (
- After iterating through the string, if the stack is empty, all brackets were matched, and the string is valid.
Edge Cases (边缘情况):
- An empty string is valid.
- A string starting with a closing bracket is automatically invalid since there’s no corresponding opening bracket.
- Unbalanced strings (like too many opening or closing brackets) are invalid.
Interviewer Follow-up (追问解答环节):
Interviewer: "What would happen if the string starts with a closing bracket?"
Candidate: "If the string starts with a closing bracket, the stack will either be empty, or the top of the stack won’t match the closing bracket, which will cause the function to return false."
Interviewer: "Good. What would be the time and space complexity of your solution?"
Candidate: "The time complexity is O(n), where n is the length of the string since we iterate over each character only once. The space complexity is also O(n) because, in the worst case, we might need to store all opening brackets in the stack."
解题思路沟通环节 (Solution Discussion):
候选人提出使用栈来解决该问题。以下是他对解决方案的解释:
候选人: “我们可以用一个栈来解决这个问题。主要思路是将左括号压入栈中,当遇到右括号时,检查栈是否为空,并弹出栈顶的左括号,确保它和当前的右括号匹配。”
算法解释 (Algorithm Explanation):
- 初始化一个栈,用来记录左括号。
- 遍历字符串的每个字符:
- 如果是左括号
(
,{
或[
,将其压入栈中。 - 如果是右括号
)
,}
或]
,检查栈是否为空(如果为空,表示没有对应的左括号),或者弹出栈顶元素,确保其类型与当前右括号匹配。
- 如果是左括号
- 遍历结束后,检查栈是否为空,若为空,则表示所有的括号都正确匹配,字符串有效。
边缘情况 (Edge Cases):
- 空字符串是有效的。
- 以右括号开头的字符串是无效的,因为没有对应的左括号。
- 括号不平衡的字符串(例如左括号或右括号多余)是无效的。
Complexity Summary (总结时空复杂度环节):
- Time complexity (时间复杂度): O(n), where n is the length of the string.
- Space complexity (空间复杂度): O(n), because the stack could store up to n/2 opening brackets in the worst case.
Behavioral Question (BQ问题对话):
After discussing the algorithm, the interviewer asked a behavioral question to understand the candidate's teamwork approach.
Interviewer: "Can you tell me about a time when you had to work with a team to solve a difficult problem?"
Candidate: "Sure, there was a project where we needed to integrate multiple services into a single platform. Each service had different APIs and data formats, which was challenging. My role was to lead the API integration, so I organized regular check-ins with team members to ensure everyone was on the same page. We faced several issues with data inconsistencies, but by working closely with the team, we were able to build a solution that standardized the data and ensured smooth integration. Communication was key in overcoming the challenges we faced."
追问解答环节 (Interviewer Follow-up):
面试官: “如果字符串以右括号开头会发生什么情况?”
候选人: “如果字符串以右括号开头,栈要么为空,要么栈顶的括号类型不会匹配,这将导致函数返回 false
。”
面试官: “很好。你认为这个解决方案的时间和空间复杂度是多少?”
候选人: “时间复杂度是 O(n),其中 n 是字符串的长度,因为我们只遍历每个字符一次。空间复杂度也是 O(n),因为在最坏情况下,我们可能需要在栈中存储所有的左括号。”
复杂度总结 (Complexity Summary):
- 时间复杂度: O(n),其中 n 是字符串的长度。
- 空间复杂度: O(n),因为栈中最多可能存储 n/2 个左括号。
行为问题对话 (Behavioral Question):
在讨论完算法之后,面试官询问了一道行为问题,目的是了解候选人如何与团队协作解决问题。
面试官: “你能谈谈你在团队中解决一个棘手问题的经历吗?”
候选人: “当然。有一次,我们的项目需要将多个服务整合到一个平台上。每个服务都有不同的 API 和数据格式,这非常具有挑战性。我负责 API 的整合部分,因此我组织了定期的团队会议,确保每个人都能保持同步。我们遇到了数据不一致的问题,但通过与团队的紧密合作,我们最终构建了一个标准化的数据解决方案,确保了顺利的整合。在这个过程中,良好的沟通起到了至关重要的作用。”
如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
In this Microsoft Technical Interview Blog: Valid Parentheses Problem - 微软技术面试博客:括号有效性问题 - 一亩三分地 - 微软面经 - 面试代面 - VO support interview, I demonstrated my understanding of common algorithmic problems and my problem-solving abilities. Each problem presented different challenges, but all could be solved through logical algorithm strategies.
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
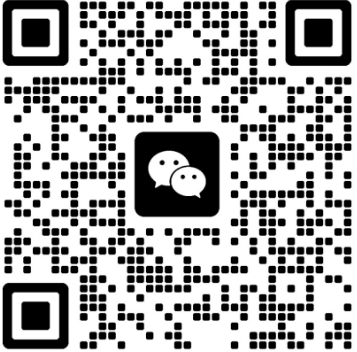