问题一:代码替换
问题描述(原文):
Question 1: Code Replacement
Problem Description (Original):
- Given an input
sourceCode = "num foo;"
, and a list ofreplacements = [{start: 0, before: "num", after: "String"}, {start: 4, before: "foo", after: "bar"}]
, replace the values in thesourceCode
with the given replacements, ensuring the result matches the expected output.
Example:
Input:
sourceCode = "num foo;"
replacements = [
{start: 0, before: "num", after: "String"},
{start: 4, before: "foo", after: "bar"}
]
Output:
"String bar;"
澄清问题环节
候选人:对于这道题,是否需要假设输入总是合法的?也就是说,给定的 before
确实会在 sourceCode
的 start
位置开始?
面试官:是的,输入数据是可信的。你可以假设 before
和 start
是正确的,不需要额外验证。
候选人:好的,那是否允许我们进行多次遍历或者重构 sourceCode
?比如我可以通过一次扫描或者构造新的字符串来解决这个问题吗?
面试官:没问题,可以自由选择实现方式。我们更关注的是你如何处理这种有序替换的问题。
(在这个环节,候选人确认了输入数据的边界条件,并进一步探讨了实现细节,确保自己的理解与面试官保持一致。)
Clarification Stage
Candidate: Should I assume the input is always valid? Meaning, the before
string will always start at the start
index in the sourceCode
?
Interviewer: Yes, you can assume the input data is correct. You don't need to handle any validation for the before
or start
positions.
Candidate: Alright, is it acceptable to make multiple passes over the sourceCode
, or can I restructure it in any way, such as by creating a new string?
Interviewer: Yes, feel free to choose any approach. We're more interested in how you handle the ordered replacements efficiently.
(In this stage, the candidate clarifies the edge cases of the problem, specifically about input validity, and confirms if restructuring the string is a valid approach. This ensures that their understanding aligns with the interviewer's expectations.)
解题思路沟通环节
候选人:这个问题本质上可以看作是一个有序的替换问题。首先,我需要处理每个 replacement
,根据 start
来找到字符串中的替换位置,验证 before
是否匹配,然后用 after
替换掉对应部分。
我有两种方案:
- 遍历整个
sourceCode
并进行原地替换:逐个处理每个替换,依次扫描,直接在字符串上进行修改。 - 构造新字符串:通过将每个替换结果插入新的字符串中,这样可以避免直接操作原始字符串,保证替换过程不会破坏后续替换的准确性。
面试官:听起来不错,你能说说这两种方法的时间和空间复杂度吗?
候选人:第一种方法的时间复杂度是 O(n)(n 是 sourceCode
的长度),但每次替换可能需要移动字符串的一部分,因此在最坏情况下复杂度可能更高。
第二种方法的时间复杂度理论上也是 O(n),但由于我们每次都将结果追加到新的字符串上,可以有效避免因原地替换导致的移位问题。此外,第二种方法的空间复杂度是 O(n),因为我们构建了一个新的字符串。
Algorithm Discussion Stage
Candidate: This problem can be viewed as an ordered replacement task. The main idea is to process each replacement
, find the position in the string using the start
, check if the before
string matches the expected substring, and then replace it with the after
string.
I can think of two approaches:
- Iterate over the
sourceCode
and perform in-place replacements: I can go through each replacement one by one, scanning the string and directly modifying it. - Build a new string: Instead of modifying the original string, I can construct a new one by processing each replacement and appending the result to this new string.
Interviewer: Sounds good, can you explain the time and space complexity of these two approaches?
Candidate: The first method has a time complexity of O(n), where n is the length of the sourceCode
. However, each replacement might involve shifting parts of the string, so in the worst case, the complexity could be higher.
The second method also has a time complexity of O(n) because we’re constructing the result incrementally without shifting elements. The space complexity is O(n) since we’re creating a new string.
追问解答环节
面试官:如果 replacements
中的元素不是按照 start
排序的,你的算法还能正常工作吗?你会如何处理这种情况?
候选人:如果 replacements
没有排序,那我会先对它们进行排序,按照 start
的位置从小到大排序,这样可以保证替换时不破坏后续的位置。排序的时间复杂度是 O(m log m),其中 m 是 replacements
的长度。整体时间复杂度仍然可以保持在 O(n + m log m)。
(面试官通过追问,考察了候选人对顺序替换问题的应对能力。候选人通过补充排序步骤,展示了应对无序替换时的处理方法。)
Follow-up Questions Stage
Interviewer: What if the elements in replacements
are not sorted by the start
position? Would your algorithm still work correctly? How would you handle this scenario?
Candidate: If the replacements
are not sorted, then I would first sort them based on the start
position in ascending order. This ensures that each replacement happens in the correct sequence without affecting the positions of subsequent replacements. Sorting would add a time complexity of O(m log m), where m is the length of replacements
. The overall time complexity would then be O(n + m log m).
(Here, the interviewer tests the candidate's ability to handle unsorted input. The candidate's solution involves sorting the replacements to maintain order, which shows their problem-solving flexibility.)
总结时空复杂度环节
面试官:很好,你能再总结一下这道题的时间和空间复杂度吗?
候选人:对于有序替换的情况,时间复杂度是 O(n),其中 n 是 sourceCode
的长度。空间复杂度也是 O(n),因为我们可能会构造一个新的字符串。如果 replacements
无序,则需要额外的 O(m log m) 时间来进行排序。
Time and Space Complexity Summary
Interviewer: Can you summarize the time and space complexity for this problem?
Candidate: For ordered replacements, the time complexity is O(n), where n is the length of the sourceCode
. The space complexity is also O(n) because we may construct a new string. If the replacements are unordered, the time complexity becomes O(n + m log m) due to sorting, where m is the number of replacement operations.
问题二:三元组问题
问题描述(原文):
Question 2: Tuple Matching Problem
Problem Description (Original):
- Given three arrays A, B, and C of the same length N and an integer D, find how many tuples (i, j, k) satisfy all of the following:
- |A[i] - B[j]| <= D
- |A[i] - C[k]| <= D
- |B[j] - C[k]| <= D
Additional Information:
- There may be duplicate integers in the arrays.
- The arrays are sorted.
澄清问题环节
候选人:我有几个问题想确认一下。首先,是否可以假设数组 A、B、C 都是已经排好序的?另外,数组中可能有重复的元素对吗?
面试官:是的,数组是排好序的,元素可以重复。
候选人:那么,D 是正数,并且不会对数组元素的绝对值范围产生影响,对吧?
面试官:是的,D 是一个正整数,不需要担心超出范围的情况。
(候选人通过澄清问题,确认了输入数据的特性,包括数组的有序性和重复元素的处理,这对后续解题思路的设计非常重要。)
Clarification Stage
Candidate: I have a couple of questions. First, can I assume the arrays A, B, and C are sorted? Also, are duplicates allowed in the arrays?
Interviewer: Yes, you can assume the arrays are sorted, and duplicates are allowed.
Candidate: Understood. Is D always positive, and we don't have to worry about array element ranges, correct?
Interviewer: That's correct, D is a positive integer, and you don’t need to worry about the range of values.
(In this stage, the candidate ensures they have all the necessary information about the problem, confirming the sorted property of the arrays and the allowance of duplicates. This understanding sets up the foundation for the candidate’s solution.)
解题思路沟通环节
候选人:既然数组是有序的,我们可以利用这一特性来减少不必要的比较操作。我考虑采用三指针法,即分别用三个指针遍历 A、B、C 三个数组,逐步寻找满足条件的三元组。
基本思路是:
- 初始化三个指针 i, j, k 分别指向 A, B, C 的第一个元素。
- 然后通过比较
|A[i] - B[j]|
、|A[i] - C[k]|
和|B[j] - C[k]|
的结果,来判断当前指针组合是否满足条件。如果满足,则记录下来。 - 每次移动指针时,尽量保持满足条件,优先移动那些差距较小的指针,避免不必要的遍历。
面试官:那你觉得这个方法的时间复杂度如何?
候选人:因为每个数组都只遍历一次,所以时间复杂度是 O(N),其中 N 是数组的长度。由于我们只需要常数空间来存储指针,所以空间复杂度是 O(1)。
Algorithm Discussion Stage
Candidate: Since the arrays are sorted, we can use this property to reduce unnecessary comparisons. I plan to use the three-pointer approach, which involves having three pointers, one for each array (i for A, j for B, k for C), and iterating through the arrays to find tuples that satisfy the conditions.
The general approach would be:
- Initialize three pointers i, j, and k at the start of A, B, and C respectively.
- For each combination of values at A[i], B[j], and C[k], I’ll check if they satisfy the conditions:
|A[i] - B[j]| <= D
,|A[i] - C[k]| <= D
, and|B[j] - C[k]| <= D
. - If they do, I’ll record the valid tuple. Based on the result of the comparisons, I’ll adjust the pointers to explore other valid combinations, prioritizing moving the pointer that minimizes the gap.
Interviewer: That sounds reasonable. What do you think the time complexity would be for this approach?
Candidate: Since we are only iterating through the arrays once, the time complexity would be O(N), where N is the length of the arrays. The space complexity is O(1) since we only use a constant amount of space to store the pointers.
追问解答环节
面试官:如果数组中有大量重复元素,这个方法会遇到什么问题吗?你会如何优化它?
候选人:如果有大量重复元素,可能会导致很多无效的比较操作。我可以在每次移动指针时,跳过重复的元素,这样可以减少重复计算。此外,由于数组是有序的,跳过重复元素不会影响最终结果。
面试官:很好,如果数组的长度 N 非常大,你认为这个算法还能有效运行吗?有没有什么优化空间?
候选人:对于非常大的数组,最关键的是减少不必要的遍历操作。我可以进一步优化指针的移动方式,比如当某个条件不满足时,快速跳过多个不可能的元素,而不是逐个移动指针。这可能需要更复杂的逻辑,但能够提升在大规模数据上的表现。
(通过这些追问,面试官测试了候选人对重复元素和大规模数据处理的思考。候选人提出了跳过重复元素和快速跳转的优化方案,展示了对效率的关注。)
Follow-up Questions Stage
Interviewer: What if there are many duplicate elements in the arrays? How would that affect your solution? Can you optimize it further?
Candidate: If there are duplicates, the algorithm could make unnecessary comparisons, which could slow things down. I can skip over the duplicate elements when moving the pointers, which would reduce redundant operations. Since the arrays are sorted, skipping duplicates is simple and won’t affect the correctness of the solution.
Interviewer: Good. If N is very large, do you think this approach will still perform well? Are there any further optimizations you’d consider?
Candidate: For very large arrays, the main concern is avoiding unnecessary comparisons. One potential optimization is to move the pointers faster when a condition is not met. For example, if |A[i] - B[j]| > D
, I can skip multiple elements at once if I know they won’t satisfy the condition, instead of moving one by one. This would require more complex logic but could improve performance for large datasets.
(In this stage, the interviewer explores edge cases and scalability concerns. The candidate responds with an approach to handle duplicates efficiently and mentions possible optimizations for large input sizes.)
总结时空复杂度环节
面试官:现在你能总结一下这个三指针方法的时间和空间复杂度吗?
候选人:这个方法的时间复杂度是 O(N),因为我们只需要遍历每个数组一次。空间复杂度是 O(1),因为除了存储指针之外,我们没有使用额外的空间。
Time and Space Complexity Summary
Interviewer: Can you summarize the time and space complexity for your three-pointer solution?
Candidate: The time complexity is O(N), where N is the length of the arrays, because we traverse each array once. The space complexity is O(1) since we are only using a few pointers and not any additional data structures.
行为问题环节(Behavioral Questions)
在技术问题结束后,面试官进入了行为问题环节,以了解候选人在团队中的表现和工作方式。
面试官:你能谈谈在过去的项目中,你是如何与团队成员合作解决复杂问题的吗?
候选人:有一次我们在一个项目中需要处理大量实时数据,挑战在于如何在不牺牲性能的前提下保持系统的稳定性。我和团队一起分析了系统的各个模块,确定了性能瓶颈,并制定了逐步优化的策略。我们保持了紧密的沟通,确保每个小的改进都有明确的指标验证,最终我们成功在规定时间内交付了项目。
(候选人通过具体项目的例子,展示了自己在团队合作和解决复杂问题方面的能力,强调了沟通和数据驱动的决策。)
Behavioral Questions Stage
After the technical questions, the interviewer transitions to behavioral questions to gauge the candidate's teamwork and communication skills.
Interviewer: Can you share a time when you worked with a team to solve a complex problem? How did you handle it?
Candidate: Sure, I worked on a project that involved processing a large volume of real-time data. The main challenge was balancing performance and stability. My team and I analyzed each module to identify performance bottlenecks and developed a plan to optimize them step by step. We communicated closely and validated each improvement with clear metrics. By focusing on incremental changes and maintaining a collaborative environment, we were able to deliver the project successfully and on time.
(Here, the candidate highlights their teamwork, problem-solving ability, and focus on data-driven decision-making, showcasing soft skills that are crucial in a collaborative work environment.)
通过这次csoahelp提供的面试辅助服务,候选人成功在此次面试中取得了良好的成绩。如果您也希望在面试过程中获得专业的指导和支持,请随时联系我们,我们将为您提供定制化的面试辅助服务,帮助您顺利通过面试。
With the interview assistance provided by CSOAHelp, the candidate successfully achieved excellent results in this interview. If you are also looking for professional guidance and support during your interview process, feel free to contact us. We offer customized interview assistance services to help you confidently tackle interview challenges and succeed.
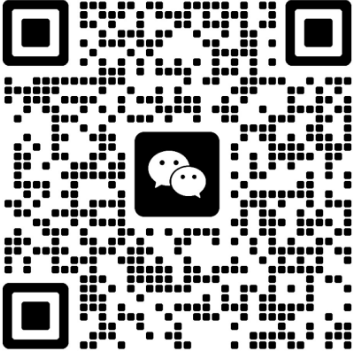