面试官:我们有一个二叉树,任务是返回它的按层遍历结果,也就是说,从上到下,逐层返回每个节点的值。然而,这次我们需要以“之”字形(zigzag)的顺序返回每一层的节点值。也就是说,一层从左到右,下一层从右到左,依次交替。
Given a binary tree, the task is to return its level order traversal, i.e., the values of the nodes from top to bottom, level by level. But this time, you need to return the nodes' values at each level in zigzag order (i.e., left to right, then right to left for the next level and alternate between).
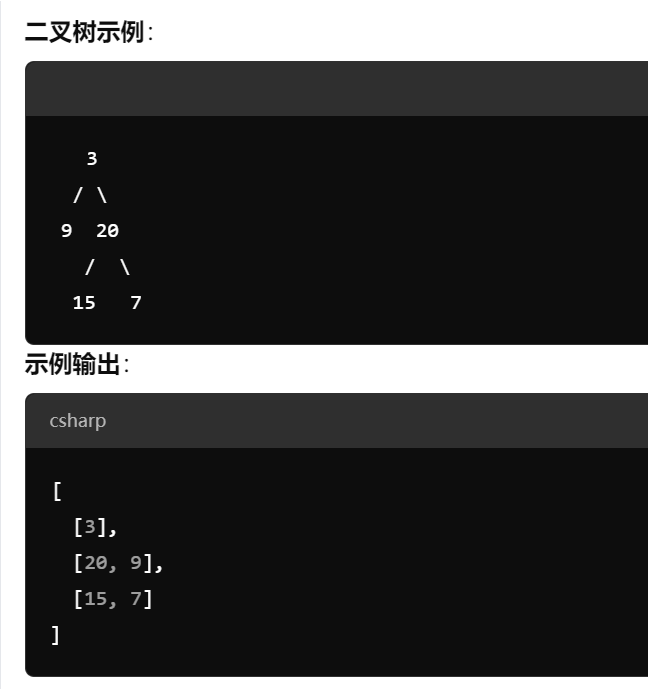
澄清问题环节
候选人:树可能是空的吗?如果是空树,输出结果是否应该是空数组?
面试官:是的,树可以为空。如果输入的是一棵空树,输出就是一个空数组。
(候选人在此通过一个简单的问题确认了输入数据的边界条件,这不仅帮助明确了输入的特殊情况处理,也展现了候选人关注细节的态度。)
Clarification Stage
Candidate: Can the tree be null? In that case, is the output simply an empty array?
Interviewer: Yes, the tree can be null. If the input is an empty tree, you should return an empty array.
(The candidate immediately seeks clarification on edge cases, which is a common interview practice. This not only helps clarify potential input but also shows attention to detail in handling special conditions.)
解题思路沟通环节
澄清问题结束后,候选人开始详细阐述自己的解题思路。他提出了多种解法,并依次分析了每种方法的可行性。
候选人:好的,我能想到几种不同的解决方法。第一种是通过队列进行广度优先遍历,并且我可以使用一个布尔标志位 flag
来控制当前层是否需要反转。
- 我们可以使用队列来实现广度优先搜索,每次处理完一层后切换
flag
的状态。如果flag
为true
,我们就直接将该层的节点顺序加入结果;如果flag
为false
,我们就反转该层的节点顺序再加入结果。- 时间复杂度:O(n),因为我们需要访问每个节点一次。
- 空间复杂度:O(n),因为队列需要保存所有节点的指针。
面试官:嗯,继续。
候选人:另外,我还可以使用双端队列(deque),它可以从两侧同时操作,效率会更高一些。在每一层根据 flag
的状态选择从哪一侧插入节点。这个方法和队列的实现类似,但是利用了双端队列的灵活性。
- 使用双端队列实现广度优先遍历,当
flag
为true
时,我们从左侧插入节点;当flag
为false
时,我们从右侧插入节点。- 时间复杂度:O(n),每个节点只会被访问一次。
- 空间复杂度:O(n),双端队列同样需要存储所有节点。
面试官:双端队列确实是个好主意。那么你觉得这两种方法的空间复杂度有区别吗?
候选人:其实空间复杂度都是 O(n),因为在最坏情况下,队列或双端队列中都可能存储整棵树的所有节点。不过,实际占用的空间取决于树的最大层数。也就是说,虽然总体复杂度是 O(n),但每一层的最大节点数决定了所需的空间。
面试官:很好,你有没有考虑过其他方法,比如修改树的结构来解决这个问题?
候选人:嗯,修改树结构的确是一个思路。如果我们可以在某些节点中存储额外的信息,或许能够减少某些操作的复杂度。不过我觉得就目前来看,使用双端队列的方法应该是最直接和高效的,我能最快实现这一解法。
面试官:那你打算从哪种方法入手?
候选人:我会优先选择使用双端队列的方案。
(在这一环节中,候选人详细阐述了两种主要解法,面试官通过追问帮助候选人进一步确认了最佳解决方案,同时候选人通过层层分析展示了自己对算法效率和实现难度的理解。)
追问解答环节
面试官:在这种广度优先遍历中,如何处理节点的顺序变化?特别是从左到右和从右到左的交替过程,你如何保证不出错?
候选人:这里的关键在于双端队列的使用。由于双端队列可以同时从两侧插入元素,当 flag
为 true
时,我们从队列的右侧插入节点值,也就是按照正常顺序处理;当 flag
为 false
时,我们从左侧插入节点值,相当于对这一层进行了反转。
面试官:听起来可行。你能再详细解释一下这一过程在 Python 中是如何实现的吗?
候选人:在 Python 中,我们可以直接使用 collections
模块中的 deque
,这个双端队列支持从左右两侧插入和弹出元素。对于每一层的节点,我们使用 deque
存储节点的值,根据 flag
决定从哪一侧插入。每处理完一层,切换 flag
,以确保下一个层次的节点顺序正确。
(通过这部分追问,面试官测试了候选人对实现细节的掌握情况。候选人通过对具体操作的详细描述展示了自己对数据结构和语言特性的熟悉度。)
Algorithm Discussion Stage
After clarifying the input constraints, the candidate proceeds to outline their approach. They present several potential solutions, discussing the pros and cons of each.
Candidate: Okay, I have a few solutions in mind. The first is a breadth-first search (BFS) using a queue and a boolean flag to determine whether to reverse the current level.
- We can perform a standard breadth-first search using a queue. After processing each level, we can toggle the
flag
to reverse the nodes in that level or not. If theflag
istrue
, we append the nodes in order; if it'sfalse
, we reverse the order before appending.- Time complexity: O(n) because we visit each node exactly once.
- Space complexity: O(n) because we need to store all the nodes in the queue.
Interviewer: Sounds good, keep going.
Candidate: Another approach is to use a deque. A deque (double-ended queue) allows us to insert and remove elements from both ends. We can use this to alternate the direction for each level based on the flag
. When the flag
is true
, we append nodes from the left side; when flag
is false
, we append from the right side.
- Using a deque, we can handle the zigzag traversal more efficiently. On each level, we can add nodes from one side based on the
flag
. If theflag
istrue
, we append nodes normally; if it'sfalse
, we append them in reverse order.- Time complexity: O(n), same as the queue-based solution, since we process each node once.
- Space complexity: O(n) as well, since we need to store all nodes.
Interviewer: The deque idea is interesting. Do you see any differences in space complexity between the two methods?
Candidate: Both solutions have a space complexity of O(n), as we need to store nodes in either the queue or deque. However, the actual space usage may vary depending on the number of nodes in the largest level of the tree. So while the complexity is technically O(n), the maximum space required depends on the number of nodes at the widest level of the tree.
Interviewer: That's correct. Have you thought about any methods where you modify the tree itself to solve the problem?
Candidate: Modifying the tree structure is an option. I could potentially store extra information at certain nodes to optimize traversal. However, I think the deque solution is the most straightforward and efficient way to solve the problem quickly.
Interviewer: So, which approach are you leaning towards?
Candidate: I'll go with the deque approach. It seems the most efficient and easy to implement for this problem.
(Here, the candidate clearly presents their thought process, considers multiple solutions, and communicates effectively with the interviewer. The discussion of space complexity reveals the candidate's deep understanding of how different approaches can impact memory usage, even within the same asymptotic complexity.)
总结时空复杂度环节
面试官:很好,那我们来总结一下这个算法的时间和空间复杂度吧。
候选人:从时间复杂度来看,我们每个节点只会访问一次,所以时间复杂度是 O(n),其中 n 是节点的总数。空间复杂度同样是 O(n),因为我们需要存储每一层的节点,最坏情况下可能是树中最大的一层节点数。
面试官:对的,空间复杂度可以理解为取决于最大层数的节点。
(在这个环节中,候选人再次确认了算法的时空复杂度,并且展示了对树结构特性的理解,进一步展现了全面的思维过程。)
行为问题环节(Behavioral Questions)
技术问题结束后,面试官进入了行为问题环节,通常这是面试的重要组成部分,考察候选人的沟通能力、团队合作以及解决问题的方式。
面试官:你能分享一个你曾经面对挑战的项目吗?你是如何解决这些挑战的?
候选人:有一次我参与了一个大规模的项目,项目的复杂度很高,需要在短时间内实现高效的数据处理系统。在这个项目中,我们面临的主要挑战是如何在性能和内存之间找到平衡。我的团队通过细致分析每个模块的时间消耗,找到了瓶颈并做出了相应的优化。我们采取了渐进式优化的策略,确保每个小的改进都能够有效提升系统的整体性能。最终我们成功提前交付了项目。
(通过这个例子,候选人展示了自己在面对复杂项目时的解决问题能力,并且强调了团队合作和技术决策的重要性。)
Follow-up Question Stage
Interviewer: How will you manage the node order changes during traversal? Specifically, how do you ensure the alternation between left-to-right and right-to-left levels is handled correctly?
Candidate: The key lies in the use of the deque. By leveraging its ability to insert from both ends, I can dynamically adjust how I process each level. When flag
is true
, I append the nodes from the left side of the deque; when flag
is false
, I insert nodes from the right. This allows me to toggle between left-to-right and right-to-left traversal seamlessly.
Interviewer: That makes sense. Could you explain how this works in Python, and how you’d implement the deque approach?
Candidate: In Python, we can use the collections.deque
data structure. It's a built-in deque that allows for efficient append and pop operations from both ends. For each level, I will store the node values in a deque. If the flag
is true
, I append the nodes from the left; if it's false
, I append them from the right. After processing each level, I toggle the flag
to ensure the next level is processed in the opposite order.
(This part of the conversation demonstrates the candidate's familiarity with Python's built-in data structures and their ability to explain the details of the implementation clearly.)
Time and Space Complexity Summary
Interviewer: Great, now let’s wrap up by summarizing the time and space complexity of your approach.
Candidate: The time complexity is O(n) because we visit each node exactly once. The space complexity is also O(n) because we need to store nodes in the deque, and in the worst case, we might have to store all nodes in the largest level of the tree. So both time and space complexity are O(n), where n is the total number of nodes in the tree.
Interviewer: Correct. Space complexity is largely dependent on the maximum number of nodes in any level.
(In this stage, the candidate concisely wraps up the complexity analysis, ensuring they have covered both time and space considerations in their explanation.)
Behavioral Questions Stage
After the technical part, the interviewer shifts focus to behavioral questions, which are equally important in many interviews to assess the candidate's soft skills and cultural fit.
Interviewer: Can you tell me about a time when you faced a challenging project? How did you handle it?
Candidate: Sure, I was part of a large-scale project where the goal was to build a high-performance data processing system. The main challenge was balancing between memory usage and speed, as the system had to handle a large volume of data in real time. My team and I carefully analyzed each module’s performance, identifying bottlenecks. We optimized the most critical sections of the system incrementally, making sure each change had a measurable impact. In the end, we were able to deliver the project ahead of schedule.
(Through this behavioral question, the candidate showcases their ability to manage challenges in a team setting, highlight problem-solving skills, and demonstrate a proactive approach to technical challenges.)
如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
In this Microsoft interview, I demonstrated my understanding of common algorithmic problems and my problem-solving abilities. Each problem presented different challenges, but all could be solved through logical algorithm strategies.
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
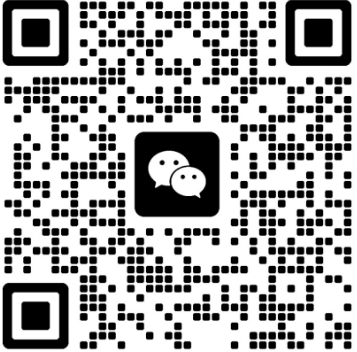