Hi there!
Google invites you to complete a coding exercise.
- 90 Minutes
- 2 Questions
At a glance:
- This timed coding exercise will give us a sense of your skills.
- The timer begins counting down as soon as you start the exercise.
- Practice first to familiarize yourself with the code editor and exercise format.
Question One
Problem:
Given an array A
of length N
, return an integer representing the minimum number of moves needed to transform a zero-filled array into A
.
Examples:
- Given
A = [2, 1, 3]
, the function should return 4.
For example, the following sequence of moves leads to the solution:[0, 0, 0] → [1, 1, 1] → [2, 1, 1] → [2, 1, 2] → [2, 1, 3]
. - Given
A = [2, 2, 0, 0, 1]
, the function should return 3.
The following sequence of moves leads to the solution:[0, 0, 0, 0, 0] → [1, 1, 0, 0, 0] → [2, 2, 0, 0, 0] → [2, 2, 0, 0, 1]
. - Given
A = [5, 4, 2, 4, 1]
, the function should return 7.
One possible optimal sequence of moves is:[0, 0, 0, 0, 0] → [1, 1, 1, 1, 1] → [2, 2, 2, 2, 1] → [3, 3, 2, 2, 1] → [4, 4, 2, 2, 1] → [5, 4, 2, 2, 1] → [5, 4, 2, 3, 1] → [5, 4, 2, 4, 1]
.
Write an efficient algorithm for the following assumptions:
N
is an integer within the range [1..100,000].- Each element of array
A
is an integer within the range [0..1,000,000,000]. - We guarantee that the answer will not exceed 1,000,000,000.
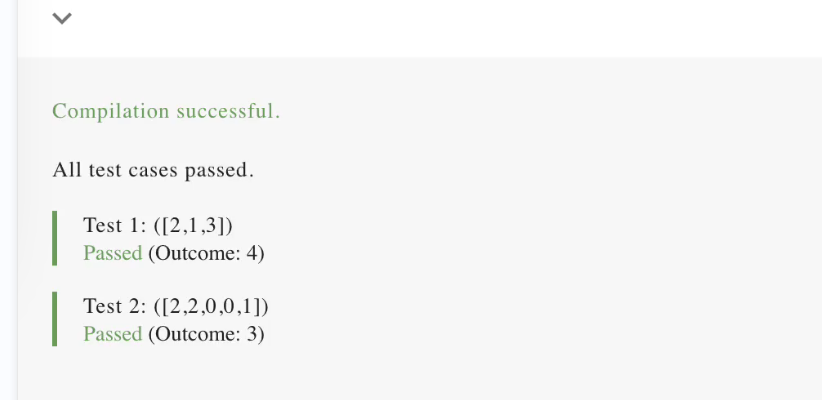
Question Two
Problem:
There is an array, named digits
, consisting of N
digits. Choose at most three digits (not necessarily adjacent) and merge them into a new integer without changing the order of the digits. What is the biggest number that can be obtained this way?
Write a function:def solution(digits)
That, given an array of N
digits, returns the biggest number that can be built.
Examples:
- Given
digits = [7, 2, 3, 3, 4, 9]
, the function should return 749. - Given
digits = [0, 0, 5, 7]
, the function should return 57.
Assume that:
N
is an integer within the range [3..50].- Each element of array, named
digits
, is an integer within the range [0..9].
In your solution, focus on correctness. The performance of your solution will not be the focus of the assessment.
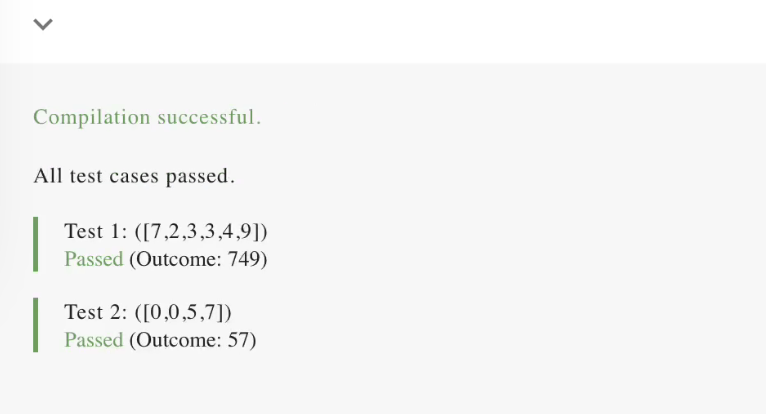
我们长期稳定承接各大科技公司如TikTok、Google、Amazon等的OA笔试代写服务,确保满分通过。如有需求,请随时联系我们。
We consistently provide professional online assessment services for major tech companies like TikTok, Google, and Amazon, guaranteeing perfect scores. Feel free to contact us if you're interested.
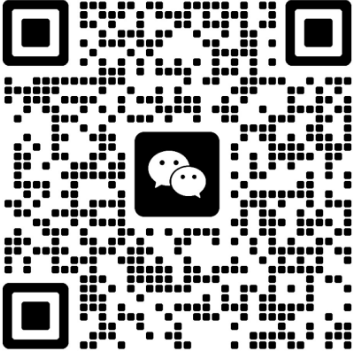