Introduction
Google技术面试中,算法题占据了非常重要的部分。本文将基于实际的多轮面试题目,详细描述每道题的解题思路,并还原候选人与面试官的沟通过程。这些问题不仅考察了候选人解决问题的能力,还考验了他们对算法复杂度的理解。
Problem 1: String Matching with Pattern and Block Configuration
Problem Statement (English):
string: ".###.##.#..."
pattern: "?###???????"
block_config = [3, 2, 1]
.
- empty space#
- occupied space?
- wildcard (matches any symbol)
Return the number of substrings that match the pattern and block configuration.
Input Example:
string = ".###.##.#..."
pattern = "?###???????"
block_config = [3, 2, 1]
面试过程:
在开始题目之前,面试官解释了题目的背景。候选人首先澄清了block_config的具体含义:“这里的数字是否表示连续的#
符号的个数?” 面试官确认了这个理解是正确的。
接着,候选人提出了使用滑动窗口的解法,通过遍历string
的子串,逐个与pattern
匹配。候选人通过两个计数器来统计#
符号的数量,以及?
符号如何灵活匹配.
和#
。在过程中,面试官进一步要求候选人优化算法,特别是要早期判断哪些子串无效,从而减少计算量。
Problem 2: Concatenate Strings with a Maximum Length
Problem Statement (English):
Concatenate a list of strings so that the resulting string doesn't exceed some given maximum length. This might require removing characters from the end of strings, but we need to preserve at least one character from each string.
Example:
input = [ "abcd", "1234" ]
max_length = 9
面试过程:
面试官给出这个题目时,候选人首先仔细分析了例子。候选人询问:“我们需要在保持顺序的情况下,如何处理超过最大长度的问题?” 面试官提醒要确保至少保留每个字符串的一个字符,并且要使用分隔符。
候选人随后采用了贪心算法来解决这个问题,通过从每个字符串的末尾删除字符直到满足最大长度,同时确保至少保留一个字符。面试官表示对这一思路的认同,并建议测试不同的边界情况,例如所有字符串的长度总和小于最大长度,或者字符串长度差异很大的情况。
Problem 3: Find the First Failing Test
Problem Statement (English):
To represent code changes, you are given an array of objects that have two class methods.
- `run_tests()` returns the result of running the tests on the object and returns a boolean.
- `get_name()` returns a string unique identifier of the object.
The result of `run_tests()` will be either `true` (tests passed) or `false` (tests failed). The first object will have passing tests, and the last will have failing tests. Going from the first object to the last, find and return the name of the first object that fails the tests.
面试过程:
在给出题目后,候选人理解了要从一组对象中找到第一个失败的测试对象。候选人通过二分查找法来优化查找过程。候选人提出:“能否通过并行化的方式减少测试时间?” 面试官对这个问题表示赞同,并引入了一个新的函数run_tests_parallel()
,该函数可以并行执行多个测试。
面试官要求候选人解释如何使用这个新函数来提升算法效率。候选人则通过分批测试的方式来减少总测试次数,最终实现了时间复杂度的显著优化。
Problem 4: Find Maximum Profit in Stock Trading with Transaction Fees
Problem Statement (English):
You are given an array `prices` where `prices[i]` is the price of a given stock on the `i`th day, and an integer `fee` representing a transaction fee. Find the maximum profit you can achieve. You may complete as many transactions as you like, but you need to pay the transaction fee for each transaction.
Example:
Input: prices = [1, 3, 2, 8, 4, 9], fee = 2
Output: 8
面试过程:
候选人采用了动态规划的解法,通过两个状态:hold
表示持有股票的最大利润,cash
表示未持有股票的最大利润。在解答过程中,候选人向面试官询问是否允许多次交易并支付手续费。 面试官确认了这一点。
候选人逐步构建了状态转移方程,并且通过动态规划优化了时间复杂度,最终达到了O(n)的线性时间解法。面试官对这一解法表示认可,并建议候选人进一步优化空间复杂度。
如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
In this Google interview, I demonstrated my understanding of common algorithmic problems and my problem-solving abilities. Each problem presented different challenges, but all could be solved through logical algorithm strategies.
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
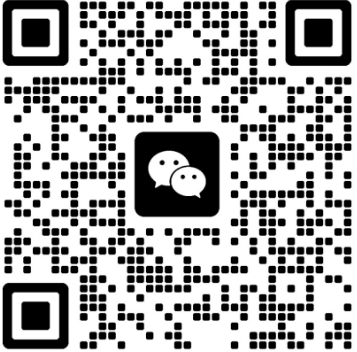