最近,谷歌在技术面试中频繁考察一些经典的算法题目,这些题目不仅考验候选人的编程能力,更注重他们的逻辑思维和解决问题的方式。在这篇文章中,我们将详细解析谷歌近期面试中的几道高频算法题,帮助你深入理解这些题目的解题思路和技巧。通过这些案例,你将更好地准备自己的面试,提升通过率。
题目1:在线匹配算法(Match-Making Algorithm)
英文原文
Create an online match-making algorithm that receives players and dispatches a call to a game server when it finds players with close enough skill ratings to play against each other.
中文翻译
创建一个在线匹配算法,该算法接收玩家,当发现两个技术水平接近的玩家时,将他们派送到游戏服务器进行对战。
澄清问题与解答
- 澄清问题1: "What would be an example input and output of this question?"
- 解答: 假设玩家的评分如下:玩家1的评分为1500,玩家2的评分为1490,玩家3的评分为1600。当玩家加入时,系统应该将评分最接近的两个玩家进行匹配。
- 澄清问题2: "So there is an existing GameServer class and we can do something like GameServer.dispatch()?"
- 解答: 是的,我们假设存在一个
GameServer
类,并且可以使用GameServer.dispatch(<玩家对>)
这样的调用来启动游戏。
- 解答: 是的,我们假设存在一个
攻略
这道题目主要考察了如何通过匹配算法找到评分相近的玩家对,并将他们匹配在一起进行游戏。题目的核心难点在于如何高效地找到最接近的玩家对,并在匹配成功后从等待队列中移除他们。
解题思路:
- 创建一个
MatchMaking
类,包含一个用于存储玩家队列的数组和一个定义“足够接近”的评分差异的阈值。 - 当有玩家加入时,将其加入队列并尝试与队列中的其他玩家进行匹配。
- 遍历队列,寻找评分最接近的玩家对,如果找到符合条件的对,将他们从队列中移除并调用
GameServer.startGame(player1, player2)
开始游戏。
通过这种方式,系统可以有效地处理玩家的匹配需求。
题目2:字符串匹配与音译(String Matching with Transliteration)
英文原文
You may or may not know that Google Docs has a feature to find and replace even for characters not in the standard English alphabet (A-Z). To do this, we first need a transliteration map. Given a haystack string and a needle string and the transliteration mapping, return whether the needle is matched within the haystack.
中文翻译
你可能知道或不知道,Google Docs 有一个功能,可以查找和替换非标准英文字母(A-Z)的字符。为此,我们首先需要一个音译映射表。给定一个长字符串(haystack)和一个短字符串(needle)以及音译映射表,返回短字符串是否与长字符串匹配。
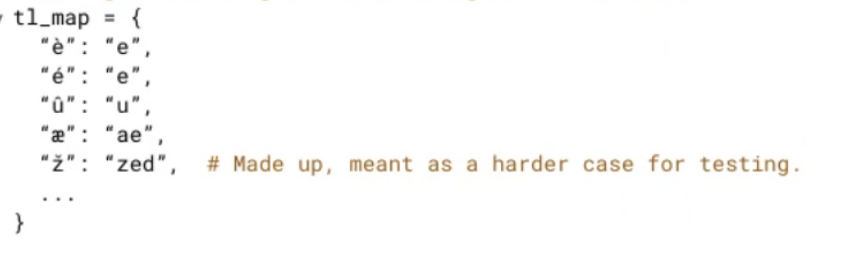
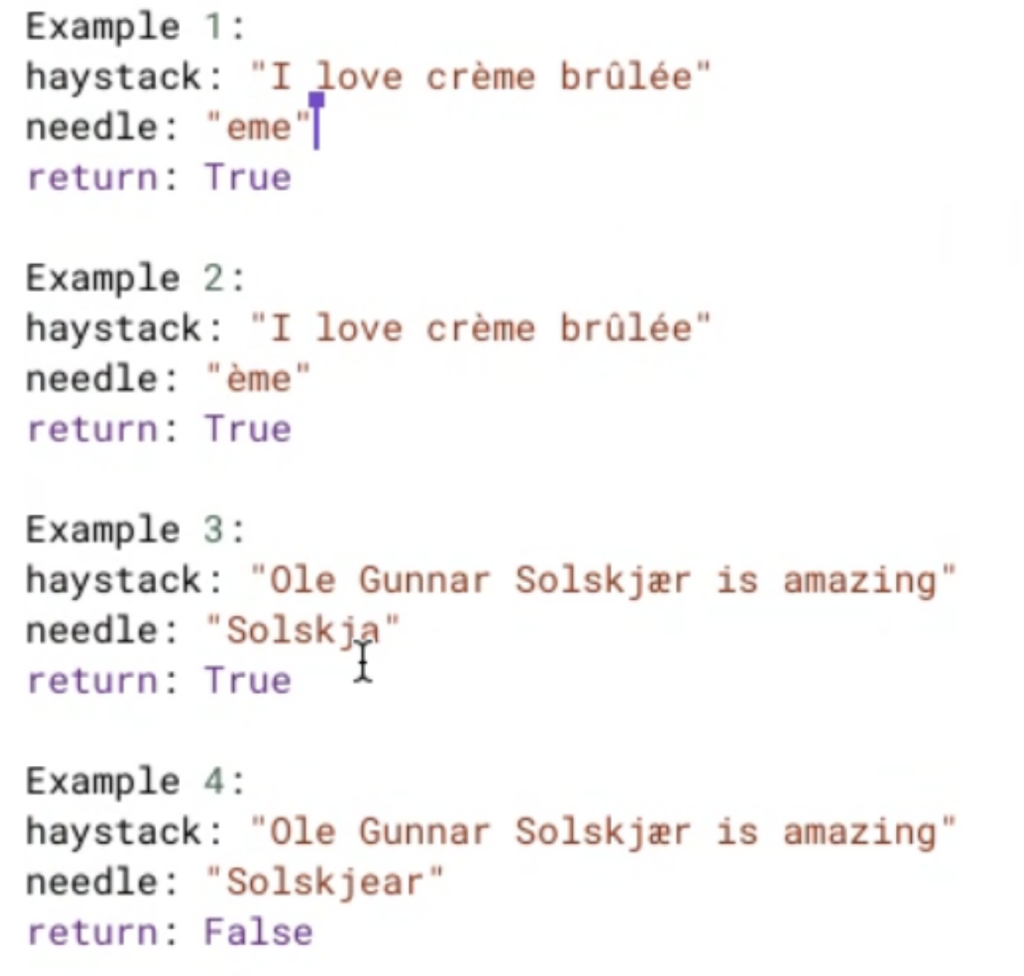
澄清问题与解答
- 澄清问题1: "So basically we want to know if every character in needle is contained by haystack in the same order, and it could either be the original character or the corresponding character based on tl_map?"
- 解答: 是的,我们需要判断
needle
中的每个字符是否按照顺序包含在haystack
中,并且这些字符可以是原始字符或根据tl_map
转换后的字符。
- 解答: 是的,我们需要判断
攻略
这道题目要求设计一个能够处理字符音译的字符串匹配算法。核心问题在于如何判断一个字符串是否在另一个字符串中,且可以允许一定的字符替换。
解题思路:
- 使用两个指针分别遍历
haystack
和needle
。 - 对每对字符,检查它们是否相等,或者根据音译映射表
tl_map
是否可以互相替代。 - 如果找到一个匹配的字符对,则移动
needle
和haystack
的指针,否则只移动haystack
的指针。 - 如果成功遍历
needle
中的所有字符,则匹配成功。
这个思路确保了我们能够处理字符替换的情况,并且可以高效地进行字符串匹配。
题目3:餐厅等候名单管理(Restaurant Waitlist Management)
英文原文
Implement a restaurant waitlist data structure. It should support the following features:
- A party of customers can join the waitlist.
- A previously joined party can leave the waitlist at any time.
- The restaurant can ask the data structure for the first party that fits a given table size (a table size is given as an argument).
中文翻译
实现一个餐厅的等候名单数据结构。该数据结构应支持以下功能:
- 顾客可以加入等候名单。
- 已加入的顾客可以随时退出等候名单。
- 餐厅可以查询列表中第一个适合指定桌子大小的顾客(桌子大小作为参数提供)。
澄清问题与解答
- 澄清问题: "What happens if multiple parties fit the table size?"
- 解答: 系统应该返回列表中第一个适合的顾客。这意味着我们需要保持列表的顺序。
攻略
这道题目要求我们实现一个支持动态添加和删除操作的餐厅等候名单,并能够根据指定的桌子大小查找第一个符合条件的顾客。
解题思路:
- 使用一个列表来管理等候名单。
- 在顾客加入名单时,将其加入列表末尾。
- 当顾客离开时,将其从列表中移除。
- 查询时,遍历列表,找到第一个符合条件的顾客并返回。
通过这种方法,可以确保等候名单的管理高效且有序。
题目4:字符串匹配与替换(String Matching with Replacement)
英文原文
Design a string matching algorithm that can find a target string within a given text, even if the characters are not standard English letters and might be replaced with others.
中文翻译
设计一个字符串匹配算法,该算法可以在给定的文本中找到目标字符串,即使其中的字符不是标准的英文字母,也可能被替换为其他字符。
澄清问题与解答
- 澄清问题: "How do we handle cases where multiple characters could be replaced in different ways?"
- 解答: 我们可以使用一种字符替换映射表,并按顺序进行匹配。如果匹配失败则返回
False
。
- 解答: 我们可以使用一种字符替换映射表,并按顺序进行匹配。如果匹配失败则返回
攻略
这道题目与前面的音译题目类似,但更加复杂,因为它要求我们处理多个可能的字符替换。
解题思路:
- 创建一个映射表,存储可能的字符替换关系。
- 使用双指针算法遍历长短字符串,并按顺序进行匹配。
- 如果匹配成功,则继续,如果失败则返回
False
。
这种方法确保了我们可以处理复杂的字符替换匹配问题。
题目5:岛屿撤离计划(Island Evacuation Plan)
英文原文
You're on a remote island that needs evacuation due to pending flooding. The government is sending planes tomorrow to get some people out. If you miss the last plane, you'd have to take a slower boat the following day which you don't want. Given the plane schedule and the schedule of all your fellow islanders, what is the latest you could get to the airport tomorrow and still get evacuated?
中文翻译
你在一个偏远的岛屿上,岛屿即将被洪水淹没。政府明天会派飞机来接走部分人。如果错过最后一班飞机,你就得乘坐第二天的慢船离开,而你并不想这样。给定飞机的时间表以及所有岛民的到达时间表,计算你最晚能到达机场并成功撤离的时间。
澄清问题与解答
- 澄清问题: "What if multiple people have the same latest time?"
- 解答: 系统应考虑所有可能的时间,并找出最适合的撤离时间。
攻略
这道题目要求我们找到在不影响撤离计划的情况下,能够最晚到达机场的时间点。
解题思路:
- 根据飞机的起飞时间表和所有岛民的时间表,找出每个岛民能够成功登机的最晚时间。
- 计算所有可能的撤离时间点,并选择一个最优的时间点。
通过这种方法,可以确保每个岛民在最晚的时间点成功撤离。
题目6:字符串匹配算法(String Matching Algorithm)
英文原文
Given a haystack string and a needle string and the transliteration mapping, return whether the needle is matched within the haystack.
中文翻译
给定一个长字符串(haystack)和一个短字符串(needle)以及音译映射表,返回短字符串是否与长字符串匹配。
澄清问题与解答
- 澄清问题: "What if the transliteration mapping is ambiguous?"
- 解答: 在这种情况下,我们应按照最直接的方式进行匹配,并在发现冲突时返回
False
。
- 解答: 在这种情况下,我们应按照最直接的方式进行匹配,并在发现冲突时返回
攻略
这道题目是对字符串匹配算法的进一步挑战,要求我们在字符替换时处理可能的模糊匹配。
解题思路:
- 使用双指针遍历
haystack
和needle
。 - 对每对字符,检查它们是否可以通过直接匹配或替换匹配成功。
- 如果成功,则继续匹配,如果失败则返回
False
。
这种方法保证了匹配过程的高效性,同时能够处理复杂的字符替换关系。
在这次谷歌面试中,候选人面对了两道具有挑战性的算法题,通过与面试官的互动,不仅展示了解题思路,还展现了良好的沟通能力和问题解决能力。
如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
In this Google interview, I demonstrated my understanding of common algorithmic problems and my problem-solving abilities. Each problem presented different challenges, but all could be solved through logical algorithm strategies.
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
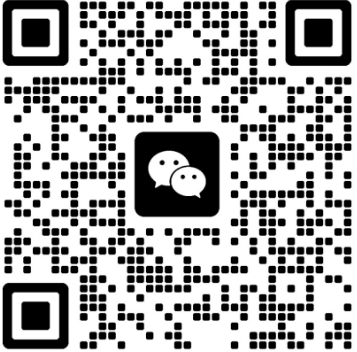