在近期的亚马逊面试中,候选人被要求实现一个程序,用于根据不同因素来计算披萨的价格。这道题很好地展示了面向对象设计原则在解决实际问题中的应用。让我们深入分析问题,并探索如何构建解决方案。
In a recent Amazon interview, candidates were asked to implement a program that calculates the price of a pizza based on several factors. This question is a great example of how object-oriented design principles are applied to solve practical problems. Let's dive into the problem and explore how to structure a solution.
题目描述 (Problem Statement):
Write a program that allows the user to calculate the price of a pizza. A pizza has:
- a base
- a size
- toppings
Assume the system stores everything in-memory, no storage required.
题目要求候选人编写一个程序,允许用户计算披萨的价格。每个披萨有以下几个可定制的组件:
- Base:例如薄底、厚底、无麸质底等;
- Size:例如小号、中号、大号;
- Toppings:例如奶酪、意大利香肠、蘑菇、洋葱、橄榄等。
系统不需要持久化存储所有这些组件,只需要在内存中管理即可。
Problem Statement:
You are required to write a program that allows the user to calculate the price of a pizza. Each pizza has the following customizable components:
- Base: For example, thin crust, thick crust, gluten-free, etc.
- Size: For example, small, medium, large.
- Toppings: For example, cheese, pepperoni, mushrooms, onions, olives, etc.
The program will need to store all these components in-memory and does not require persistent storage.
澄清问题 (Clarifications):
在进入编码之前,有几个问题需要澄清:
- Size Categories:尺寸有具体分类吗?比如小号、中号、大号?我能假设不同尺寸有不同的价格系数吗?例如小号是基础价格的0.8倍,中号是1.5倍,大号是2倍?
- Base Options:披萨底有不同的选项吗?例如薄底、厚底、无麸质底?
- Topping Choices:配料包含哪些选项?是否包括奶酪、意大利香肠、蘑菇等标准配料?每个配料是否有固定价格?
在得到面试官的确认后,假设如下:
- 尺寸会按价格系数计算:
- 小号 = 基础价格的0.8倍,
- 中号 = 基础价格的1.5倍,
- 大号 = 基础价格的2倍。
- 披萨底选项价格固定:
- 薄底 = $5,
- 厚底 = $7,
- 无麸质底 = $6。
- 每种配料有预设的固定价格:
- 奶酪 = $2,
- 意大利香肠 = $3,
- 蘑菇 = $1.5,
- 洋葱 = $1,
- 橄榄 = $1.2。
Clarifications:
Before jumping into the coding, some questions need to be clarified:
- Size Categories: What are the specific sizes, and how are their prices scaled? Can we assume small, medium, and large, with price factors like small = 0.8x, medium = 1.5x, and large = 2x the base price?
- Base Options: Will there be different options for the base, such as thin crust, thick crust, or gluten-free?
- Topping Choices: Will the toppings include options like cheese, pepperoni, mushrooms, and other standard choices? Additionally, will each topping have a fixed cost?
After receiving confirmation from the interviewer, the assumptions were set as follows:
- The sizes will follow a price factor, where:
- Small = 0.8x the base price,
- Medium = 1.5x the base price,
- Large = 2x the base price.
- The base options will have fixed prices:
- Thin Crust = $5,
- Thick Crust = $7,
- Gluten-Free = $6.
- Each topping will have a predefined cost:
- Cheese = $2,
- Pepperoni = $3,
- Mushrooms = $1.5,
- Onions = $1,
- Olives = $1.2.
解决方案设计 (Solution Design):
为了解决这个问题,我们可以使用面向对象编程(OOP),通过枚举类来表示披萨的不同组件(底、尺寸、配料)。以下是具体的解决方案设计:
- 枚举类:这些类表示披萨的底、尺寸和配料的不同选项,每个选项都有其关联的成本或价格系数。
- Pizza 类:该类表示披萨本身,保存有关底、尺寸和配料的信息。它还包括计算披萨总价的方法。
- 计算逻辑:通过将披萨底的成本和所有配料的成本相加,然后乘以尺寸的价格系数,计算披萨的总价格。
Solution Design:
To structure the solution, we can use object-oriented programming (OOP) with enumerations representing different pizza components (Base, Size, Topping). Here's how we can break down the solution:
- Enumeration Classes: These classes represent the different possible values for the pizza's base, size, and toppings, each with their associated costs or price factors.
- Pizza Class: This class will represent the pizza itself, holding information about its base, size, and toppings. It will also include methods to calculate the total price based on the selected options.
- Calculation Logic: The total cost of the pizza is calculated by adding the base cost and the cost of all selected toppings, then multiplying by the size's price factor.
算法思路 (Algorithm Outline):
该解决方案将按以下步骤进行:
- 设置枚举类:表示披萨底、尺寸和配料及其各自的成本。
- 创建 Pizza 类:用于存储披萨的底和尺寸,并能动态添加配料。
- 实现价格计算:通过将底价和配料的总价相加,然后应用尺寸乘数来计算总价。
- 处理用户输入:模拟披萨的定制(这一步可以根据命令行环境或图形用户界面进行调整)。
Algorithm Outline:
The solution will follow these steps:
- Set up enumerations for the pizza base, size, and toppings with their respective costs.
- Create a Pizza class that can store the base and size of the pizza and add toppings dynamically.
- Implement price calculation by summing up the base cost and the cost of the toppings, then applying the size multiplier.
- Handle user input to simulate the customization of the pizza (this step can be adapted depending on whether you are in a command-line environment or using a GUI).
示例讲解 (Example Walkthrough):
让我们演示一个场景,用户想要计算一个大号披萨的价格,披萨为薄底,配料为奶酪、意大利香肠和蘑菇。
- 底:薄底 = $5
- 尺寸:大号 = 价格系数2倍
- 配料:
- 奶酪 = $2
- 意大利香肠 = $3
- 蘑菇 = $1.5
价格计算如下:
- 配料总价 = $2 + $3 + $1.5 = $6.5
- 底价 = $5
- 总价(尺寸乘数前) = $5 + $6.5 = $11.5
- 最终价格(大号尺寸乘数) = $11.5 * 2 = $23
因此,这个披萨的价格为 $23。
Example Walkthrough:
Let's walk through a scenario where a user wants to calculate the price of a large pizza with a thin crust and toppings of cheese, pepperoni, and mushrooms.
- Base: Thin Crust = $5
- Size: Large = 2x price factor
- Toppings:
- Cheese = $2
- Pepperoni = $3
- Mushrooms = $1.5
The price calculation would work as follows:
- Total Topping Cost = $2 + $3 + $1.5 = $6.5
- Base Cost = $5
- Total Before Size Factor = $5 + $6.5 = $11.5
- Final Price (with Large Size Factor) = $11.5 * 2 = $23
Thus, the price of the pizza is $23.
总结 (Summary):
这个问题强调了面向对象设计和模块化思维在解决类似披萨定制订单的实际任务时的重要性。通过将任务拆分为底、尺寸和配料等组件,并根据不同的价格系数和固定成本进行应用,问题的解决变得更加直观和清晰。
在这场面试中,候选人展示了澄清假设、处理动态输入,并有效利用面向对象原则的能力,这些都是软件工程中的关键技能。
This problem emphasizes the importance of OOP design and modular thinking when solving real-world tasks like customizing a pizza order. By breaking down the task into smaller components like base, size, and toppings, and applying price factors accordingly, the problem becomes straightforward to solve.
In the interview, the candidates demonstrated the ability to clarify assumptions, handle dynamic inputs, and effectively utilize object-oriented principles, which are essential skills in software engineering.
We provide interview assistance and proxy interview services to help you get into your dream company. Feel free to contact us anytime.
我们提供面试辅助,代面试服务,助您进入梦想大厂,欢迎随时咨询我。
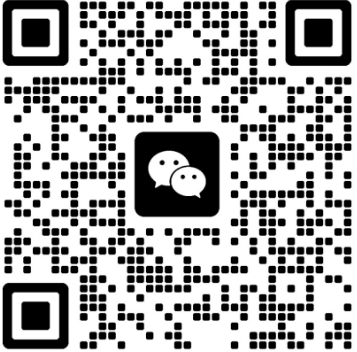