1. Spring Dependency Injection (Book Recommendation Service)
Task:
- Implement a book recommendation service where
BookRepository
should be injected intoRecommendationService
using Spring dependency injection. - Missing annotations should be added so that
RecommendationService
andBookRepository
are recognized as Spring beans and Spring injectsBookRepository
properly.
Code Snippet Example:
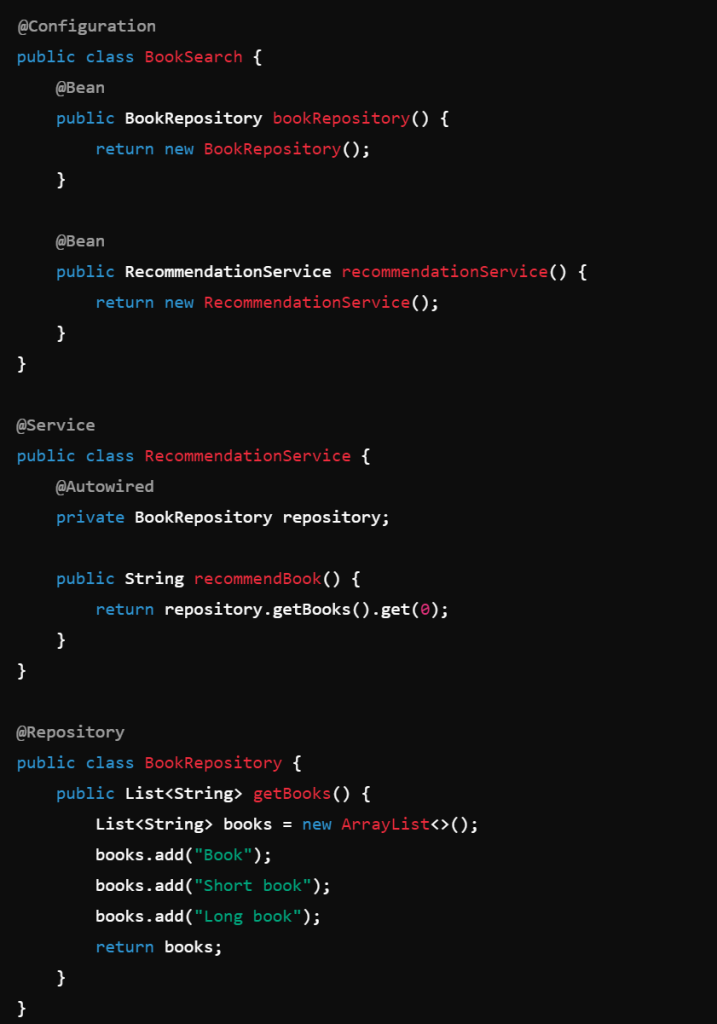
2. Spring MVC: Appointment Controller (Multiple Choice Question)
Question:
Based on the provided code, select the correct statements (select all that apply):
Code Snippet:
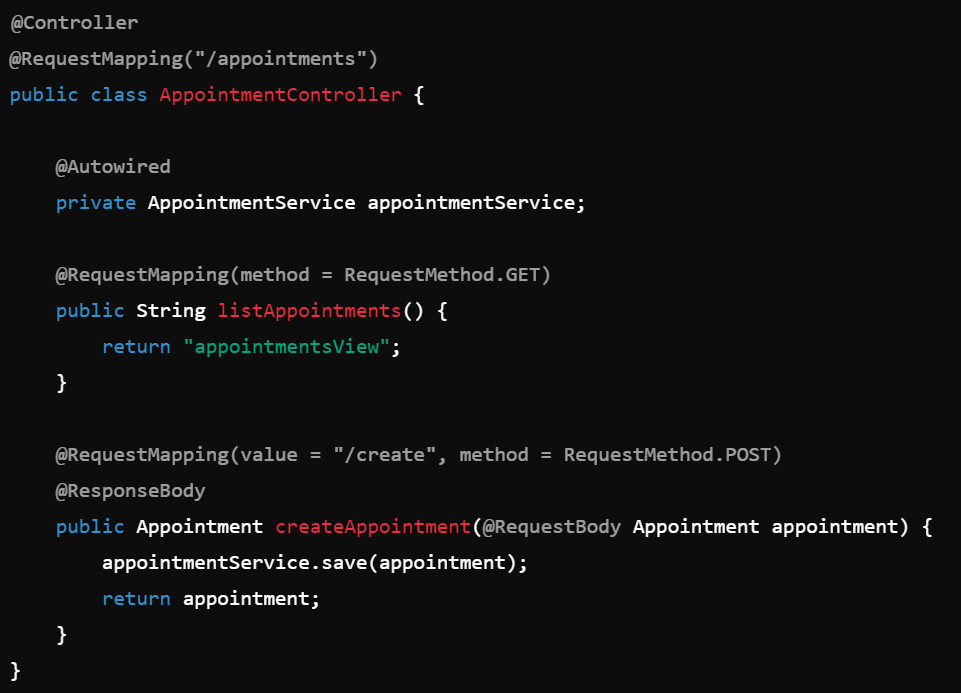
Options:
- A. Calling
createAppointment()
will result in an error as the@RequestBody
annotation can only be used with either aString
or primitive types. - B.
createAppointment()
will be called only if a user submits an HTTP POST request to/appointments/create
. - C. Values returned to the client by
@ResponseBody
methods are automatically mapped to a specific data format (e.g. XML, JSON) depending on Spring's configuration and theaccept
HTTP header. - D.
listAppointments()
will be called when a user visits/appointments/get
. - E. The content served by
listAppointments()
will be returned with the HTTP header "Content-Type: text/plain".
Correct Answers:
- B:
createAppointment()
will be called only if a user submits an HTTP POST request to/appointments/create
. - C: Values returned to the client by
@ResponseBody
methods are automatically mapped to a specific data format (e.g. XML, JSON) depending on Spring's configuration and theaccept
HTTP header.
3. Algorithm: findMaxSum
Method
Task:
- Implement the
findMaxSum
method that, efficiently with respect to time, returns the largest sum of any two elements in the given list of positive numbers.
Example:
For example, the largest sum of the list { 5, 9, 7, 11 }
is the sum of the elements 9 and 11, which is 20.
Code Snippet:
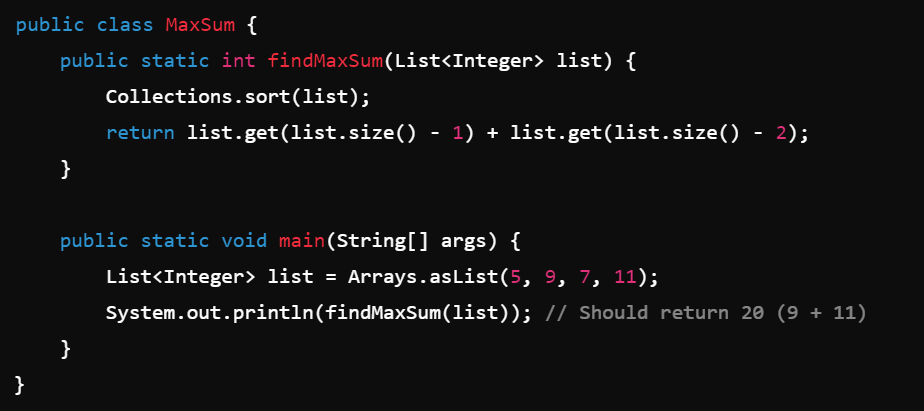
4. JUnit Test Cases: Shelf
Class
Task:
- Write unit tests using JUnit 4's
Assert
class for aShelf
class. - The tests should cover the following scenarios:
- The
put
method will do nothing when passednull
. It should also do nothing when passed an empty string. - Each individual item can only be taken from the shelf once.
- Duplicate items can exist on the shelf at the same time.
- The
Code Snippet:
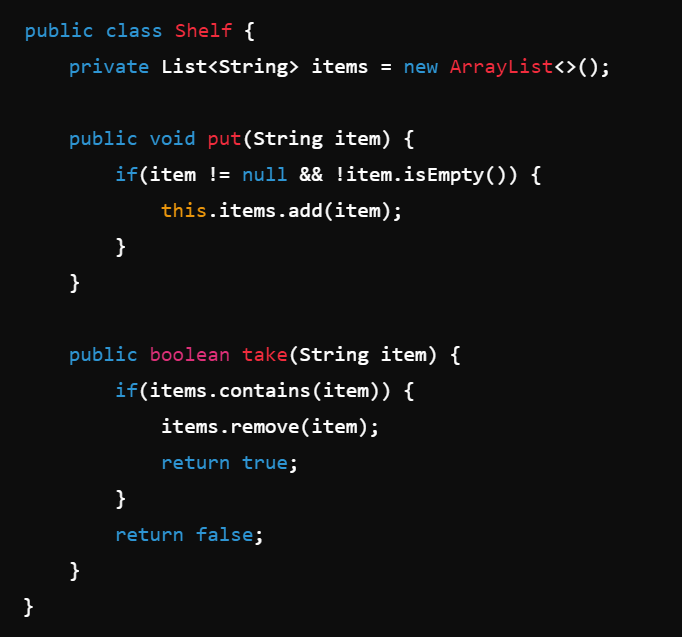
If you need more interview support or interview proxy practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.
如果您需要面试辅助或面试代面服务,帮助您进入梦想中的大厂,请随时联系我。
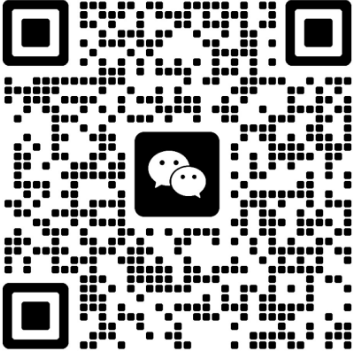