在本文中,我将分享谷歌的两个面试题目,并尽可能详细地还原面试场景以及与面试官的对话。这些题目涉及树的遍历以及经典的井字游戏胜负判断,旨在考察候选人对算法的理解与编程能力。
In this blog post, I will share two interview questions from Google, along with a detailed simulation of the interview scenario, including the dialogue with the interviewer. These questions involve tree traversal and the classic Tic-Tac-Toe game outcome determination. The goal is to assess a candidate's understanding of algorithms and coding skills.
题目 1:树中的岛屿数量
题目描述:
在一个由0和1组成的树中,岛屿被定义为由1组成的组,且这些1被0所包围,或者位于树的边界上。
示例:
0(1)
/ \
1(2) 1(3)
/ \
0(5) 1(4)
/ \
1(6) 1(7)
\ \
1(8) 1(9)
在上面的示例中,一共有4个岛屿。
问题:
计算树中岛屿的数量。
追问 1:
找到不同岛屿大小的数量。
- 大小定义为岛屿中1的数量。
- 在上面的示例中,一共有2种不同的岛屿大小(1和2)。
Question 1: Counting the Number of Islands in a Tree
Problem Description:
In a tree composed of zeros and ones, an island is defined as a group of ones surrounded by zeros or located at the boundary of the tree.
Example:
0(1)
/ \
1(2) 1(3)
/ \
0(5) 1(4)
/ \
1(6) 1(7)
\ \
1(8) 1(9)
In the above example, there are 4 islands.
Task:
Count the number of islands in the tree.
Follow-up 1:
Find the number of distinct island sizes.
- The size is defined as the number of ones in the island.
- In the above example, there are 2 distinct island sizes (1 and 2).
面试过程还原
面试官: “你能简要地解释一下你会如何解决这个问题吗?”
我: “当然,我首先想到的方法是使用深度优先搜索(DFS)。每次当我遇到一个值为1的节点时,我将检查它的父节点是否为0。如果是这样,那么这个节点就是一个新的岛屿的起点。我会递归遍历所有的子节点,同时传递当前节点的值,以确保岛屿的计数是正确的。”
面试官: “这听起来不错。你能详细解释一下如何处理边界情况吗?”
我: “对于边界情况,如果根节点本身为1,那么它将被视为一个岛屿。对于其他节点,只要它的父节点为0,并且自身为1,我们就会递增岛屿的数量。”
面试官: “很好。假设你已经解决了第一个问题,现在我们来看第二个问题——如何计算不同岛屿大小的数量?”
我: “为了计算岛屿的大小,我会在递归中增加一个计数器。当一个岛屿遍历完成后,我将记录该岛屿的大小,并将其存储在一个集合中。集合的大小即为不同岛屿大小的数量。”
Interview Process Simulation
Interviewer: "Can you briefly explain how you would approach solving this problem?"
Me: "Certainly. The first method that comes to mind is using Depth-First Search (DFS). Every time I encounter a node with a value of 1, I will check if its parent node has a value of 0. If so, this node marks the beginning of a new island. I would then recursively traverse all its child nodes while passing the current node's value to ensure the island count is accurate."
Interviewer: "That sounds good. Could you explain in more detail how you would handle edge cases?"
Me: "For edge cases, if the root node itself is 1, then it would be considered an island. For other nodes, as long as their parent node is 0 and the current node is 1, we would increment the island count."
Interviewer: "Great. Let's assume you've solved the first problem. Now, let's move on to the follow-up question—how would you calculate the number of distinct island sizes?"
Me: "To calculate the island sizes, I would add a counter in the recursion. When an island is fully traversed, I would record the size of the island and store it in a set. The size of the set would then represent the number of distinct island sizes."
面试过程中的讨论
面试官: “对于这个算法,有没有什么特殊的注意点或者是你认为需要优化的地方?”
我: “是的,我认为在递归过程中需要特别注意的是,在每一个节点进行递归调用时,要传递父节点的值。这样可以确保我们在遍历时能够正确地识别出岛屿的边界,并且在处理不同的情况时可以有所区分。”
面试官: “这个想法很好。那么在遇到岛屿的过程中,你会如何计算岛屿的大小呢?”
我: “每次进入一个新的岛屿时,我会启动一个大小计数器。当递归遍历完这个岛屿的所有节点后,我会将这个大小计数器的值记录下来,并将其加入一个集合中。这样可以确保即使是相同大小的岛屿,也会被正确地记录和区分。”
面试官: “明白了。那么在处理多个相邻岛屿时,你会如何确保它们不会被重复计算?”
我: “在每次遍历一个新的节点时,我会首先检查它的父节点的值。如果父节点为0,而当前节点为1,那么这就标志着一个新的岛屿的开始。接着,我会通过递归遍历这个岛屿的所有部分,确保这个岛屿的每个节点只会被计算一次。这样可以避免重复计数的问题。”
Discussion During the Interview
Interviewer: "Are there any special considerations or optimizations you would make for this algorithm?"
Me: "Yes, I think it's important to ensure that the parent node's value is passed correctly during recursion. This helps identify the boundaries of the islands and distinguish between different cases."
Interviewer: "This makes sense. How would you ensure that islands are not counted multiple times when dealing with adjacent islands?"
Me: "Each time I traverse a new node, I would first check its parent's value. If the parent node is 0 and the current node is 1, this signifies the start of a new island. I would then recursively traverse all parts of the island, ensuring that each node within the island is counted only once. This prevents duplicate counting."
题目 2:井字游戏
题目描述:
确定井字游戏中某一玩家的回合后的游戏结果。
参数说明:
- @param board 当前游戏棋盘的状态。3x3的二维数组,其中:
- 'X' 代表玩家X的标记
- 'O' 代表玩家O的标记
- ' ' 代表空格
返回值说明:
- 返回游戏的结果:
- “X” 如果玩家X获胜
- “O” 如果玩家O获胜
- “Tie” 如果游戏平局
- “None” 如果游戏还在继续
Question 2: Tic-Tac-Toe Game Outcome
Problem Description:
Determine the game outcome after a player's turn in Tic-Tac-Toe.
Parameter Explanation:
- @param board: The current state of the game board, represented by a 3x3 array where:
- 'X' represents Player X's mark.
- 'O' represents Player O's mark.
- ' ' represents an empty cell.
Return Value Explanation:
- Return the outcome of the game:
- "X" if Player X won.
- "O" if Player O won.
- "Tie" if the game is a draw.
- "None" if the game is still ongoing.
面试过程还原
面试官: “现在我们来看第二个问题,关于经典的井字游戏。你能告诉我如何判断游戏的结果吗?”
我: “当然可以。井字游戏是一个3x3的棋盘游戏,我会通过检查棋盘的每一行、每一列以及两个对角线来判断是否有玩家获胜。如果在这些检查中发现有一条线上的三个格子都被同一个玩家占据,那么这个玩家就是赢家。”
面试官: “这个方法听起来很合理。你认为这个方法的时间复杂度是多少?”
我: “对于一个3x3的棋盘,检查每一行、每一列以及对角线总共需要检查8条线,每条线最多需要检查3个格子。所以时间复杂度是O(1),也就是常数时间。即使棋盘变大,这个方法的时间复杂度也不会改变,依然是常数时间,因为我们总共只需要检查有限的几个方向。”
面试官: “很好。那么,如果游戏还未结束,也就是说棋盘上还有空位但没有玩家获胜,你会返回什么?”
我: “如果没有玩家获胜并且棋盘上仍然有空位,我会返回‘None’,表示游戏还在进行中。如果棋盘已经填满而没有玩家获胜,我会返回‘Tie’,表示游戏以平局结束。”
面试官: “非常好。你在处理这个问题时,有没有什么其他的优化思路?”
我: “我认为在实际编写代码时,可以通过优化循环结构来减少冗余代码。此外,还可以为每个可能的结果编写单元测试,以确保代码在所有情况下都能正确运行。”
面试官: “好的,听起来你对这个问题的理解很深刻。”
Interview Process Simulation
Interviewer: "Now, let's move on to the second problem, which involves the classic Tic-Tac-Toe game. Can you tell me how you would determine the outcome of the game?"
Me: "Certainly. Tic-Tac-Toe is a 3x3 board game. I would check each row, each column, and both diagonals to see if there is a winner. If any of these lines have three identical marks, that player is the winner."
Interviewer: "This approach seems reasonable. What do you think the time complexity of this method would be?"
Me: "For a 3x3 board, checking each row, column, and diagonal involves checking a total of 8 lines, with each line containing at most 3 cells. So, the time complexity is O(1), which is constant time. Even if the board size increases, the time complexity remains O(1) because we are only checking a fixed number of directions."
Interviewer: "Good. What would you return if the game has not ended, meaning there are still empty cells but no player has won?"
Me: "If there is no winner and there are still empty cells, I would return 'None', indicating that the game is still ongoing. If the board is full and there is no winner, I would return 'Tie', indicating that the game has ended in a draw."
Interviewer: "Excellent. Do you have any other thoughts or optimizations for this problem?"
Me: "In practice, I would optimize the loop structure to reduce redundant code. Additionally, I would write unit tests for all possible edge cases to ensure the code works correctly in all scenarios."
Interviewer: "Sounds like you have a good grasp of the problem."
总结
在这次谷歌的面试中,我们讨论了两个经典的算法问题。第一个问题考察了候选人对树的遍历以及递归算法的理解,尤其是在复杂结构中如何正确识别和计数的能力。第二个问题则集中在经典的井字游戏的胜负判断上,考察了候选人对二维数组的操作以及如何通过简单高效的方法判断游戏结果的能力。
这次面试不仅考察了算法和数据结构的基本功,还注重了候选人在面对问题时的分析能力和逻辑思维。通过详细的讨论和反复的问答,可以看出谷歌对候选人思考过程的重视,以及对代码正确性和效率的高标准要求。
如需更多面试辅导和模拟面试,请随时联系我们。我们提供全面的面试辅助服务,帮助你顺利进入梦想中的大厂。
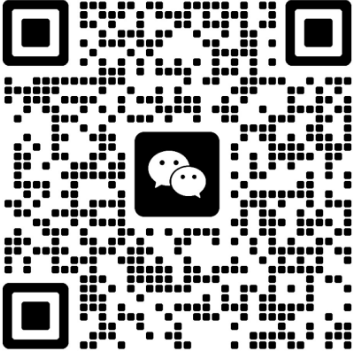
Conclusion
In this Google interview, we discussed two classic algorithm problems. The first problem tested the candidate's understanding of tree traversal and recursive algorithms, particularly in identifying and counting islands in a complex structure. The second problem focused on determining the outcome of a Tic-Tac-Toe game, testing the candidate's ability to efficiently operate on a 2D array and determine the game result using a straightforward, optimized approach.
This interview not only tested the fundamentals of algorithms and data structures but also emphasized the importance of analytical skills and logical thinking when faced with a problem. Through detailed discussions and back-and-forth questioning, it was evident that Google places a high value on the candidate's thought process and the correctness and efficiency of their code.
If you need more interview coaching or mock interview practice, feel free to contact us. We offer comprehensive interview support services to help you successfully land a job at your dream company.