Today, I will share an interesting interview question from TikTok. This question requires designing a car dealership appointment scheduling system to handle customer bookings with employees. Below is a detailed analysis and solution approach for this problem.
Problem Description
You are running a car dealership and currently have two employees. We need to build a basic function to manage customer appointments with your employees.
You have the following constraints:
- An appointment is represented by a pair of integers [start time, duration].
- Customers are assigned to either of your employees, and they don't get to choose who they get.
- Your two employees can only handle one customer at a time.
Implement a DealershipScheduler
class that has the following method:
cpp复制代码bool book(int startTime, int duration)
This method returns true
if you can book an appointment for these times and false
if it would result in your employees being double-booked.
Sample bookings:
css复制代码[10, 10], [50, 10], [10, 30], [5, 10], [5, 5], [25, 30]
Interview Dialogue
During the interview, the interviewer and I had the following conversation:
Interviewer: How would you handle conflicts in appointment scheduling?
Me: I would maintain a record of each employee's appointment time slots and check for conflicts whenever a new appointment request is made. If there are no conflicts, the appointment can be assigned to one of the employees.
Interviewer: What data structures would you use to store the appointment information?
Me: I would use two lists to store the appointment time slots for each employee. Each time a new appointment request is made, I would check these lists for conflicts.
Interviewer: How would you optimize this process to ensure efficiency as the number of appointment requests increases?
Me: I could use ordered lists or balanced binary trees to store the appointment time slots, which would help maintain efficiency during insertions and queries.
Solution Approach
- Conflict Detection:
- For each appointment request, check if the requested time slot conflicts with existing appointments.
- If no conflicts are found, the appointment can be booked for one of the employees.
- If conflicts are found, check the other employee's schedule.
- Data Structure Choice:
- Use two lists to store the appointment time slots for the two employees.
- When inserting a new appointment, check for conflicts in these lists.
- Appointment Handling Process:
- Iterate through the existing appointment time slots and check for overlaps with the new appointment time slot.
- If overlaps are found, return
false
. - If no overlaps are found, add the new appointment time slot to the list and return
true
.
Implementation Steps
- Initialization:
- Create two lists to store the appointment time slots for the two employees.
- Conflict Detection:
- Define a method
hasConflict
to check if the new appointment time slot conflicts with existing time slots. - Iterate through the list and check for overlapping time slots.
- Define a method
- Handle Appointment Requests:
- In the
book
method, call thehasConflict
method to check the appointment time slots in both lists. - If one list has no conflicts, add the new appointment time slot to that list.
- In the
Example Code (Pseudocode)
To better understand the process, here is a simple pseudocode example:
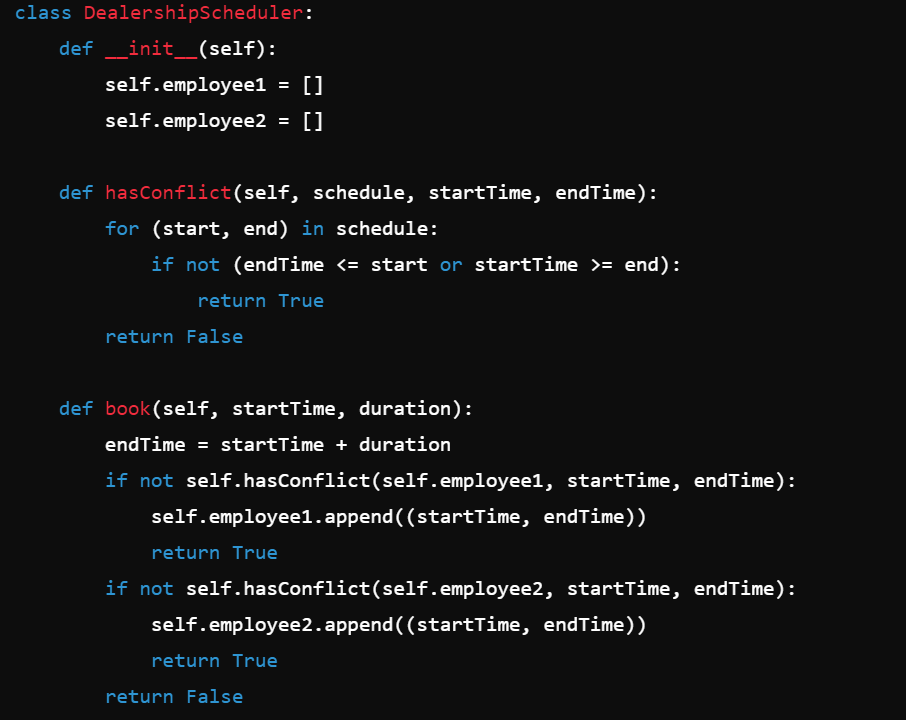
Optimizations and Improvements
- Using Ordered Lists:
- Use ordered lists to store the appointment time slots, improving insertion and query efficiency.
- Using Balanced Binary Trees:
- Implement balanced binary trees (e.g., Red-Black Tree or AVL Tree) to store appointment time slots, ensuring O(log n) time complexity for insertions and queries.
- Concurrency Handling:
- In a multi-threaded environment, use locking mechanisms to protect shared data structures from concurrent access issues.
Conclusion
This interview question tests our understanding of conflict detection in scheduling and our ability to implement efficient algorithms.
We provide interview assistance and proxy interview services to help you get into your dream company. Feel free to contact us anytime.
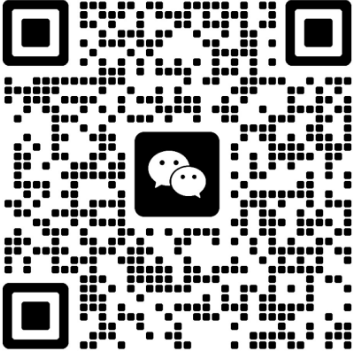