Create a class that allows you to build a directed graph of letters.
A directed graph contains Nodes, uniquely identified by a letter.
A directed graph contains Edges, which connect the Nodes as (from, to).
Your class should allow for the following operations:
- Add a letter to the graph.
- Is a letter in the graph?
- Add an edge, specifying the letter "from" and "to".
- For a given letter, get all the letters it leads to (by edges).
- Remove an edge, specifying the letter "from" and "to".
- Remove a letter, but not if it is involved in an edge.
Choose a language and begin:
创建一个类,允许你构建一个字母的有向图。
有向图包含节点,每个节点由一个字母唯一标识。
有向图包含边,连接节点的形式为 (from, to)。
你的类应该允许以下操作:
- 向图中添加一个字母。
- 检查一个字母是否在图中。
- 添加一条边,指定字母“from”和“to”。
- 对于给定的字母,获取所有它指向的字母(通过边)。
- 删除一条边,指定字母“from”和“to”。
- 删除一个字母,但如果它涉及到某条边则不能删除。
选择一种语言并开始:
This problem is a recent interview question from Amazon, focusing on Object-Oriented Design (OOD). It requires designing a class to represent a directed graph of letters with specific operations. This task tests the candidate's ability to apply OOD principles to create a flexible and efficient data structure. Key operations include adding and removing nodes and edges, checking for node existence, and retrieving adjacent nodes.
Solution Approach
- Class Definition:
- Define a class
DirectedGraph
. - Use a dictionary to store nodes and their corresponding edges for efficient lookups and updates.
- Define a class
- Attributes:
- Use a dictionary
graph
where keys are nodes and values are sets of adjacent nodes.
- Use a dictionary
- Methods:
add_node(letter)
: Add a node to the graph.contains(letter)
: Check if a node exists in the graph.add_edge(from_letter, to_letter)
: Add a directed edge from one node to another.get_adjacent(letter)
: Get all nodes that a given node leads to.remove_edge(from_letter, to_letter)
: Remove a directed edge between two nodes.remove_node(letter)
: Remove a node if it has no incoming or outgoing edges.
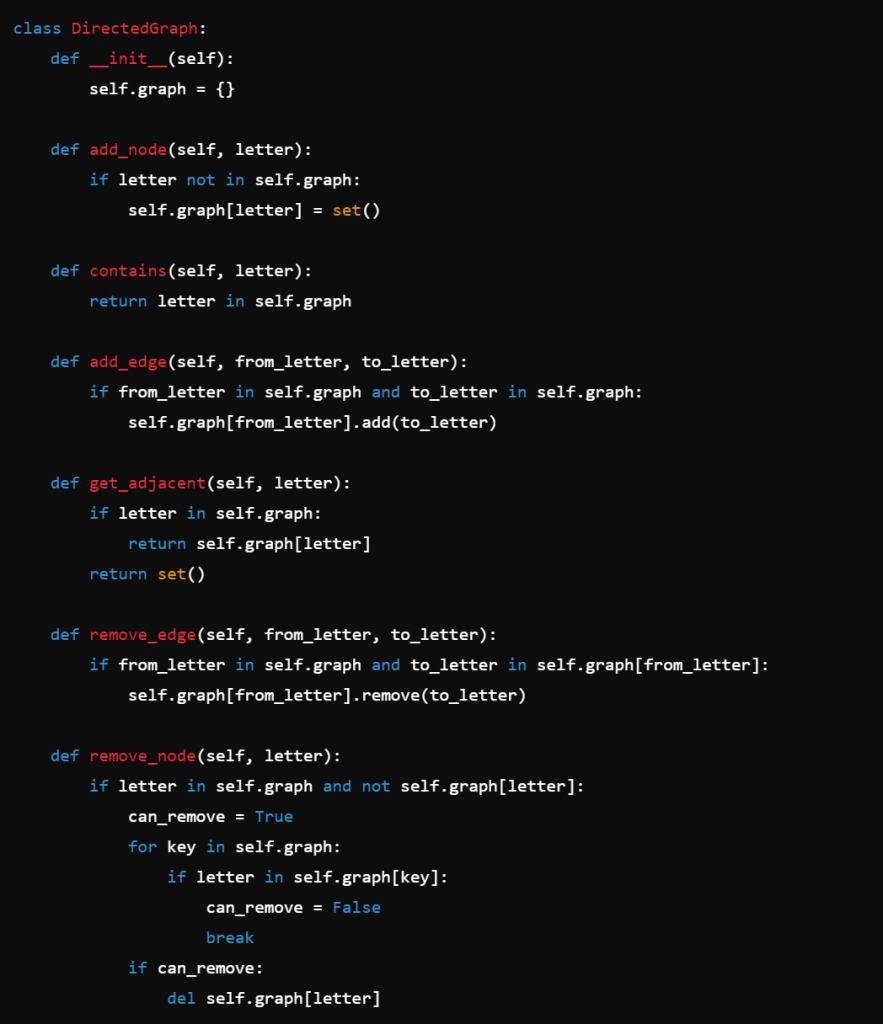
Explanation
- Initialization: The
DirectedGraph
class is initialized with an empty dictionary. - Add Node: The
add_node
method adds a new node if it doesn't already exist. - Check Existence: The
contains
method checks if a node is in the graph. - Add Edge: The
add_edge
method adds a directed edge from one node to another. - Get Adjacent Nodes: The
get_adjacent
method retrieves all nodes that the given node points to. - Remove Edge: The
remove_edge
method removes a directed edge between two nodes. - Remove Node: The
remove_node
method removes a node only if it has no edges connected to or from it, ensuring no data integrity issues.
With our powerful interview assistance, candidates can effectively analyze and communicate their solutions to these interview questions. This not only demonstrates their programming abilities to the interviewer but also showcases their clear problem-solving approach and effective communication skills. These abilities are valuable not only for Amazon interviews but also for solving real-world programming problems. We wish you all the best in your interviews!
If you need our interview assistance services, please contact us immediately.
面试辅助,面试代面,请联系我们。
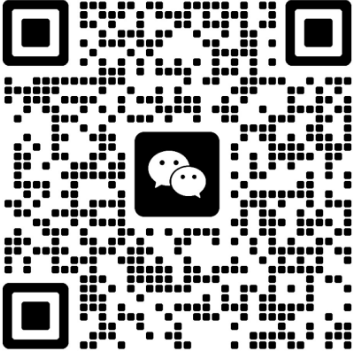