Recently, I encountered a challenging interview question from Google that tests the ability to handle connectivity problems in social networks. Today, I'll share how to solve this problem and discuss other critical skills needed to pass the interview.
Problem Statement
You are given a task to write a function that, given a list of all users and a set of timestamped logs recording when users become friends, determines the earliest time when everyone became reachable to every other person through the friends.
Example Log File:
rust复制代码1648305616 Alice and Bob become friends
1648305678 Charlie and Dan become friends
1648306171 Bob and Charlie become friends
1648306237 Alice and Erin become friends
Task Requirements:
- Input: List of all users and the log file.
- Output: The earliest time when all users are connected through friends.
Interview Simulation
Interviewer: "Hi, I'm XX. I've been with Google for almost 8 years now. I started as an intern right out of college and have been with the same team ever since, working on a variety of backend and frontend applications. The format of today's interview will be as follows: I'll start with a few behavioral questions for the first fifteen minutes or so, and then we'll spend the remaining time on a coding question. In the last five minutes, you'll have the opportunity to ask me any questions you might have about working at Google or anything else."
Candidate: "Sure, sounds good."
Behavioral Question 1
Interviewer: "Can you tell me about a time when you had to learn a completely new technology or tool to complete a project?"
Candidate: "In my previous role, I was assigned to a project that required using Docker for containerization, which I had no prior experience with. I started by taking an online course on Docker basics and followed several tutorials to understand how to create and manage containers. I also set up a small personal project to practice. This hands-on approach helped me quickly get up to speed, and I was able to successfully implement Docker in our project, improving deployment efficiency."
Interviewer: "How did you ensure that you were applying the new technology correctly?"
Candidate: "I made sure to read the official documentation thoroughly and regularly consulted with more experienced colleagues. Additionally, I participated in online forums and communities where I could ask questions and get feedback on my approach. This combination of resources ensured that I was using best practices and avoiding common pitfalls."
Behavioral Question 2
Interviewer: "Describe a situation where you had to work on a project with limited resources. How did you manage it?"
Candidate: "During one of my projects, we were short-staffed and had a tight deadline. I had to take on multiple roles, including project management and development. I prioritized tasks based on their impact and urgency, and I communicated frequently with stakeholders to manage expectations. I also automated repetitive tasks to save time. By staying organized and focused, we were able to complete the project on time without compromising on quality."
Interviewer: "What strategies did you use to stay organized and ensure the project's success?"
Candidate: "I used project management tools to track progress and deadlines. I also held daily stand-up meetings to keep the team aligned and identify any blockers early. Breaking down the project into smaller, manageable tasks helped us maintain a steady pace and achieve our goals step by step."
Coding Question
Interviewer: "Now, let's move on to the coding question. Given the log file and list of users, implement a function to find the earliest time when everyone became reachable to every other person through friends."
Candidate: "Got it. Just to clarify, should the function handle cases where not all users may become friends?"
Interviewer: "Yes, the function should return -1 if not all users become connected."
Candidate: "Okay, I'll start by thinking about the problem and planning my approach. I will use the Union-Find data structure to keep track of connected components and process the logs in chronological order. This will help us determine the earliest time when all users are connected."
Interviewer: "That sounds like a solid approach. Go ahead and explain your thought process as you write the code."
Candidate: "Sure. Here’s the function definition and my approach:
- First, I'll initialize the Union-Find structure for all users.
- I'll sort the logs by their timestamp.
- For each log entry, I'll merge the sets of the two users who became friends.
- I'll keep track of the number of connected components.
- Once there's only one connected component, I'll return the current timestamp.
- If I process all logs and still have more than one component, I'll return -1."
Candidate writes the code, explaining each step in detail, including the initialization, sorting, union operations, and the condition to check if all users are connected.
Interviewer: "Great. Now, let's analyze the time and space complexity of your solution."
Candidate: "The time complexity is O(n log n) due to the sorting step, where n is the number of logs. The Union-Find operations are almost constant time, so they don't significantly affect the complexity. The space complexity is O(u), where u is the number of unique users, to store the parent and rank arrays in the Union-Find structure."
Interviewer: "Good analysis. Now, let's move on to the final part of the interview. Do you have any questions for me about working at Google or anything else?"
Candidate: "Yes, I have a few questions. How do you find working at Google, and what do you enjoy most about your job here?"
Interviewer: "Working at Google is great. One of the things I enjoy the most is the flexibility and ownership we have over our projects. As a software engineer, I'm involved in both frontend and backend development, and I get to make significant decisions about the design and architecture of the applications. There's also a lot of variety in the projects we work on, which keeps things interesting."
Candidate: "What kind of projects do you typically work on, and what was your experience like during your internship?"
Interviewer: "My team works on internal applications that support various business functions, such as vendor and seller-facing applications. During my internship, I primarily worked on rebuilding a Java Spring application from scratch. It was a great learning experience, and I got to see firsthand how large-scale systems are designed and implemented."
Candidate: "Thank you for the insights. One last question, how would you describe the company culture at Google?"
Interviewer: "Google has a very dynamic and fast-paced culture. There's a strong emphasis on innovation and delivering results. The company values collaboration and teamwork, and there's a supportive environment for learning and growth. The leadership principles are a big part of our day-to-day work, guiding how we approach problems and interact with each other."
Candidate: "Thank you so much for answering my questions. I appreciate it."
Interviewer: "You're welcome. It was great speaking with you. You'll hear back from the recruiter within two to five business days regarding the next steps. Have a great day!"
Candidate: "Thank you. You too!"
Beyond Technical Skills: Key Competencies for Google Interviews
Besides technical questions, Google interviews also focus on several key soft skills and thought processes:
- Problem-Solving Ability: Interviewers want to see how you analyze and solve problems. Clearly break down the problem and solve it step-by-step.
- Code Quality: Code should be clean, readable, and well-commented. Interviewers will look at your coding style and structure.
- Communication Skills: Clearly articulate your thoughts and solutions during the interview. Good communication helps the interviewer understand your thinking and showcases your potential for teamwork.
- Flexibility in Thinking: Be prepared to adapt your approach during the interview, demonstrating flexibility and adaptability.
- Learning Ability: Interviewers may ask questions you're not familiar with; show your ability to quickly learn and apply new knowledge.
By focusing on these skills in addition to your technical prowess, you'll be well-prepared for your Google interview and other similar challenges. Good luck!
Through our powerful interview support, the candidate successfully navigated these interview questions. The analysis and discussion not only showcased the candidate's programming skills but also demonstrated their clear problem-solving approach and effective communication abilities. These insights are valuable not only for tackling Google 's interviews but also for enhancing our capability to solve real-world programming challenges. Good luck to everyone with your interviews!
经过我们的强力面试辅助,候选人通过这些面试题的解析和沟通,面试官不仅了解了候选人的编程能力,也看到了我在解决问题过程中清晰的思路和有效的沟通技巧。这些不仅有助于应对 Google 的面试,同时也能提升我们解决实际编程问题的能力。祝大家面试顺利!
如果你也需要我们的面试辅助服务,请立即联系我们。
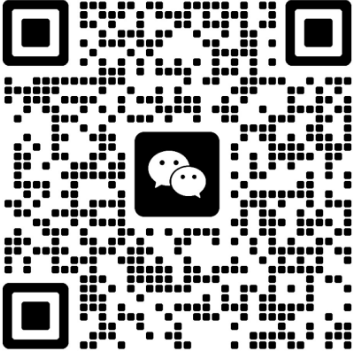