Background and Preparation
In preparation for Amazon's technical interview, I focused on problem-solving skills related to data structures and algorithms. I solved numerous coding problems on platforms like LeetCode, participated in mock interviews, and studied algorithmic patterns extensively.
为了帮助客户准备亚马逊的技术面试,我专注于提升与数据结构和算法相关的问题解决能力。我在LeetCode等平台上解决了大量的编程问题,参与了模拟面试,并深入研究了常见的算法模式。
Interview Setup
The virtual interview included multiple stages, each assessing different aspects of technical skills and problem-solving abilities. One stage involved a problem related to parsing and evaluating arithmetic expressions using Reverse Polish Notation (RPN).
面试是以虚拟形式进行的,包括多个阶段,每个阶段评估不同方面的技术技能和问题解决能力。其中一个阶段涉及解析和求解逆波兰表达式的问题。
Problem Description
The task was to design and implement a data structure to represent and evaluate complex arithmetic expressions given in Reverse Polish Notation. The problem required a thorough understanding of stack operations, operator precedence, and tree data structures.
Example Problem Statement
Given an arithmetic expression in RPN, convert it to an Arithmetic Expression Tree (AET) and evaluate it. Examples included:
给定一个逆波兰表达式,将其转换为算术表达式树(AET)并求解。示例如下:
a
should simply bea
a b +
should bea + b
a b + c *
should be(a + b) * c
a b + c d + *
should be(a + b) * (c + d)
a b + c d + * 5 *
should be((a + b) * (c + d)) * 5
Approach to Solution
Step 1: Parsing the Expression
The first step involved parsing the arithmetic expression to convert it into RPN. This required managing operator precedence using a stack. Here’s a concise breakdown of the approach:
- Initialize Structures: Create a stack for operators and a list for the output.
- Iterate Over Tokens: Loop through each token of the expression.
- Handle Operands: If the token is an operand (e.g., a number or variable), add it directly to the output.
- Handle Operators: If the token is an operator, pop operators from the stack to the output until an operator with lower precedence is found or the stack is empty, then push the current operator onto the stack.
- Handle Parentheses: Push opening parentheses onto the stack, and pop from the stack to the output until an opening parenthesis is encountered for closing parentheses.
- Flush the Stack: After processing all tokens, pop any remaining operators from the stack to the output.
第一步:解析表达式
第一步是解析算术表达式,将其转换为逆波兰表达式。这需要使用栈来管理运算符优先级。具体步骤如下:
- 初始化结构:创建一个栈用于运算符,一个列表用于输出。
- 遍历令牌:遍历表达式中的每个令牌。
- 处理操作数:如果令牌是操作数(如数字或变量),直接添加到输出中。
- 处理运算符:如果令牌是运算符,将栈中优先级不低于当前运算符的所有运算符弹出到输出中,然后将当前运算符压入栈中。
- 处理括号:将左括号压入栈中,遇到右括号时将栈中的运算符弹出到输出,直到遇到左括号。
- 清空栈:处理完所有令牌后,将栈中剩余的运算符弹出到输出中。
Step 2: Constructing the Expression Tree
The next step involved constructing an expression tree from the RPN expression:
- Initialize a Stack: Use a stack to build the tree.
- Iterate Over Tokens: Loop through each token in the RPN expression.
- Handle Operators: When an operator is encountered, pop two nodes from the stack, create a new tree node with the operator, and set the two popped nodes as its children. Push the new node back onto the stack.
- Handle Operands: When an operand is encountered, create a new tree node with the operand and push it onto the stack.
- Retrieve the Root: The remaining node on the stack after processing all tokens is the root of the expression tree.
第二步:构建表达式树
接下来是从逆波兰表达式构建表达式树:
- 初始化栈:使用栈来构建树。
- 遍历令牌:遍历逆波兰表达式中的每个令牌。
- 处理运算符:当遇到运算符时,从栈中弹出两个节点,创建一个新的树节点作为运算符,并将这两个节点设置为新节点的子节点。将新节点压入栈中。
- 处理操作数:当遇到操作数时,创建一个新的树节点作为操作数,并将其压入栈中。
- 获取根节点:处理完所有令牌后,栈中剩下的节点就是表达式树的根节点。
Evaluation
After constructing the tree, the final step was to evaluate it. This involved writing a function to traverse the tree and compute the result based on the operators and operands.
构建树后,最后一步是对其进行求值。这需要编写一个函数来遍历树,并根据运算符和操作数计算结果。
Conclusion
The problem was an excellent demonstration of my understanding of stack-based parsing and tree construction. It also highlighted the importance of thorough preparation and practice with complex data structures and algorithms. The interview experience was challenging yet rewarding, providing valuable insights into problem-solving under pressure.
这个问题是展示我对栈解析和树构建理解的一个很好的机会。它也强调了通过复杂数据结构和算法的全面准备和实践的重要性。这次面试经验既具有挑战性又令人满意,为在压力下解决问题提供了宝贵的见解。
Tips for Future Candidates
- Practice Core Data Structures: Ensure you are comfortable with stacks, queues, and trees.
- Understand Operator Precedence: This is crucial for parsing expressions correctly.
- Simulate the Interview Environment: Practice coding by hand or in a plain text editor to simulate the interview setting.
- Clarify Problem Requirements: Ask questions if any part of the problem statement is unclear.
练习核心数据结构:确保你对栈、队列和树的使用非常熟练。
理解运算符优先级:这是正确解析表达式的关键。
模拟面试环境:通过手写代码或在简单的文本编辑器中练习,模拟面试场景。
明确问题要求:如果问题描述有不清楚的地方,一定要提问。
Focusing on these areas can enhance problem-solving skills and performance in technical interviews.
经过我们的强力面试辅助,候选人通过这些面试题的解析和沟通,面试官不仅了解了候选人的编程能力,也看到了我在解决问题过程中清晰的思路和有效的沟通技巧。这些不仅有助于应对 Meta 的面试,同时也能提升我们解决实际编程问题的能力。祝大家面试顺利!
如果你也需要我们的面试辅助服务,请立即联系我们。
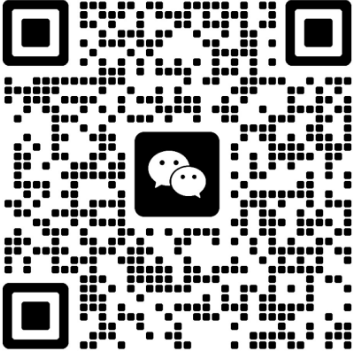