Welcome! In this guide, we will explore a real interview question from Apple. The challenge is to merge multiple 2D bounding boxes into a single bounding box that contains all the given boxes. This type of problem tests your understanding of geometry and your ability to manipulate data structures effectively. Let's dive in and break down the solution step-by-step.
Problem Statement
Given the following 2D Bounding Box struct, write a function to merge nnn bounding boxes together, and return a final bounding box with the result.
struct BBox
{
float x; // x position of the box center.
float y; // y position of the box center.
float w; // width of the box (x direction).
float h; // height of the box (y direction).
};
BBox merge(BBox *boxes, int boxCount)
{
// Your implementation here
}
Step-by-Step Guide to Implementing the Bounding Box Merge Function
Overview
The goal is to merge n
bounding boxes into a single bounding box that encapsulates all the given boxes. Each bounding box is represented by its center coordinates (x
, y
), width (w
), and height (h
). The result should be a bounding box that contains all the input bounding boxes.
Steps to Implement the Solution
- Handle Invalid Input:
- Check if the input array of bounding boxes (
boxes
) isnullptr
or if the number of bounding boxes (boxCount
) is less than or equal to zero. - If either condition is met, return an empty bounding box with all fields set to zero.
- Check if the input array of bounding boxes (
- Initialize Boundary Variables:
- Initialize variables to keep track of the minimum and maximum x and y coordinates (
minX
,maxX
,minY
,maxY
). - Use the first bounding box in the array to initialize these variables.
- Initialize variables to keep track of the minimum and maximum x and y coordinates (
- Update Boundary Variables:
- Loop through the remaining bounding boxes.
- For each bounding box, calculate its boundary coordinates:
currMinX = x - w / 2
currMaxX = x + w / 2
currMinY = y - h / 2
currMaxY = y + h / 2
- Update the boundary variables (
minX
,maxX
,minY
,maxY
) based on the current box’s boundaries.
- Calculate Merged Bounding Box:
- Compute the center of the merged bounding box using the average of the minimum and maximum boundary coordinates:
mergedBox.x = (minX + maxX) / 2
mergedBox.y = (minY + maxY) / 2
- Compute the width and height of the merged bounding box as the differences between the maximum and minimum boundary coordinates:
mergedBox.w = maxX - minX
mergedBox.h = maxY - minY
- Compute the center of the merged bounding box using the average of the minimum and maximum boundary coordinates:
- Return the Result:
- Create a
BBox
object with the calculated center, width, and height. - Return this
BBox
object as the result.
- Create a
Detailed Explanation
- Handle Invalid Input:
- It is crucial to validate the input to ensure that the function does not proceed with invalid data, which could cause undefined behavior or crashes.
- Initialize Boundary Variables:
- Using the first bounding box helps set a reference point for the initial boundaries. This is important as it sets a baseline for comparisons when updating boundaries with other boxes.
- Update Boundary Variables:
- For each bounding box, calculate its boundary by considering its width and height around its center.
- Update the minimum and maximum x and y coordinates by comparing with the current box’s boundaries. This ensures that you get the smallest and largest possible coordinates that encompass all boxes.
- Calculate Merged Bounding Box:
- The center of the merged bounding box is the midpoint between the smallest and largest x and y coordinates.
- The width and height are the total distances covered by the bounding boxes in the x and y directions, respectively.
- Return the Result:
- Constructing the
BBox
object with the computed center, width, and height encapsulates all input bounding boxes.
- Constructing the
By following these steps, you can effectively merge multiple bounding boxes into a single bounding box that contains all the original boxes. This approach ensures that the merged box is the smallest possible box that can contain all given boxes, maintaining the simplicity and efficiency of the algorithm.
Conclusion
Apple's interview questions cover a diverse array of topics, from basic geometric calculations and data structure manipulations to more complex algorithmic challenges. These examples showcase the breadth and variety of problems you may face during the interview process. Preparing for such questions will help you build the problem-solving skills and technical knowledge necessary to excel.
In conclusion, Apple's interview process includes a wide range of technical and problem-solving questions designed to comprehensively assess a candidate's abilities. From geometric calculations and data structure manipulations to complex algorithmic challenges, these questions demand a thorough understanding of fundamental programming concepts and the ability to apply them effectively.
If you need assistance preparing for your Apple interview, we offer interview coaching and support services. Our team is available to provide real-time support during your online interviews. Contact us to purchase our services and boost your interview readiness.
苹果的面试题涵盖了广泛的主题,从基本的几何计算和数据结构操作到更复杂的算法挑战。这些例子展示了您在面试过程中可能遇到的问题的广度和多样性。准备这些类型的问题将帮助您培养解决问题的技能和必要的技术知识,从而取得成功。
总之,苹果的面试过程包含各种技术和解决问题的问题,旨在全面评估候选人的能力。从几何计算和数据结构操作到复杂的算法挑战,这些问题需要您对基本编程概念有深入的理解,并能够有效地应用它们。
如果您需要帮助准备apple面试,我们提供面试辅导和面试辅助服务。我们的团队可以在您的线上面试过程中提供实时支持。请联系我们购买服务,提升您的面试准备水平。
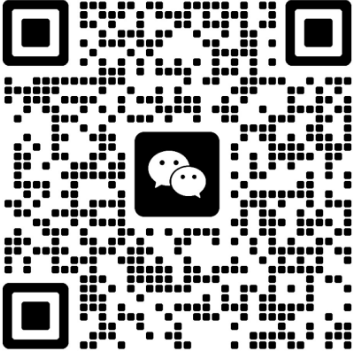