During my recent technical interview at TikTok, I was presented with two intriguing coding problems. Here is the original text of the problems and my approach to solving them.
Problem 1:
Given a string s, return true if it is a palindrome, or false otherwise. Input: s = "aba" Output: true
This problem is relatively simple, essentially checking whether a string reads the same backward as forward. The definition of a palindrome is just that, identical in sequence in both directions.
Approach 1: Using the REVERSE Method:
The simplest method is to reverse the string and compare if the reversed string is the same as the original string.
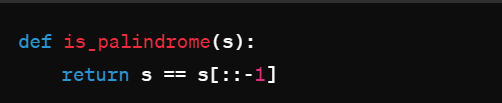
Time Complexity: The time complexity of this method is O(n), where n is the length of the string. This is because reversing the string itself requires traversing the entire string.
Space Complexity: The space complexity of this method is also O(n) since you need additional space to store the reversed string.
Approach 2: Using the Two-Pointer Method:
The two-pointer method involves starting from the beginning and end of the string simultaneously, moving towards the center, and comparing the characters at each step.
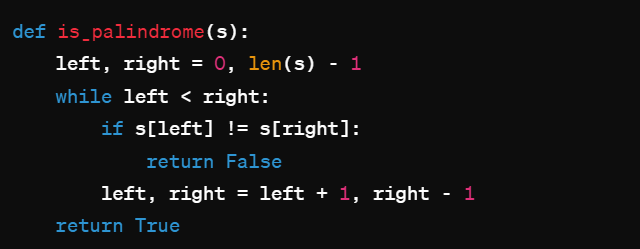
Time Complexity: The time complexity of this method is also O(n) since you may need to traverse half of the entire string.
Space Complexity: The space complexity of this method is O(1) because it only requires a constant level of additional space, that is, two pointers.
Comparison:
Performance: Although both methods have a time complexity of O(n), the two-pointer method can terminate early in the best case (when the string is not a palindrome from the start), while the reverse method must traverse the entire string.
Space Efficiency: The two-pointer method is clearly more space-efficient as it does not require creating a copy of the string. In practice, if the string is very large, avoiding a copy can save a significant amount of memory.
Readability: The reverse method code is more concise and straightforward, which might be a better choice for scenarios that do not pursue extreme performance and space optimization, as it is easier to understand and maintain.
Problem 2:
You are given a string s. You can convert it to a palindrome by adding characters in front of it. Return the shortest palindrome you can find by performing this transformation. Input: s = "aacecaaa" Output: "aaacecaaa"
This problem is more complex than the first. It not only requires us to check if a string is a palindrome but also to be able to create a palindrome by adding characters.
Solving this Problem
The method is to use string matching techniques. My approach was to compare the string s with its reverse s', finding the longest prefix and suffix that match at the beginning of s and the end of s'. Then, add the unmatched part of s' to the front of s to form the shortest palindrome.
The specific steps are as follows:
- Create the reverse of string s, denoted as s'.
- Start from the first character of s and compare it with the last character of s', moving character by character forward (backward for s') until the longest matching prefix is found.
- Add the unmatched part of s' to the front of s.
While writing the code, I used Python, as it's very convenient and powerful for string handling. I also had to consider edge cases, such as an empty string or a string that is already a palindrome.
Interview Process:
During the interview, I first explained my thought process, which the interviewer approved of. Then, I wrote down the pseudocode on the whiteboard, discussed potential edge cases, and explained how my code would handle these cases.
For the first problem, my code quickly passed all the test cases. However, for the second problem, I initially overlooked a special case where if the input string was already a palindrome, it should return the original string. After the interviewer pointed this out, I quickly adjusted my code, and it passed all tests.
The interviewer was satisfied with my coding style and problem-solving ability and engaged in some technical depth discussions with me, such as how to optimize my algorithm and potential performance issues when running on large datasets.
Overall, this interview was a very positive experience. It tested not only my coding skills but also my problem-solving techniques and communication abilities. Contact me for more interview experiences; we offer interview assistance, coaching, and other services.